cookieParser.signedCookie seems to not work properly
See original GitHub issueHello everyone. I’m trying to “decode” a signed cookie I receive in a websocket session. First, here is how I setup my express session with cookie-parser:
static.ts file
import * as cookieParser from 'cookie-parser';
import * as express from 'express';
import * as session from 'express-session';
const app = express();
app.use(cookieParser('foobarbaz1234567foobarbaz1234567'));
app.use(session({
cookie: {
httpOnly: true,
maxAge: 600000, // 3600000 for 1 hour & 600000 for 10 minutes
secure: process.env.VSCodeDebug && process.env.VSCodeDebug === 'true' ? false : true,
},
genid: () => genuuid(),
resave: false,
saveUninitialized: false,
secret: 'foobarbaz1234567foobarbaz1234567',
store: mystore,
}));
Somewhere in my code, when I receive a websocket connection, I’m able to get the signed cookie. But I want to decode it in order to have the session ID stored inside.
This is what I’m trying to do:
As you can see, i’m using the same secret password with “signedCookie” than in the cookieParser initialization. The result of signedCookie returns everytime the same string as the signed cookie (temp3 value in my current example). And according to your documentation:
it kinda says that the signature is invalid or something like that. Is it possible that I’m missing something in my approch ? In my database where the cookie is stored, I can see the decoded cookie (i.e the session ID). So I guess that something looks wrong with “signedCookie” function (or maybe with my approch).
You can see bellow what I have in my request parameter. Also, bellow, you can see what I observe when I’m trying to user signedCookie function. The output of this function is the same as the input (signedSession). And the secret is the same as in the cookie-parser configuration.
Here is what I have in my webSocketSingleton.ts file
import * as cookieParser from 'cookie-parser';
Here is what I observe when using the signedCookie function
Here is the content of the “req” parameter
Can you help me please to resolve this issue ? Thank you in advance for your answer
Issue Analytics
- State:
- Created 5 years ago
- Comments:5 (3 by maintainers)
Nowadays you need to decode the cookie value using
decodeURIComponent()
before using any of the following functions:Because it doesn’t decode itself inside the function, now they just check if it starts with ‘j:’ or ‘s:’ (before, when this issue was created, it required an encodedURI and it would check if it starts with ‘j%3A’, for JSONCookie, or ‘s%3A’, for signedCookie, and then both of these functions would decode the input to continue their codes)
these prints are from the actual expressjs/cookie-parser source code
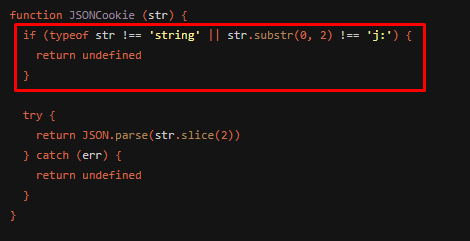
Conclusion: You need to use decodeURIComponent() before using JSONCookie(), JSONCookies(), signedCookie() or signedCookies() otherwise it will return the input value.
Example:
Taking the cookie from the last screenshot (please if you could send as text, as it look a really long time to type it out correctly 😃 ), here is the flow of unsigning the cookie, as an example:
Basically:
(1) Parse the cookie header and get
connect.sid
value (2) Pass to signedCookie with signature