Changing the Configuration at Runtime
See original GitHub issueI would like to change the configuration at runtime:
const [someConvention, setSomeConvention] = useState();
const configuration = getConfiguration(someConvention);
return <AuthenticationProvider
configuration={configuration}
{...restOfProps}
>
{children}
</AuthenticationProvider>
But because of how authenticationServiceInternal
in authenticationService.ts
is made with that if
at the beginning, new configurations will never be set.
export const authenticationServiceInternal = (
WebStorageStateStoreInt: typeof WebStorageStateStore
) => (configuration: UserManagerSettings, UserStore?: UserStoreType) => {
if (userManager) {
return userManager;
}
[...]
Can you please instruct me on what is the proper way to change the configuration at runtime without having to access setUserManager
and set it to null
. The code doesn’t seem to want that export being used by client developers … I think. And it feels like a dirty workaround.
Maybe include a comparison in that if
to see if the config is changed?
Issue Analytics
- State:
- Created 3 years ago
- Reactions:2
- Comments:36 (16 by maintainers)
Top Results From Across the Web
How do I modify config file at runtime - Stack Overflow
I need to change the config file myApp.exe.config at runtime. How to make sure that the program would be started to work with...
Read more >How to change configuration at runtime ? · Issue #923 - GitHub
Yes, switching configs at runtime is not possible. Not without a reload.
Read more >Modifying Configuration Settings at Runtime - CodeProject
This article will demonstrate how to add, delete, and update key value pairs in an App.config file.
Read more >Modifying .NET Configuration Files at Runtime - C# Corner
Now set the value to be changed in the AdminConsole application and click on the "Modify" button. Changing-the-configuration-element.gif. Figure ...
