How to query a Graph and instantiate C# Classes with the data?
See original GitHub issueSuppose I have C# classes like Customer, Invoice, Invoice Items, and these have the corresponding Nodes on Neo4j Graph. How can I query the graph and instantiate these objects and the relations?
Now I do the Cypher Query to read this Graph and follow it the way I need, by grouping the main nodes, a foreach iterating the sub nodes grouping them, and instantiating the C# classes and putting the data on the classes using the Customer = client.Return<Customer>…
I am using this wonderful neo4j Driver, and need a better, cleaner, way to “transfer” my graph that I just queried into my C# classes and related classes. Please give me some light on this 😃 Is there some example online you can point to me?
This is the graph:
(Pessoa:Pessoa)-[:Possui]->(PeriodoAtividade:PeriodoAtividade)-[:Possui]->(Turno:Turno)
Suppose I query it using:
MATCH (Pessoa:Pessoa { id: "aac9f060-e838-48e9-8a42-0f1c22040abc" })-[:Possui]->(CoordenacaoPedagogica:CoordenacaoPedagogica)-[:Possui]->(PeriodoAtividade:PeriodoAtividade)
OPTIONAL MATCH (PeriodoAtividade)-[:Possui]->(Turno:Turno)
OPTIONAL MATCH (PeriodoAtividade)-[:Possui]->(UnidadeLetiva:UnidadeLetiva)
RETURN CoordenacaoPedagogica AS CoordenacaoPedagogica, PeriodoAtividade AS PeriodoAtividade, UnidadeLetiva AS UnidadeLetiva, Turno AS Turno
ORDER BY Turno.Titulo, UnidadeLetiva.Titulo
The classes look like this:
[AddINotifyPropertyChangedInterface]
public class CoordenacaoPedagogica
{
public class Dados : IDados
{
public Guid id { get; set; } = Guid.NewGuid();
public Guid TenantID { get; set; }
}
public Dados Propriedades { get; set; } = new Dados();
[Relacao("Possui", DirecaoRelacao.In)]
public Pessoa.Pessoa Empresa { get; set; }
[Relacao("Possui", DirecaoRelacao.Out)]
public ObservableCollection<PeriodoAtividade> PeriodoAtividade { get; set; } = new ObservableCollection<PeriodoAtividade>();
}
[AddINotifyPropertyChangedInterface]
public class PeriodoAtividade
{
public class Dados : IDados
{
public Guid id { get; set; } = Guid.NewGuid();
public Guid TenantID { get; set; }
public DateTime DataHoraInicial { get; set; }
public DateTime DataHoraFinal { get; set; }
public string Titulo { get; set; } // 2020 ou I Semestre 2020 ou Semi-Extensivo 2020
}
public Dados Propriedades { get; set; } = new Dados();
[Relacao("Possui", DirecaoRelacao.In)]
public CoordenacaoPedagogica CoordenacaoPedagogica { get; set; }
[Relacao("Possui", DirecaoRelacao.Out)]
public ObservableCollection<UnidadeLetiva> UnidadesLetivas { get; set; } = new ObservableCollection<UnidadeLetiva>();
[Relacao("Possui", DirecaoRelacao.Out)]
public ObservableCollection<Turno> Turnos { get; set; } = new ObservableCollection<Turno>();
}
public class UnidadeLetiva
{
public class Dados : IDados
{
public Guid id { get; set; }//= Guid.NewGuid();
public Guid TenantID { get; set; }
public DateTime DataHoraInicial { get; set; }
public DateTime DataHoraFinal { get; set; }
public string Titulo { get; set; } // 2020 ou I Semestre 2020 ou Semi-Extensivo 2020
}
public Dados Propriedades { get; set; } = new Dados();
[Relacao("Possui", DirecaoRelacao.In)]
public PeriodoAtividade PeriodoAtividade { get; set; }
[Relacao("Possui", DirecaoRelacao.Out)]
public ObservableCollection<Avaliacao> Avaliacoes { get; set; } = new ObservableCollection<Avaliacao>();
}
[AddINotifyPropertyChangedInterface]
public class Turno
{
public class Dados : IDados
{
public Guid id { get; set; } = Guid.NewGuid();
public Guid TenantID { get; set; }
