The global style should be at the top
See original GitHub issueCurrent behavior:
When there is more than one emotion cache I want the global style to always be at the top and then be overridden in order
The prepend
option can only be set to the top or bottom, and the insertionPoint
option needs to be passed HTMLElement
which does not support SSR

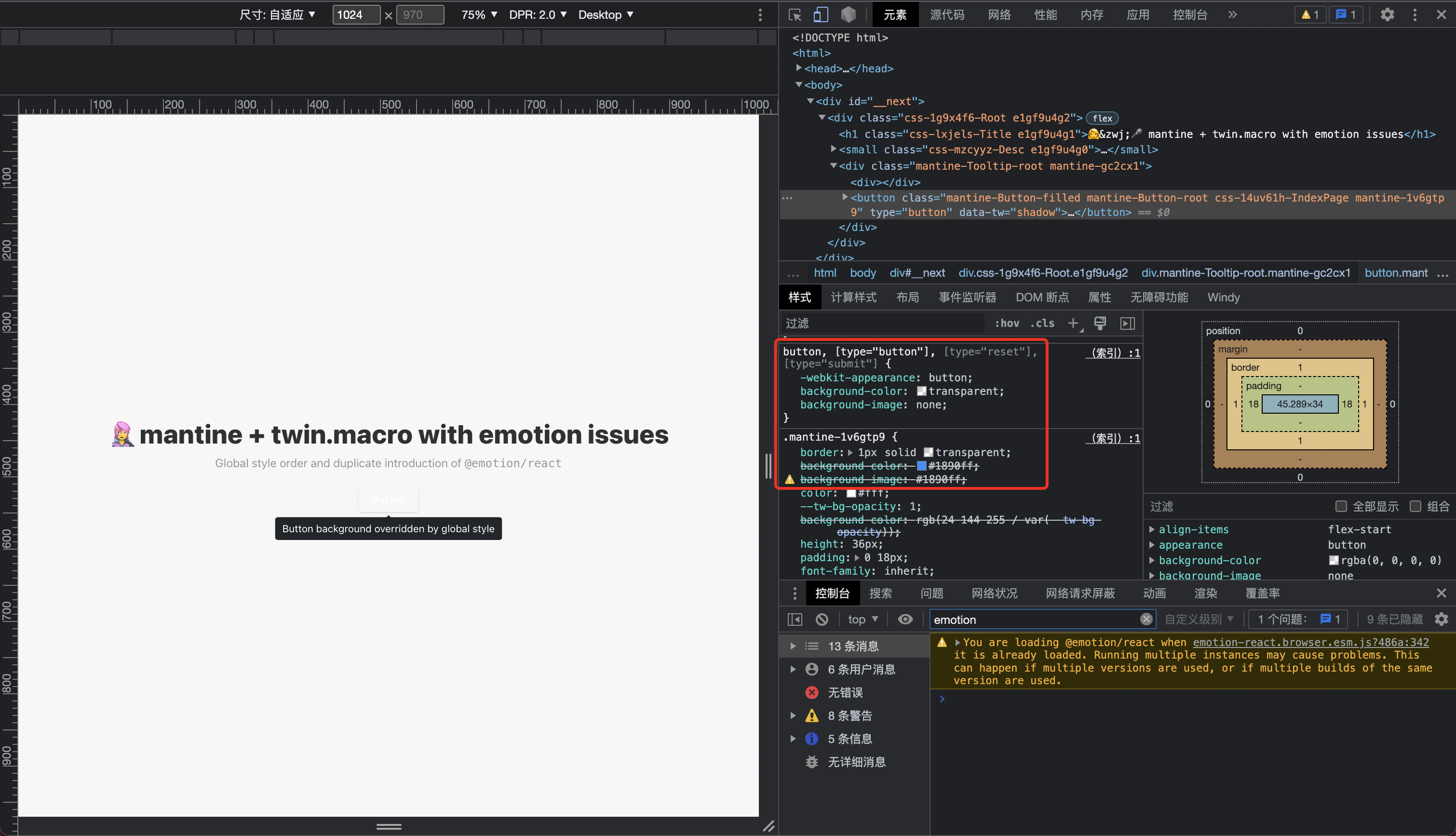
I can adjust the order of ServerStyles
here, but there’s no way to override the mantine
style directly
MyDocument.getInitialProps = async (ctx: DocumentContext) => {
const initialProps = await Document.getInitialProps(ctx)
const server = createEmotionServer(cache)
return {
...initialProps,
styles: (
<>
{initialProps.styles}
<ServerStyles html={initialProps.html} server={stylesServer} />
<ServerStyles html={initialProps.html} server={server} />
</>
),
}
}
To reproduce:
https://github.com/u3u/emotion-issue-2803
Expected behavior:

Environment information:
react
version: 18.2.0@emotion/react
version: 11.9.3
Issue Analytics
- State:
- Created a year ago
- Comments:7 (4 by maintainers)
Top Results From Across the Web
Global and local styling - Every Layout
This section will contextualize layout components in a larger system that includes global styles. What are global styles? When people talk about the...
Read more >How to Create Global Styles with Styled Components
How to Create Global Styles with Styled Components · Step 1: Create a global styles file · Step 2: Place GlobalStyle at the...
Read more >Standard Styling with Global CSS Files - Gatsby
The best way to add global styles is with a shared layout component. ... You should see your global styles taking effect across...
Read more >How to manage the basic settings of Global Style | Help Center
Step 2: Click on the Global Style tab at the top of the right sidebar. Next, hit the “Create New Preset” button. You...
Read more >Creating a Global Style Sheet - meyerweb.com
What HTML tags will I use over and over again? Are there certain colors I want to use, and if so, which ones...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
Top Related Hashnode Post
No results found
You can use this patch to work around your issue:
patch for the repro
@Andarist Thank you! This problem has been solved 😁