Suggestion to improve code and performance
See original GitHub issueWhy does the BindingFactoryHelpers class uses reflection to build the CustomTriggerArgumentBinding? There are no advantages in this approach, only disadvantages:
- It makes the code very difficult to understand.
- Worsens performance.
Solution option
Reflection is not needed to build the SimpleTriggerArgumentBinding
, it is enough to take the following steps:
- To get the FuncAsyncConverter you need to use the
FuncAsyncConverter GetConverter<TAttribute>(Type typeSource, Type typeDest)
method in the IConverterManager instance. - Create
CustomTriggerArgumentBinding<TMessage, TTriggerValue>
class in which “UserType” can be passed through the constructor.
Code examples
The GetDirectTriggerBindingWorker
method in the BindingFactoryHelpers class should be rewritten as follows.
private static SimpleTriggerArgumentBinding<TMessage, TTriggerValue>
GetDirectTriggerBindingWorker<TMessage, TTriggerValue>(
ITriggerBindingStrategy<TMessage, TTriggerValue> bindingStrategy,
IConverterManager converterManager,
Type userType)
{
var directConvert = converterManager.GetConverter<Attribute>(typeof(TMessage), userType);
if (directConvert != null)
{
var argumentBinding = new CustomTriggerArgumentBinding<TMessage, TTriggerValue>(
bindingStrategy, converterManager, directConvert, userType);
return argumentBinding;
}
return null;
}
The GetDirectTriggerBinding
method in the BindingFactoryHelpers class should be rewritten as follows.
private static SimpleTriggerArgumentBinding<TMessage, TTriggerValue>
GetDirectTriggerBinding<TMessage, TTriggerValue>(
Type exactType,
ITriggerBindingStrategy<TMessage, TTriggerValue> bindingStrategy,
IConverterManager converterManager)
{
return GetDirectTriggerBindingWorker(bindingStrategy, converterManager, exactType);
}
An example of the implementation of the class CustomTriggerArgumentBinding<TMessage, TTriggerValue>
.
internal class CustomTriggerArgumentBinding<TMessage, TTriggerValue> :
SimpleTriggerArgumentBinding<TMessage, TTriggerValue>
{
private IBindingDataProvider _bindingDataProvider;
private readonly FuncAsyncConverter _converter;
private readonly Type _userType;
public CustomTriggerArgumentBinding(
ITriggerBindingStrategy<TMessage, TTriggerValue> bindingStrategy,
IConverterManager converterManager,
FuncAsyncConverter converter,
Type userType) : base(bindingStrategy, converterManager)
{
if (converter == null)
{
throw new ArgumentNullException(nameof(converter));
}
this._converter = converter;
_userType = userType;
this.ElementType = _userType;
_bindingDataProvider = BindingDataProvider.FromType(ElementType);
AddToBindingContract(_bindingDataProvider);
}
internal override async Task<object> ConvertAsync(
TMessage value,
Dictionary<string, object> bindingData,
ValueBindingContext context)
{
var obj = await _converter(value, null, context);
AddToBindingData(_bindingDataProvider, bindingData, obj);
return obj;
}
}
Result
The above code examples allow you to get the same behavior, but with more readable code and without sacrificing performance.
Issue Analytics
- State:
- Created 2 years ago
- Reactions:6
- Comments:5 (2 by maintainers)
Top Results From Across the Web
7 Steps to Improve Code Quality
Improving code quality is a crucial aspect of software development. Whether a beginner or an experienced developer, always look for ways to ...
Read more >6 Keys to Improving Your Programming Performance
6 Keys to Improving Your Programming Performance · 1. Design for Success · 2. Tools for Efficiency · 3. Collaborate to Reach Your...
Read more >6 Ways to improve coding speed and optimize performance
1.Typing Speed ... Typing speed isn't the single biggest factor for increasing your coding speed, but it plays an important role in your...
Read more >Five Ways to Improve Your Code Quality
Five Ways to Improve Your Code Quality ; Improve Readability: How will others understand? Tip 1: Create and enforce a consistent coding style....
Read more >10 ways to improve your code after it's 'finished'
10 ways to improve your code after it's 'finished' · Lint · Profile · Remove debugging tools · Analyze with AI · Curate...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Hi @v-anvari , I raised a new pull request for this case. The solution was successfully built, but I can’t figure out what the problem is with vstest.console. Looks like it’s a pipeline setup problem. Let me know if the problem is with my pull request.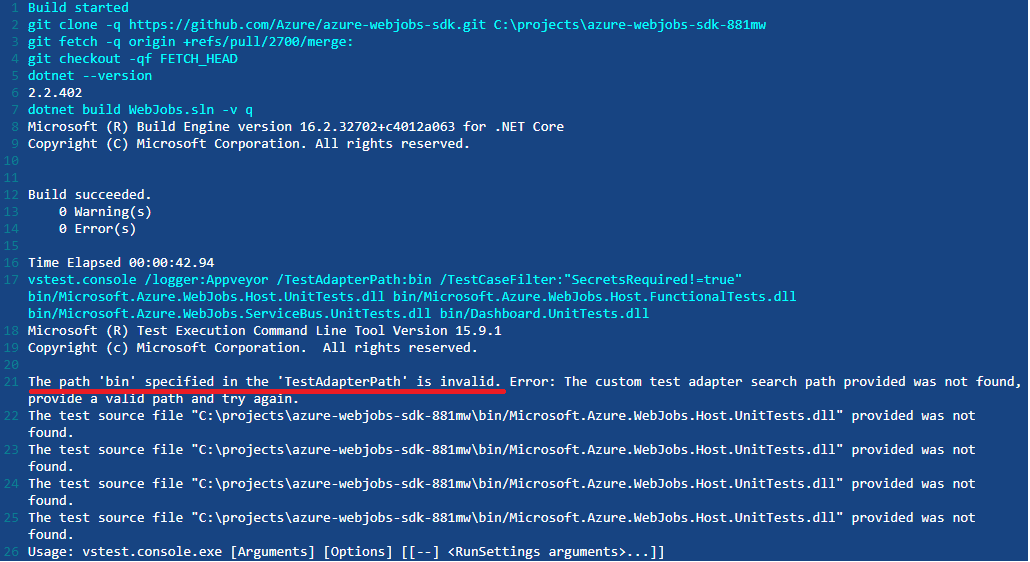
Hi @Shazhko-Artem , I am following up with the team and the PR will be assigned to anyone from the team for further look up.