How to declare fragment in functional component in Typescript?
See original GitHub issueI can easily declare a fragment in a class component by adding a static method.
export default class PokemonCard extends React.Component {
static fragments = {
pokemon: gql`
fragment PokemonCardPokemon on Pokemon {
url
name
}
`
}
// ...
}
What is the best way to do the same in functional component in Typescript and pass the type checking?
PokemonCard.fragments = {
pokemon: gql`
fragment PokemonCardPokemon on Pokemon {
url
name
}
`,
};
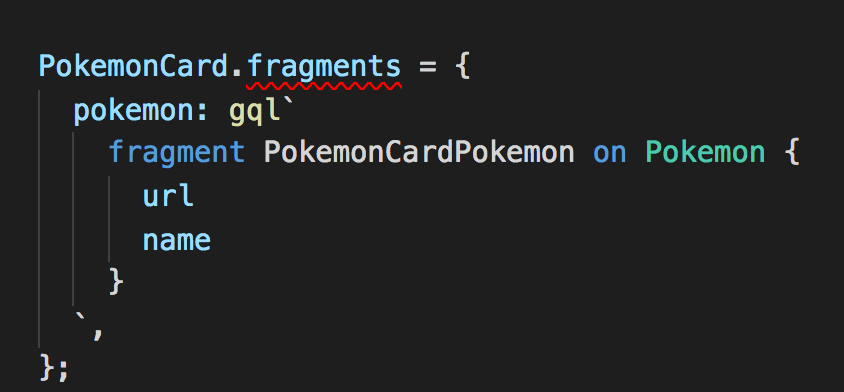
// error: ‘fragments’ does not exist on type ‘typeof PokemonCard’
Issue Analytics
- State:
- Created 6 years ago
- Reactions:6
- Comments:7 (1 by maintainers)
Top Results From Across the Web
react 16 fragment functional component and typescript TS2605
When I write a functional component that returns a fragment: function MyComponent() { return [<div>1</div>, <div>2</div>]; }. Typescript ...
Read more >JSX Fragment Syntax in TypeScript - Marius Schulz
The compiler replaces the short fragment syntax by a call to the React.createElement() method and passes it React.Fragment as the first argument ...
Read more >Fragments - React
Fragments let you group a list of children without adding extra nodes to the DOM. There is also a new short syntax for...
Read more >React with TypeScript: Components as Function Declarations ...
When using React with Typescript, let's look at using components as function declarations vs. function expressions.
Read more >Documentation - JSX - TypeScript
If the process succeeds, then TS finishes resolving the expression to its declaration. If the value fails to resolve as a Function Component, ......
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Yeah, we ended up doing pretty much what @danielrasmuson described, except we colocate the fragment with the component, and use default and non-default exports to use this pattern:
My understanding (feedback welcome) is that doing
ReactComponent.fragments
is just a convention.I’m using typescript and I’ve decided to opt out of using fragments this way for exactly the reason you detailed above.
Instead I have a file devoted to a fragment…
file:
queries/pokemon-card.fragment.tsx
Then in your query…
Lastly pro tip! Include id in your fragment query and anytime that fragment is fetched it will reload all components that use that fragment data.
I.E. if you have some mutation
And as long as your mutation returns
PokemonCardPokemon
it will reload all components using that information.