Injectable decorator not recognized when used with custom decorator
See original GitHub issue🐞 bug report
Affected Package
The issue is caused by package @angular/compiler
Is this a regression?
Yes, since everything worked fine in previous versions (Angular 8, Angular 9)
Description
In my projects I use decorators and now for some reason the error is constantly shown, what does this mean and how to get around it? Is this a bug in Angular? The decorator is ordinary and made according to the TypeScript guide
import { Component, VERSION, Injectable } from '@angular/core';
// simple example
export function Decorator(): any {
return function <T extends any>(parent: any) {
return class extends parent {
constructor(...args: any[]) {
super(...args);
}
};
};
}
@Decorator()
@Injectable()
class MyService {
public world() {
return 'world';
}
}
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ],
providers: [MyService]
})
export class AppComponent {
name = 'Angular ' + VERSION.major;
constructor(private my: MyService){
console.log('hello', my.world())
}
}
🔬 Minimal Reproduction
https://stackblitz.com/edit/token-invalid?file=src/app/app.component.ts
🔥 Exception or Error
DEPRECATED: DI is instantiating a token "" that inherits its @Injectable decorator but does not provide one itself.
This will become an error in a future version of Angular. Please add @Injectable() to the "" class.
🌍 Your Environment
Angular Version:
Angular 10.1
Issue Analytics
- State:
- Created 3 years ago
- Reactions:6
- Comments:17 (7 by maintainers)
Top Results From Across the Web
Why does HTTP service require @Injectable decorator and a ...
Generally speaking, an injector reports an error when trying to instantiate a class that is not marked as @Injectable() .
Read more >Migration for missing @Injectable() decorators and incomplete ...
This schematic adds an @Injectable() decorator to classes which are provided in the application but are not decorated. The schematic updates providers which ......
Read more >Angular & Dependency Injection: tricks with Decorators
The SkipSelf Decorator doesn't look for the dependency in the current injector and starts from the parent injector (and then continues to go ......
Read more >How To Use Decorators in TypeScript - DigitalOcean
The decoratorA function will be called with details about how you used the decorator in your code. For example, if you applied the...
Read more >Decorators do not work as you might expect
While working on a library called ngx-template-streams, which in a nutshell allows you to work with e... Tagged with typescript, webdev, ...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
For anybody still interested, this works:
Tested on Angular 12.
As mentioned here: https://stackoverflow.com/a/53622493/4663231
Proxy
andReflect.construct
can be used:and the output is: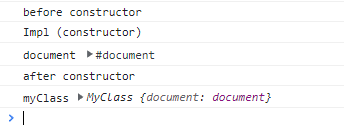
as you can see:
DI works without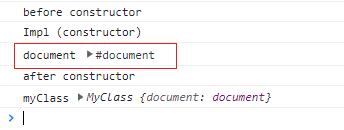
@Injectable
inside custom decoratorreturned class is the original one (not wrapped)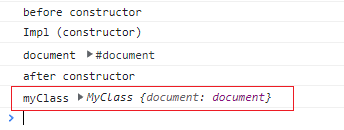