useQuery not returning data when mock doesn't match query shape
See original GitHub issueIntended outcome:
Test with MockedProvider receiving mocked data successfully.
related code (minimal repro):
import React from 'react'
import { render } from '@testing-library/react'
import '@testing-library/jest-dom/extend-expect'
import { useQuery, gql } from '@apollo/client'
import { MockedProvider, MockedResponse } from '@apollo/client/testing'
const MY_QUERY = gql`
query {
__schema {
types {
name
kind
description
}
}
}
`
function MyComponent() {
const introspectionResult = useQuery(MY_QUERY)
if (introspectionResult.loading) return <div data-testid='my-loader'>loading...</div>
console.log(introspectionResult)
if (!introspectionResult.data) throw new Error('should not throw!')
return <div data-testid='my-div'>doesnt matter what to render</div>
}
test('some test', async () => {
const mockedResponses: MockedResponse[] = [
{
request: { query: MY_QUERY },
result: {
data: {
__schema: {
types: [
{ name: 'bla', kind: 'yada' },
{ name: 'blabla', kind: 'yadayada' },
],
},
},
},
},
]
const { findByTestId } = render(
<MockedProvider mocks={mockedResponses}>
<MyComponent />
</MockedProvider>,
)
const myComponent = await findByTestId('my-div')
expect(myComponent).toBeInTheDocument()
})
expected output:
test passes with useQuery response.data filled with the mock data, like this:
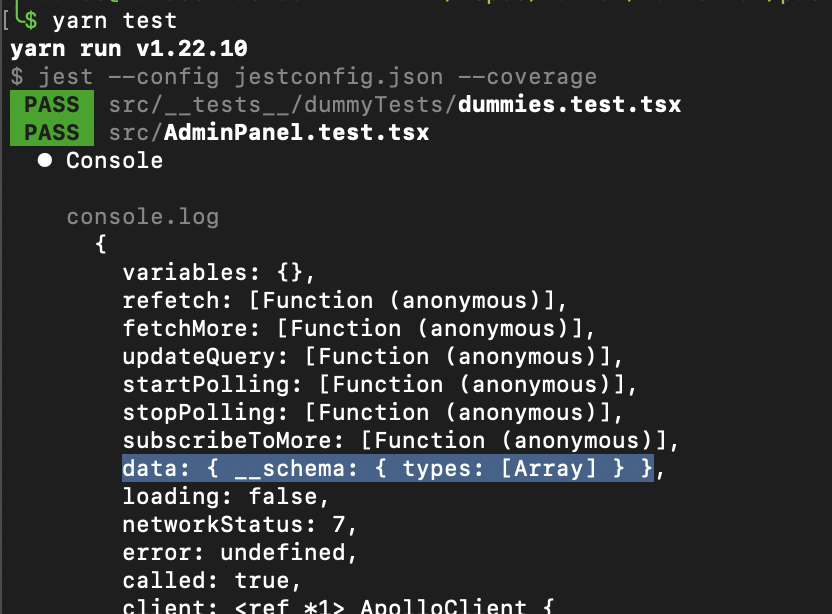
Actual outcome:
test fails with data: undefined
even after loading finishes and there are no errors reported by the useQuery hook.
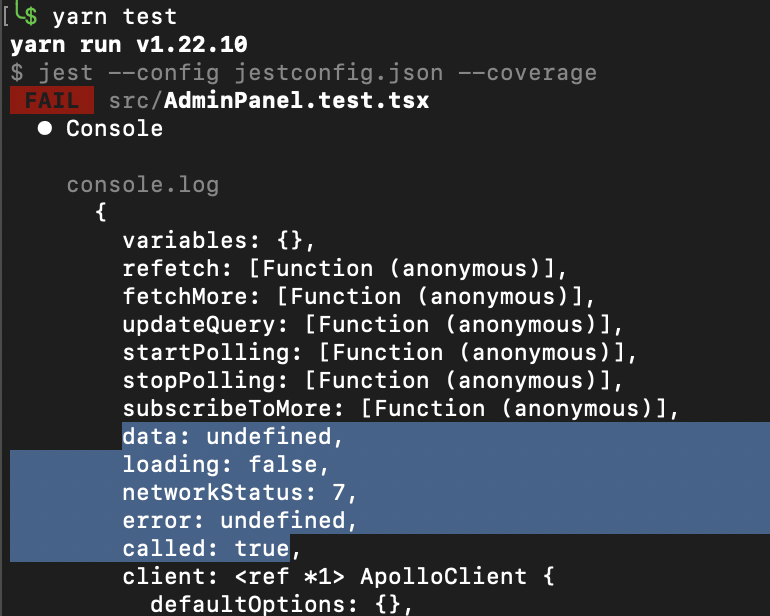
If you add description: whatever
to each of the mocks (schema.types[n].description), the mock works fine.
My guess is that the the lack of a field asked for in the query, apparently causes some silent error, I would expect the useQuery
function to return the mocked data, even without a description
field.
How to reproduce the issue:
codesandbox to reproduce: https://codesandbox.io/s/sweet-bardeen-tjn9i?file=/src/MyComponent.mocks.js
steps:
- go to tests section, tests should pass.
- comment-out the “description” fields at
MyComponent.mocks.js
(line 12 and 17). - run tests again, tests will fail.
Is this a bug or an expected behaviour I am unaware of?
if so, what do you recommend to mitigate this issue when testing? I would like to just mock the required sections of the query response for my particular test, for legibility and conciseness.
Versions
Issue Analytics
- State:
- Created 2 years ago
- Reactions:2
- Comments:6
Actually i think this is the exact same bug as #8063. If a mantainer can confirm, I think it’s safe to close this duplicate issue.
Also #8063 is already marked as “fixed in pre-release”, so I guess is just a matter of time now
I have similar issue, check out my previous post: https://github.com/apollographql/apollo-client/issues/7816
How I worked it around is I use
useApolloClient()
instead ofuseQueryI()
Here is a brief example:I think there is something wrong with
useQuery
hook