NaN training error when importing training data from JSON
See original GitHub issueWhat is wrong?
When loading training data from a local JSON file, stringify and parsing, running the network results in a training error of: NaN
.
Where does it happen?
I’m running Brain JS on my Macbook with an i7 processor within a Node JS script, executed locally through my terminal
How do we replicate the issue?
My code is as follows:
dataset-dedupe-cleansed.json
[
{
"input":"[0.97,0.94,0.56,0.94]",
"output":"[1]"
},
{
"input":"[0.24,0.54,0.35,0.74]",
"output":"[1]"
}
]
app.js
// test users
const testUsers = [
{ name1: 100, name2: 5, name3: 1000, name4: 200 },
{ name1: 1200, name2: 12, name3: 2000, name4: 900 }
]
// get a random user
const user = testUsers[Math.floor(Math.random() * testUsers.length)];
// normalize based on min/max
function normalize (val, max, min) {
return (val - min) / (max - min);
}
// round number
function round (val, precision) {
var multiplier = Math.pow(10, precision || 0)
return Math.round(val * multiplier) / multiplier
}
// specify training options
const trainingOptions = {
log: true,
iterations: 20000,
errorThresh: 0.005,
learningRate: 0.3,
timeout: Infinity
}
// get JSON training data (format is array of objects)
const trainingDataSet = JSON.parse(fs.readFileSync('./training/dataset-dedupe-cleansed.json', 'utf8'))
// init network
const network = new brain.NeuralNetwork({hiddenLayers: [4, 1]})
// format training data
const trainingData = JSON.parse(JSON.stringify(trainingDataSet))
// train the network
network.train(trainingDataSet, trainingOptions)
// run the network
var checkRisk = network.run([
round(normalize(user.name1, 100, 5000), 2),
round(normalize(user.name2, 1, 36), 2),
round(normalize(user.name3, 400, 2000), 2),
round(normalize(user.name4, 0, 4800), 2)
]);
// Result
console.log(checkRisk)
I do have a Code Pen that I’ve set up for this, for tinkering: https://codepen.io/sts-ryan-holton/pen/bGdNOGr
Note: the training data is stripped right back, but in my local environment I have a few hundred objects in my array. I’ve got the functions normalize
and round
to “normalize the network” as well 👍
How important is this (1-5)?
5
Expected behavior (i.e. solution)
I should get a correct training error returned by Brain JS when log
is set to true
Other Comments
When logging checkRisk
, I get some random response back? I’m trying to get my training data to essentially be the following from the JSON file:
const trainingData = {
{ input: [0.97,0.94,0.56,0.94], output: [1] },
{ input: [0.24,0.54,0.35,0.74], output: [1] }
}
I should get a Float32
returned
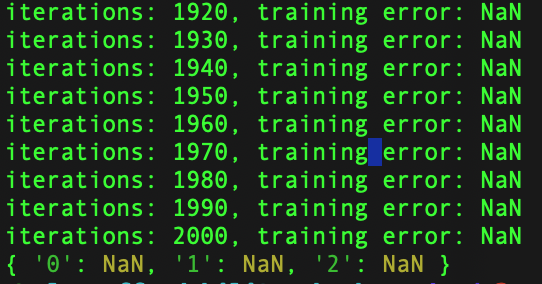
Issue Analytics
- State:
- Created 4 years ago
- Comments:8 (4 by maintainers)
Data is defined to only overcome the issue in the online pen. Main thing is the part of code which follows after that. i.e. You can load JSON file and train network as required.
You can load data rom external json file, its just that your data does not seem to be valid.
Here is working demo of above data: https://stackblitz.com/edit/js-cpsecv?file=index.js