Mock Generation
See original GitHub issueI generate restful-react hooks from my openAPI spec. I’ve been looking into various ways of handling mocks and wanted to open a discussion about the typesafe solution I’ve found.
I first tried using jest to mock the hooks themselves, but that became dangerously complicated. I eventually moved on to mocking http requests. This was clean in that I wasn’t usurping any of restful-react’s behavior. The downside to this methodology was that it “leaks”. Developers now need to be aware of url paths which the restful-react codegen had previously abstracted from them. For example, I’d include a line like this in my component test:
nock("http://localhost")
.get(`/api/v1/rbac/users`)
.reply(code, response);
This is pretty fragile, as well as not having typesafety of the response baked in- meaning the mock behavior in the test can easily deviate from real-world behavior of a changing api.
I decided to look into writing a snippet to codegen my mocks- using the same types that restful-react generates for me. Here’s an example of one of the mocks I now generate:
type GetUsersResponses = {
200: RbacUsers;
404: UserNotFound
} & {[code: number]: Error};
export function mockGetUsers<T extends keyof GetUsersResponses>(code: T,response: GetUsersResponses[T]) {
return nock("http://localhost")
.get(`/api/v1/rbac/users`)
.reply(code, response);
}
This felt great. Not only am I able to use simple functions like mockGetUsers
in my component tests, but they stay type safe and up to date with the rest of the codegen. Additionally, we keep the url path abstracted from the developers.
As an added cool feature, the response type is a dependent type on the status code, so the TS compiler will yell at you, for example: if you try to mock a 404 response to a 200 status code:
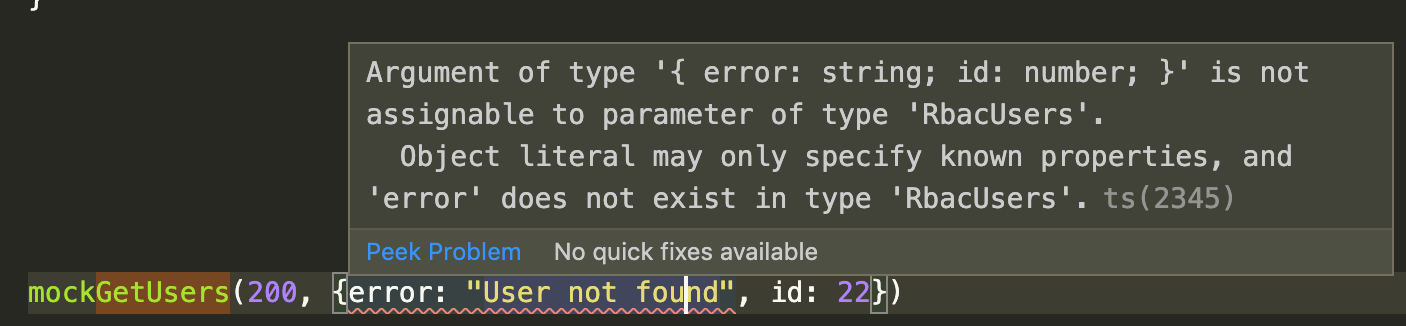

Here’s my proof-of-concept nodejs script to pull this off for responses. https://gist.github.com/seanlaff/ee4a07682bc74e3e4077a0ee394e6b15
Maybe it’s worthwhile to consider something like this as a feature of restful-react?
Issue Analytics
- State:
- Created 4 years ago
- Reactions:2
- Comments:7 (3 by maintainers)
Here’s my first pass at this for those that are interested. “Destructuring” the genericsString that is available to the generator was a little gymnastic-y @fabien0102 (I think I would have preferred them be individual params, rather than a comma delimmited string), but otherwise this is pretty powerful!
This will produce mock funcs like
while also doing dependent typing between the status code and the response body
How are you getting nock to work with fetch?