Testing atom state in Jest (React Testing Library) after event inside the component.
See original GitHub issueHi all. I’m trying to test a component called CSV
, which has two radio buttons that change the value of an atom. I have created the following test.
import { fireEvent, render } from '@testing-library/react'
import { RecoilRoot, snapshot_UNSTABLE } from 'recoil'
import { csvExportTypeAtom } from '../csv.atoms'
describe('CSV should', () => {
test('select the first radio button.', () => {
const { getByTestId } = render(
<RecoilRoot>
<CSV />
</RecoilRoot>,
)
const component = getByTestId('radio_button_marker_standard')
fireEvent.click(component)
const snapshot = snapshot_UNSTABLE()
expect(snapshot.getLoadable(csvExportTypeAtom).valueOrThrow()).toBe('standard')
})
})
I get the idea that I’m getting a snapshot from a different atom due to the RecoilRoot
context, so I don’t see the updated value after the click event.
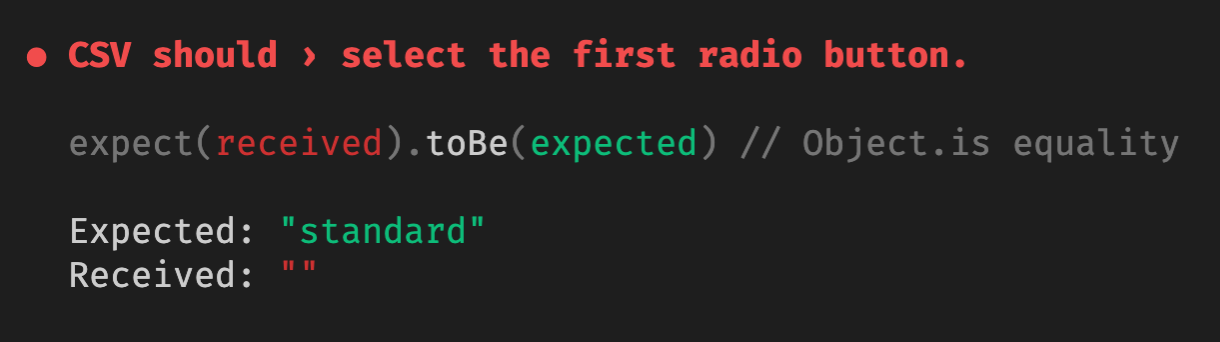
How can I access the atom used inside of the RecoilRoot
that receives the event?
Is there a better way to do this?
Please, help! I’m new to Recoil, and this is driving me crazy 🙇♂️
Issue Analytics
- State:
- Created 2 years ago
- Comments:5 (2 by maintainers)
Top Results From Across the Web
Testing components with @testing-library/react example #128
I'm especially wondering how to mock out/simplify setting up global state that's accessed via recoil hooks. For example useRecoilState as below.
Read more >How To Test a React App with Jest and React Testing Library
In this tutorial, you will test asynchronous code and interactions in a sample project containing various UI elements. You will use Jest to ......
Read more >Testing - Recoil
It can be helpful to test Recoil state when testing a component. You can compare the new state against expected values using this...
Read more >What is the pattern for ensuring a user action triggers a Recoil ...
I can imagine rendering that component under test inside a custom wrapper that sets the whole state as the value of an INPUT...
Read more >Testing with react-testing-library and Jest | by Chidume Nnamdi
We can test for DOM events in our React components using react-testing-library. DOM events like: ... Let's refactor our App component so we...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Sure! It would be really useful to test the state of the atoms/selectors used by a component because the user’s action can be tested easily by observing the changes the component does in the state. If you want @drarmstr, I could add this solution to the documentation, illustrated with a simple example: https://github.com/facebookexperimental/Recoil/pull/1198.
In the end, I have implemented an observer as explained here. I pass the atom/selector to be observed and the
onChange
function to be called when the state changes.So I can pass a
jest.fn()
and check whatever I need from the returned value.And it works great 🙂 Thanks a lot @drarmstr.