Wrong unauthorized WebSocket status code
See original GitHub issueChecklist
- The bug is reproducible against the latest release and/or
master
. - There are no similar issues or pull requests to fix it yet.
Describe the bug
I have a protected websocket endpoint, with the decorator @requires('authenticated', status_code=401)
.
If the authentication check fails, the response code from the websocket is 403 Forbidden
, but it should be 401 Unauthorized
.
To reproduce
Here an example to reproduce the use case.
import uvicorn
from starlette.applications import Starlette
from starlette.authentication import AuthenticationBackend, AuthenticationError, AuthCredentials, SimpleUser, requires
from starlette.middleware import Middleware
from starlette.middleware.authentication import AuthenticationMiddleware
from starlette.websockets import WebSocket
class BasicAuthBackend(AuthenticationBackend):
async def authenticate(self, request):
auth = request.headers.get("Authorization", None)
if auth != 'sample_key':
raise AuthenticationError('Invalid auth credentials')
return AuthCredentials(["authenticated"]), SimpleUser("username")
app = Starlette(
middleware=[
Middleware(AuthenticationMiddleware, backend=BasicAuthBackend()),
],
)
@app.websocket_route("/websocket")
@requires("authenticated", status_code=401)
async def websocket(websocket: WebSocket):
await websocket.accept()
await websocket.send_json({'message': 'Connected and authenticated!'})
await websocket.close()
if __name__ == '__main__':
uvicorn.run("websocket:app", host="0.0.0.0", port=10123)
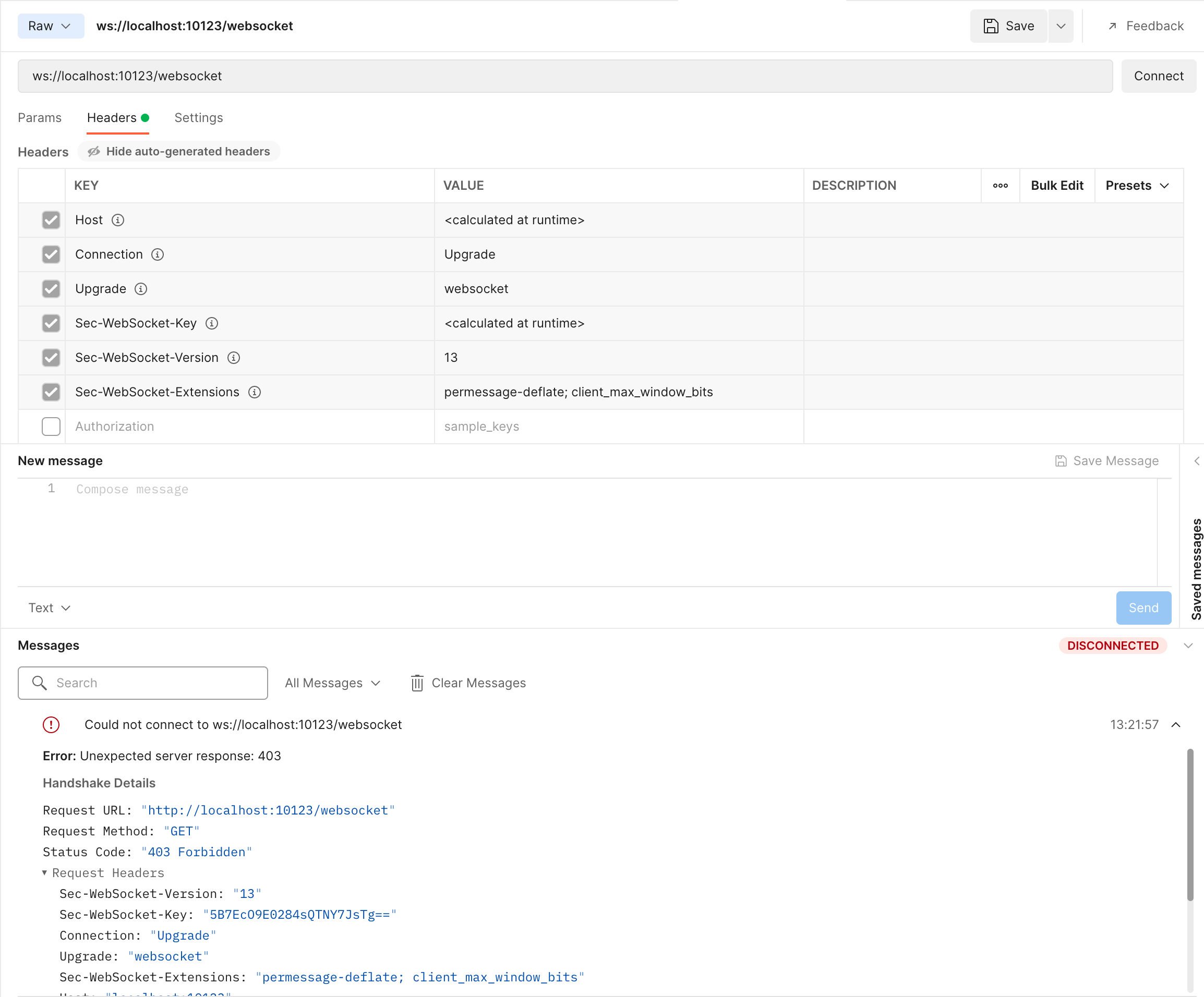
Expected behavior
The websocket endpoint should return 401, according to the status_code specified in the @requires
decorator.
Actual behavior
The websocket endpoint should return 403, even if I specify the desired status_code in the @requires
decorator.
Environment
- OS: Linux
- Python version: 3.8
- Starlette version: 0.17.1
Issue Analytics
- State:
- Created 2 years ago
- Reactions:2
- Comments:8 (6 by maintainers)
Top Results From Across the Web
How to tell websocket client that authentication failed
Chrome network tab shows no messages received, and the close event has code 1006 (CLOSE_ABNORMAL), with no other information. How do I pass...
Read more >80362 – WebSocket: Client does not support 401 ...
When opening a WebSocket connection to a server that requires authentication, the connection errors out with "Unexpected response code: 401".
Read more >Websocket Shutdown Codes — Swindon 0.7.8 documentation
Here are codes that you can see in onclose event handler: var ws = new WebSocket(..) os.onclose = function(ev) { console.log(ev.code, ev.reason) }....
Read more >401 Unauthorized - HTTP - MDN Web Docs
The HyperText Transfer Protocol (HTTP) 401 Unauthorized response status code indicates that the client request has not been completed ...
Read more >HTTP Status Codes List | HTTP Error Codes Explained
A 401 Unauthorized error status code indicates that the request does not include the appropriate authentication credentials, authentication has failed, or the ...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
I guess they are related, they point to the same code but not exactly the same issue. I think this issue is here where we hard-coded http response to 403, and the
@requires
in Starlette has no effect on http response.We are going to document this behavior first on https://github.com/encode/starlette/pull/1636.
At some point, I’ll take a look at the implementation of the WebSocket Denial Response by @paulo-raca. There are a couple of things in the list before that, but we are going to get there - I’m not confirming we are going to have the extension, it needs to be discussed, and we need to see if it’s worth it.
Note: An implementation on the uvicorn needs to take place as well.