Jest does not allow asynchronous catching of rejected promises
See original GitHub issueBug
Jest version: 22.4.3
(But was introduced in 21.x
)
Config: None (all defaults)
Node version: 6.11.5
, 8.9.4
Jest does not allow you to asynchronously attach a .catch()
to a promise, even if it’s added before the test itself is finished. The only difference between these two tests is that the catch is added within a setTimeout() in the second one.
// This passes after ~107ms (the expected amount of time)
test('Synchronous catching of promise', (done) => {
const promise = Promise.reject(new Error('nope'));
promise.catch((err) => console.log('Caught error: ', err.message));
// At a later time, finish the test
setTimeout(() => {
console.log('here');
done();
}, 100);
});
// This fails after ~10ms, with error message "nope"
test('Async catching of promise', (done) => {
const promise = Promise.reject(new Error('nope'));
setTimeout(() => {
promise.catch((err) => console.log('Caught error: ', err.message));
});
// At a later time (well after the catch() has been attached to the promise), finish the test
setTimeout(() => {
// We nver makes it to this point because Jest sees that promise was not caught immediately
console.log('here');
done();
}, 100);
});
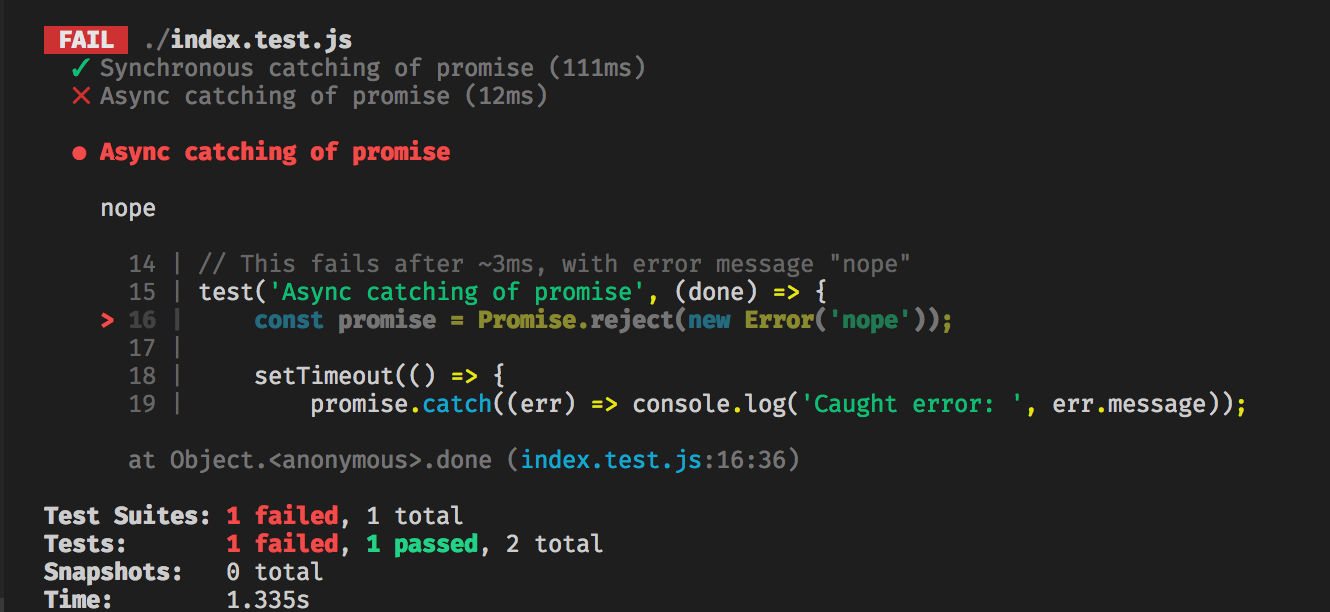
In Jest 20.x
, only warnings are printed, and the test does not fail
(node:54613) UnhandledPromiseRejectionWarning: Unhandled promise rejection (rejection id: 2): Error: nope
(node:54613) PromiseRejectionHandledWarning: Promise rejection was handled asynchronously (rejection id: 2)
(Was originally mentioned in https://github.com/facebook/jest/issues/3251)
Issue Analytics
- State:
- Created 5 years ago
- Reactions:7
- Comments:11
Top Results From Across the Web
Testing Asynchronous Code - Jest
If you expect a promise to be rejected, use the .catch method. Make sure to add expect.assertions to verify that a certain number...
Read more >Testing promise rejection in JavaScript with Jest - Codeleak.pl
It looks like using try-catch with async/await is the easiest way to achieve this as the rejected value is thrown: it("rejects (bad)", async...
Read more >Ignore a rejected fire-and-forget promise in jest - Stack Overflow
My function calls a private async function in a fire-and-forget manner, and does not add any error handling. Don't do that.
Read more >Handling those unhandled promise rejections with JS async ...
by rejecting a promise which was not handled with .catch(). The solution is simple, either use .catch() as suggested by the message:
Read more >Jest Unhandled Promise Rejection - Guide Fari
I was mocking an async function, & was testing for what would happen if that promise got rejected, ie an unsuccessful call.
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Nice idea! I reworked it into a
defuse
function:Still, this is a workaround for a problem that I believe should be fixed.
What’s more, the code may not even be part of the test suite, but of the system under test. Consider:
code-under-test.js
test.js
The function
codeUnderTest
is perfectly valid, yet fails any unit test that attempts to call it. Is there any workaround?