public string Titulo { get; set; } // exemplo Matutino, Vespertino, Noturno
public DateTime DataHoraInicial { get; set; }
public DateTime DataHoraFinal { get; set; }
}
public Dados Propriedades { get; set; } = new Dados();
[Relacao("PeriodoAtividade", DirecaoRelacao.In)]
public PeriodoAtividade PeriodoAtividade { get; set; }
[Relacao("Possui", DirecaoRelacao.Out)]
public ObservableCollection<Turma> Turmas { get; set; } = new ObservableCollection<Turma>();
}
Now my code to instantiate the classes looks like this:
public static async Task<CoordenacaoPedagogica> PegaCoordenacaoPedagogica(Guid PessoaID)
{
using (var client = await neo4jh.TryGetClientConnectedAsync())
{
var q = client.Cypher
.Match(
"(Pessoa:Pessoa { id: {PessoaID} })-[:Possui]->(CoordenacaoPedagogica:CoordenacaoPedagogica)-[:Possui]->(PeriodoAtividade:PeriodoAtividade)"
)
.OptionalMatch("(PeriodoAtividade)-[:Possui]->(Turno:Turno)")
.OptionalMatch("(PeriodoAtividade)-[:Possui]->(UnidadeLetiva:UnidadeLetiva)")
.WithParam("PessoaID", PessoaID)
.Return
(
(CoordenacaoPedagogica, PeriodoAtividade, UnidadeLetiva, Turno) => new
{
CoordenacaoPedagogica = CoordenacaoPedagogica.As<CoordenacaoPedagogica.Dados>(),
PeriodoAtividade = PeriodoAtividade.As<PeriodoAtividade.Dados>(),
UnidadeLetiva = UnidadeLetiva.As<UnidadeLetiva.Dados>(),
Turno = Turno.As<Turno.Dados>()
}
)
.OrderBy("Turno.Titulo, UnidadeLetiva.Titulo")
;
var r = await q.ResultsAsync;
var Pessoa = new Pessoa();
var list_CoordenacaoPedagogica = (
from result in r
group result by result.CoordenacaoPedagogica.id into g
select new { ItemId = g.Key, SubItems = g }
).ToList();
foreach (var gCoordenacaoPedagogica in list_CoordenacaoPedagogica)
{
var CoordenacaoPedagogica_ = new CoordenacaoPedagogica()
{
Propriedades = list_CoordenacaoPedagogica.First().SubItems.First().CoordenacaoPedagogica
};
Pessoa.CoordenacoesPedagogicas.Add(CoordenacaoPedagogica_);
var list_PeriodoAtividade = (from PeriodoAtividade in gCoordenacaoPedagogica.SubItems
group PeriodoAtividade by PeriodoAtividade.PeriodoAtividade.id into g
select new { ItemId = g.Key, SubItems = g }
).ToList();
foreach (var gPeriodoAtividade in list_PeriodoAtividade)
{
PeriodoAtividade Periodo = new PeriodoAtividade()
{
Propriedades = gPeriodoAtividade.SubItems.First().PeriodoAtividade
};
CoordenacaoPedagogica_.PeriodoAtividade.Add(Periodo);
var list_Turno = (from Turno in gPeriodoAtividade.SubItems
group Turno by Turno.Turno.id into g
select new { ItemId = g.Key, SubItems = g }
).ToList();
foreach (var gTurno in list_Turno)
{
var Turno = new Turno()
{
Propriedades = gTurno.SubItems.First().Turno
};
Periodo.Turnos.Add(Turno);
}
var list_UnidadeLetiva = (from UnidadeLetiva in gPeriodoAtividade.SubItems
where UnidadeLetiva.UnidadeLetiva?.id != Guid.Empty
group UnidadeLetiva by UnidadeLetiva.UnidadeLetiva?.id into g
select new { ItemId = g.Key, SubItems = g }
).ToList();
foreach (var gUnidadeLetiva in list_UnidadeLetiva)
{
var ul = gUnidadeLetiva.SubItems.First().UnidadeLetiva;
if (ul != null)
{
var UnidadeLetiva = new UnidadeLetiva()
{
Propriedades = ul
};
Periodo.UnidadesLetivas.Add(UnidadeLetiva);
}
}
}
}
return Pessoa.CoordenacoesPedagogicas.First();
}
}
Issue Analytics
- State:
- Created 3 years ago
- Comments:8 (5 by maintainers)
Hey @TonyHenrique Sorry for the delay in this - I missed it 😦
I have this graph: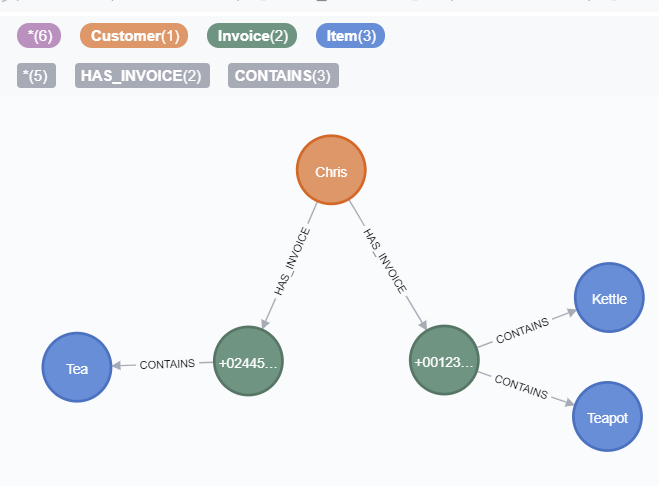
These classes:
Which I fill up with this code:
Which gives me this:
Is this doing what you wanted?
The Cypher query is:
To instantiate a DB - you can use:
I’m going to close this now - please reopen if you need to!