Request for help bundling chakra
See original GitHub issueI’ve built some components that wrap chakra components. I’m trying to strip them out into their own library that I can include in other projects. I’m receiving an error on loading my apps that I include this library into when I try to use toasts.
I’ve tried several different packages to build it, including rollup, microbundle, and tsdx. The error message I am getting is “Invalid hook call”.
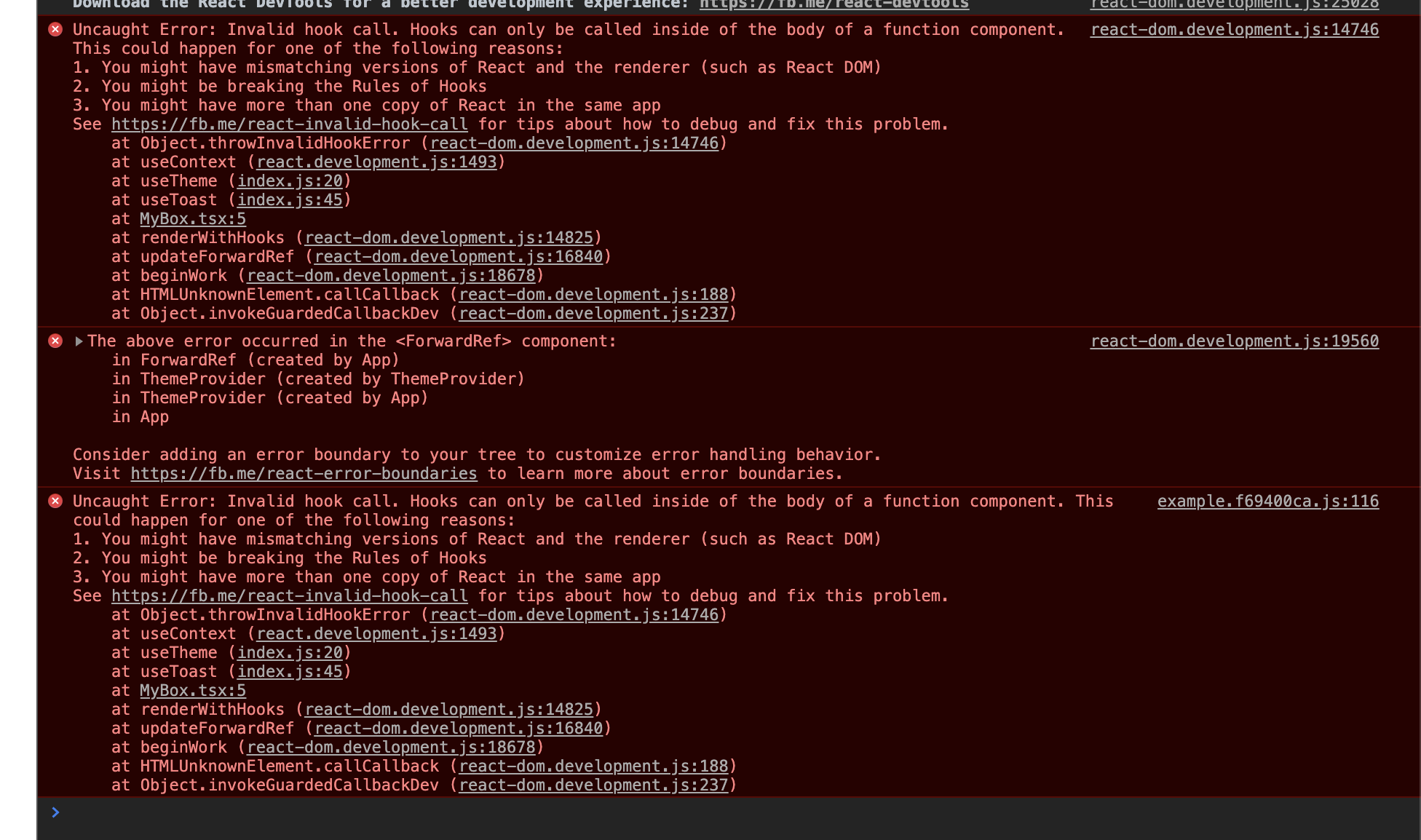
I was wondering if anyone has successfully accomplished something similar and would be generous to write something up on how it was done.
I’ve extracted it to the most basic recreation that I can come up with and uploaded it here.
https://github.com/dusty/chakra-tester
The entire library consists of this:
import { Box, BoxProps, Button, useToast } from '@chakra-ui/core'
import React, { FC, forwardRef } from 'react'
export const MyBox: FC<BoxProps> = forwardRef(({ children, ...rest }, ref) => {
const toast = useToast()
const sendToast = () => {
toast({
status: 'success',
description: 'hello',
duration: 3000,
isClosable: true,
position: 'top-right',
})
}
return (
<Box ref={ref} {...rest}>
<Button onClick={sendToast}>{children}</Button>
</Box>
)
})
The example app trying to use this library consists of the following:
import { CSSReset, theme, ThemeProvider } from '@chakra-ui/core';
import { MyBox } from 'chakra-tester';
import * as React from 'react';
import * as ReactDOM from 'react-dom';
const App = () => {
return (
<ThemeProvider theme={theme}>
<CSSReset />
<MyBox>Hello</MyBox>
</ThemeProvider>
);
};
ReactDOM.render(<App />, document.getElementById('root'));
Does anyone have any ideas on this?
Issue Analytics
- State:
- Created 4 years ago
- Comments:6 (3 by maintainers)
I’ve got the same issue with my Webpack based library that uses Chakra-UI. I solved this problem with some minor fixes in my
webpack.config.js
:Also,
webpack-node-externals
can help too.Here is my config that is working with tsdx - although I’d love to give a try using just babel and tsc.
tsconfig.json
The example directory to run the code uses this package.json setup.