Unable to use create database via API: `The CSRF token is missing.`
See original GitHub issueExpected results
POST /api/v1/database
endpoint should work because it’s in the documentation.
Actual results
When I use the Swagger client embedded into the application (/swagger/v1
), the API throws the following exception:
The CSRF token is missing.
Here is a snippet that reproduces the same issue as well:
token_request = requests.get("{}/api/v1/security/csrf_token".format(self.superset_url),
headers={'Authorization': 'Bearer {}'.format(self.current_access_token)})
if token_request.status_code != 200:
raise Exception("Unable to get CSRF token: {}".format(token_request.text))
csrf_token = token_request.json().get('result')
r = requests.post("{}/api/v1/database".format(self.superset_url),
json={
"allow_csv_upload": False,
"allow_ctas": True,
"allow_cvas": False,
"allow_dml": False,
"allow_multi_schema_metadata_fetch": True,
"allow_run_async": True,
"database_name": database_name,
"expose_in_sqllab": True,
"sqlalchemy_uri": "presto://.."
},
headers={'Authorization': 'Bearer {}'.format(self.current_access_token), "X-CSRFToken": csrf_token})
Screenshots
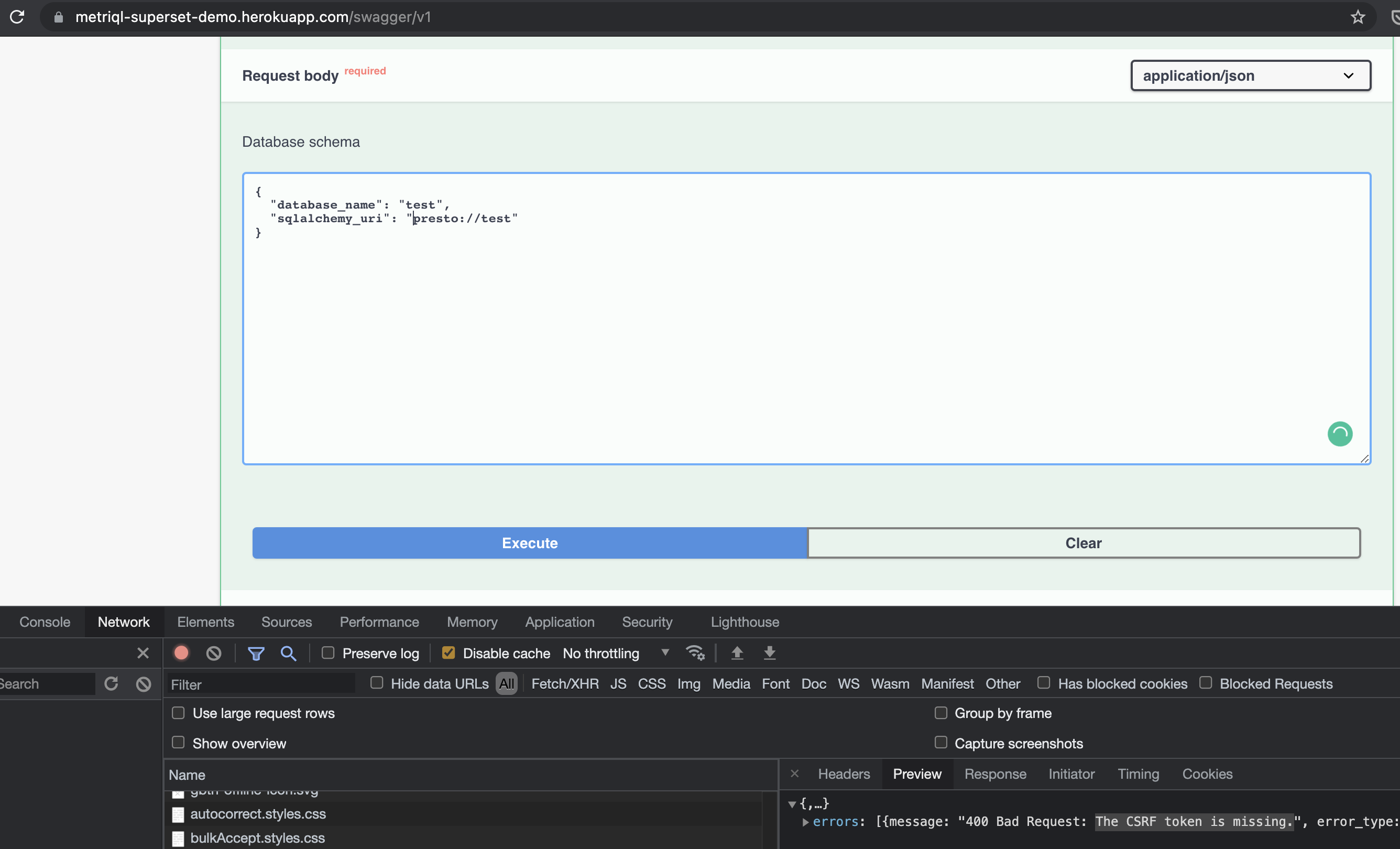
Environment:
superset version: superset version 1.2.0
python version: python --version: python-3.7.10
node.js version: node -v: not relevant
Checklist
Make sure to follow these steps before submitting your issue - thank you!
- I have checked the superset logs for python stacktraces and included it here as text if there are any.
- I have reproduced the issue with at least the latest released version of superset.
- I have checked the issue tracker for the same issue and I haven’t found one similar.
Issue Analytics
- State:
- Created 2 years ago
- Reactions:1
- Comments:11
Top Results From Across the Web
[GitHub] [superset] buremba opened a new issue #16398
[GitHub] [superset] buremba opened a new issue #16398: Unable to use create database via API: `The CSRF token is missing.`.
Read more >"detail": "CSRF Failed: CCSRF token missing." when sending ...
i am getting csrf token in my response from the views in the form of cookie but the problem is that i am...
Read more >Missing CSRF Token Fix | Part 3.5 - YouTube
Fixing missing CSRF token when submitting post data while using "fetch".I have seen multiple students have this issue in video #4 of my ......
Read more >Issues with CSRF token and how to solve them - SAP Blogs
There are several blog posts in SCN using this library. You should fetch CSRF token before every modify operation, if you want to...
Read more >OAuth 2.0 identity provider API - GitLab Docs
For example, the X-Requested-With header can't be used for preflight requests. ... These users can access the API using personal access tokens instead....
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
@youngsol Here is how it works in our case: https://github.com/metriql/metriql-superset/blob/main/metriql2superset/superset.py#L21
In case this helps anyone else, the problem with the above code is that the CSRF token must be obtained in the same session as where it is used, because there is a session cookie added by the call that creates the CSRF token. This became evident after reading: https://github.com/wtforms/flask-wtf/blob/4b90067908f0ab42bf25f4bc580a4367e09e27dd/src/flask_wtf/csrf.py#L66 However, after fixing that, I got the new error:
400 Bad Request: The referrer header is missing.
The fix for this second error must take into account the fact that Referrer header should be misspelled as Referer: https://flask.palletsprojects.com/en/2.1.x/api/#flask.Request.referrerIn the end this code worked fine: