VirtualizedLists should never be nested inside plain ScrollViews with the same orientation because it can break windowing and other functionality - use another VirtualizedList-backed container instead.
See original GitHub issue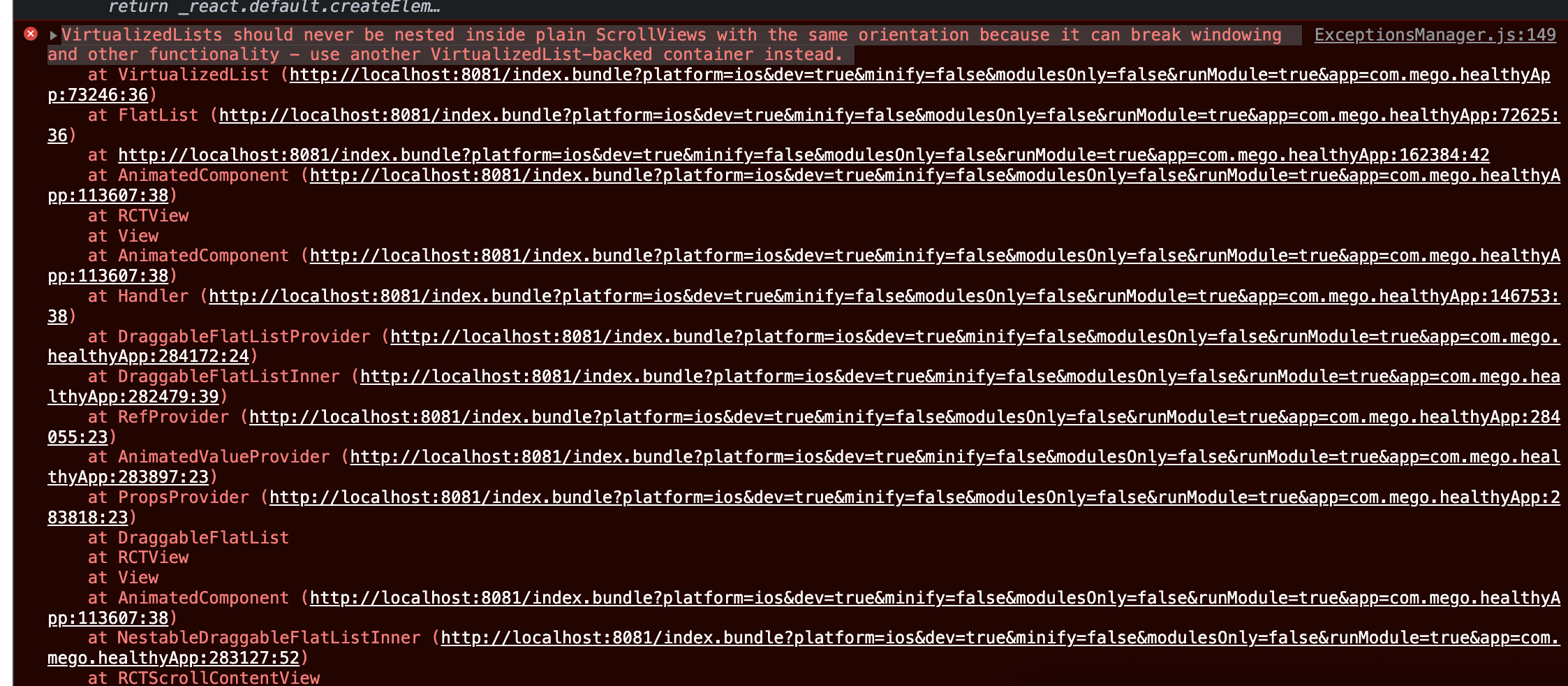
import React, { useEffect, useState } from ‘react’; import { useAppSelector, useAppDispatch } from ‘store/hook’; import { SafeAreaView } from ‘react-native-safe-area-context’; import { ScrollView, ImageBackground, Image, Modal, TouchableOpacity, Animated, PanResponder, GestureResponderEvent, PanResponderGestureState } from ‘react-native’; import { DragSortableView } from ‘react-native-drag-sort’; // import { Modal } from ‘@ant-design/react-native’; import MyStyleSheet from ‘components/MyStyleSheet’; import { UIText, UITextInput } from ‘components/Text/Texts’; import { Dimensions } from ‘react-native’; import { NestableScrollContainer, NestableDraggableFlatList, ScaleDecorator, RenderItemParams } from “react-native-draggable-flatlist”
export const WINDOW_WIDTH = Dimensions.get(‘window’).width;
export const WINDOW_HEIGHT = Dimensions.get(‘window’).height;
const NUM_ITEMS = 10;
function getColor(i: number) {
const multiplier = 255 / (NUM_ITEMS - 1);
const colorVal = i * multiplier;
return rgb(${colorVal}, ${Math.abs(128 - colorVal)}, ${255 - colorVal})
;
}
type Item = { key: string; label: string; height: number; width: number; backgroundColor: string; };
const initialData: Item[] = […Array(NUM_ITEMS)].map((d, index) => {
const backgroundColor = getColor(index);
return {
key: item-${index}
,
label: String(index) + “”,
height: 100,
width: 60 + Math.random() * 40,
backgroundColor,
};
});
const Index: React.FC<{ navigation: any }> = ({ navigation }) => { const [data, setData] = useState(initialData);
const renderItem = ({ item, drag, isActive }: RenderItemParams<Item>) => { console.log(666, item, drag, isActive); return ( <ScaleDecorator> <TouchableOpacity onLongPress={drag} disabled={isActive} style={[ styles.rowItem, { backgroundColor: isActive ? “red” : item.backgroundColor }, ]} > <UIText style={styles.text}>{item.label}</UIText> </TouchableOpacity> </ScaleDecorator> ); }; console.log(‘data’, data); console.log(‘renderItem’, renderItem); return ( <NestableScrollContainer> <NestableDraggableFlatList data={data} renderItem={renderItem} keyExtractor={(item) => item.key} onDragEnd={({ data }) => setData(data)} /> </NestableScrollContainer> ); };
const styles = MyStyleSheet.create({ rowItem: { height: 100, width: 100, alignItems: “center”, justifyContent: “center”, }, text: { color: “white”, fontSize: 24, fontWeight: “bold”, textAlign: “center”, }, });
export default Index;
Issue Analytics
- State:
- Created a year ago
- Reactions:4
- Comments:6
Same here, no matter what I tried with the components reordering etc. the warning won’t go away.