Read more >Runtime Configuration - ActiveMQ
From version 5.9.0 a new broker plugin will allow selective changes to a broker xml configuration to take effect without broker restart.
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Hello, I have a hard time sharing code in a sand box because there are 3 projects that need to go up (React + Apollo frontend, Nodejs GQL backend and the Identity server dotnet core).
So here is an attempt at putting here the important part. If it’s not enough I will try to make a sand box because we don’t need to involve GQL right now. I can show you that the token in
oidcDatabase
is not the same as the token in the session storage.Here we have the configuration function. The important part is the
acr_values
that value dictates a property inside the access token. Based on theacr_values
the Identity server setstid
(tenant id) inside the tokenHere is my authentication wrapper. I create a state that is connected to the session storage. From here we expose a method available for the whole project that can change the
tenant
value. When this value is change, we get a newacr_values
in our configuration that will dictate the value of thetid
in our token.And now to show the difference on screen
And now for some step by step pictures. When I first login everything is good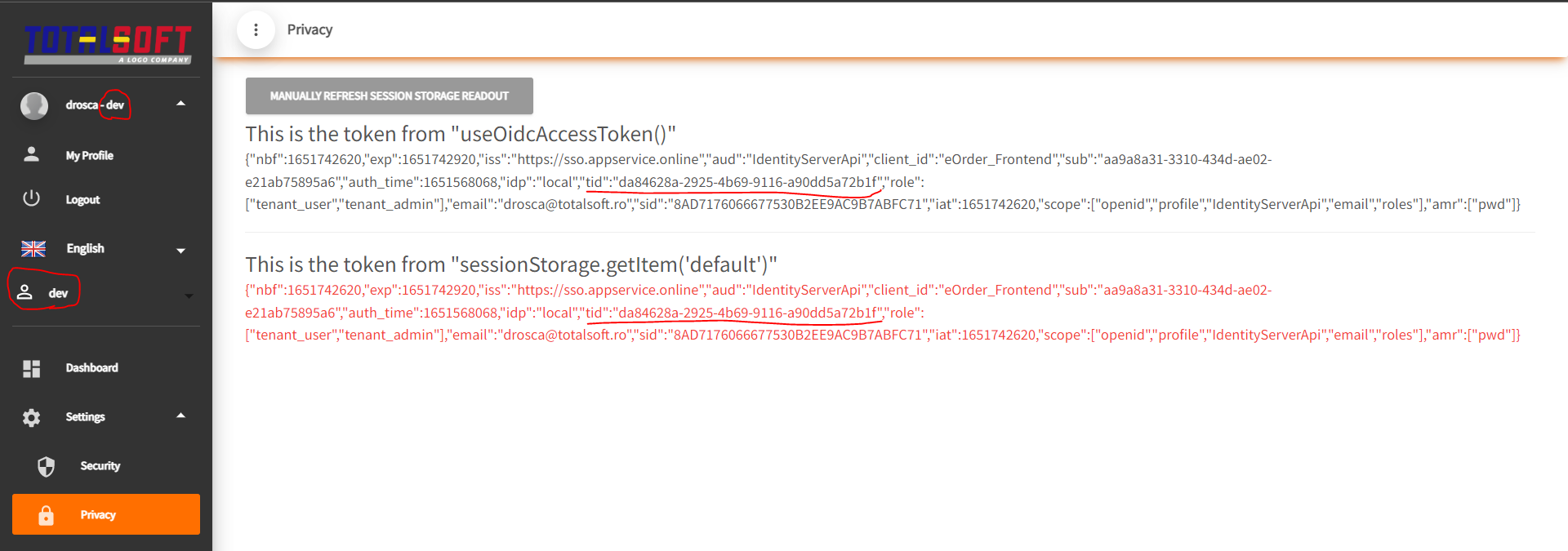
Now lets change the tenant and reread the session storage token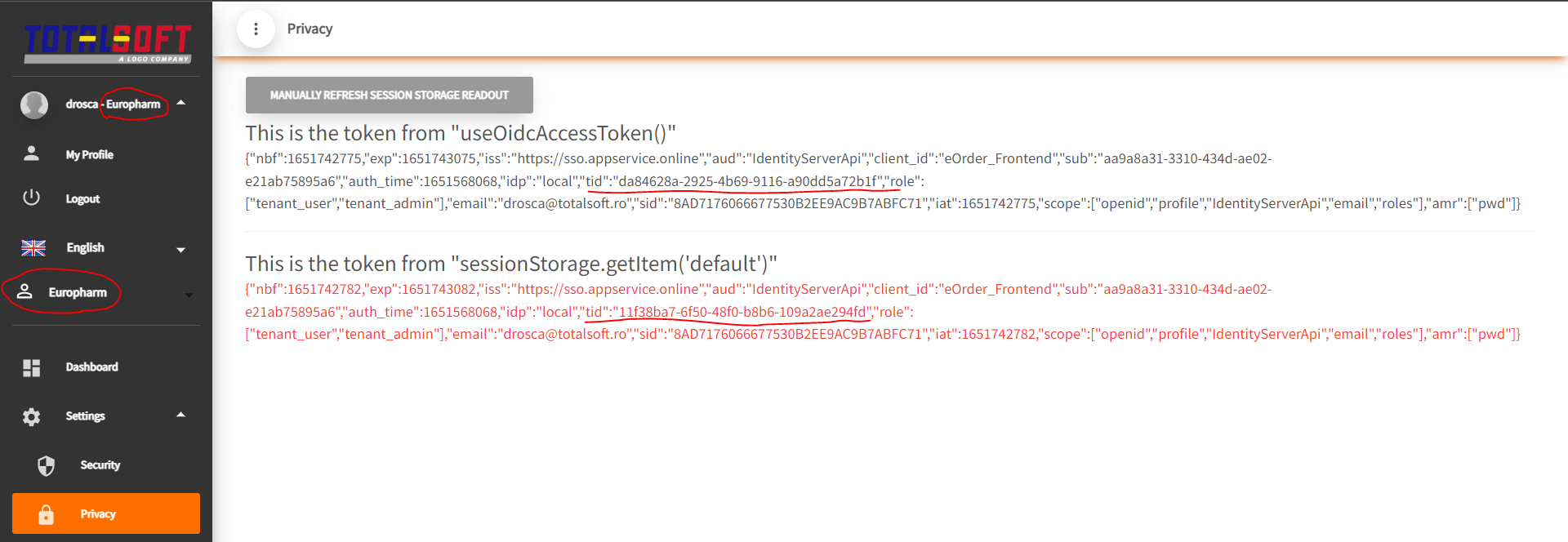
The labels on the left are the names from the session storage that dictates the
acr_values
they are not taken from the token.Hope this helps and if it doesn’t I will try to see if I can make a sand box for you to test.
Thank you for helping me and have a great day!
Yes, i would like to work on it. But i am lacking of time. Thank you for the message