[React Native] Error on submitting the form - event.preventDefault() is not a function
See original GitHub issueAre you submitting a bug report or a feature request?
Bug Report - similar to #83
What is the current behavior?
I have a form like so:
export default class extends Component<any> {
displayName = 'MyForm';
render() {
return (
<Form
onSubmit={this.props.onSubmit}
render={({ handleSubmit, values }) => (
<View>
<RowView // styled-component
style={{ ... }}
>
<Text style={{ fontSize: 16, fontWeight: 'bold' }}>Label:</Text>
<Field
component={() => (
<TextInput
placeholder={'$00.00'}
keyboardType="numeric"
style={styles.textInput}
/>
)}
/>
</RowView>
<TouchableOpacity onPress={() => handleSubmit(values)}>
<Text>Submit</Text>
</TouchableOpacity>
</View>
)}
/>
);
}
}
Which I then use in a container:
class MyContainer extends Component {
handleOnSubmit = (values) => {
alert(JSON.stringify(values));
};
render() {
return (
<KeyboardAvoidingView style={styles.container}>
<MyForm onSubmit={this.handleOnSubmit} />
</KeyboardAvoidingView>
);
}
}
Unfortunately this throws an error when I try to submit the form, with the same error as mentioned in #83.
What is the expected behavior?
Should submit form without any errors regarding the event
object.
Sandbox Link
https://snack.expo.io/r1Dj7J6vM || https://snack.expo.io/S1IY_JawG
What’s your environment?
"react": "16.0.0-beta.5",
"react-final-form": "^3.1.0",
"react-native": "0.49.3",
Other information
I see that error is coming from this function over here:
_this.handleSubmit = function (event) {
event && event.preventDefault();
// console.log('event: ', JSON.stringify(event));
return _this.form.submit();
};
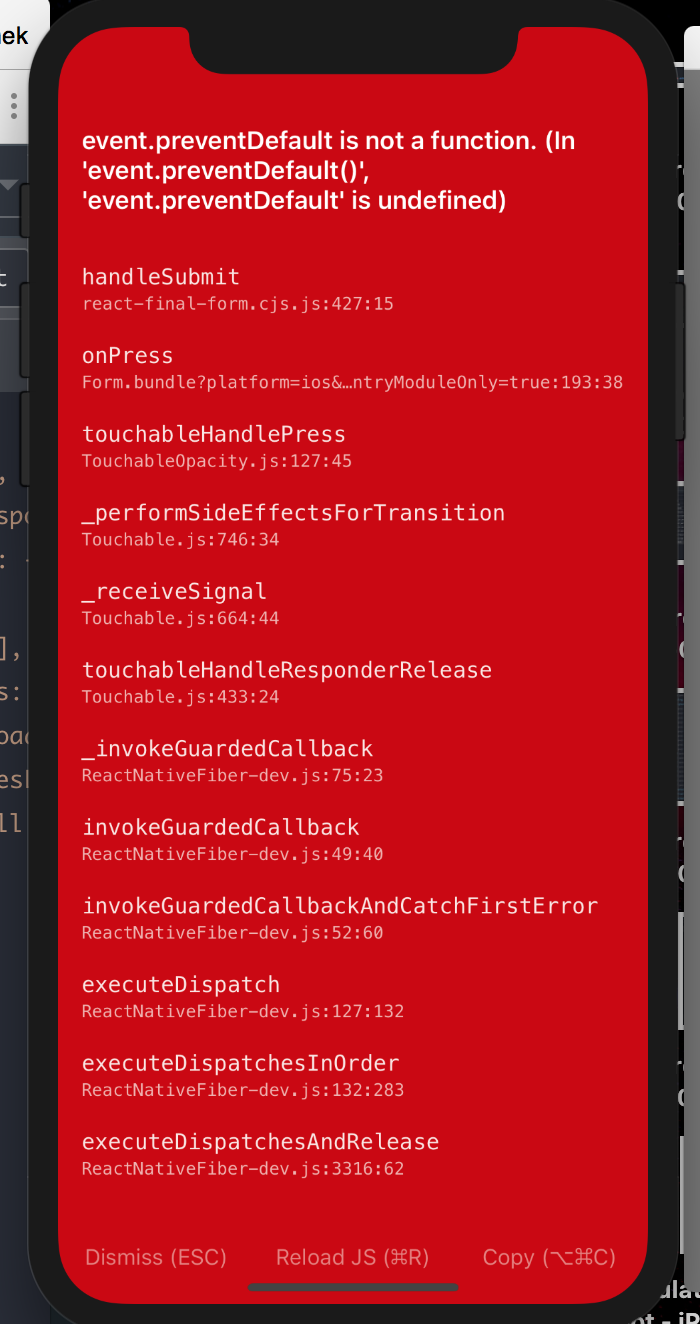
I am not really sure if I am doing anything wrong or using any of the components from the library wrongly.
Issue Analytics
- State:
- Created 6 years ago
- Comments:6 (3 by maintainers)
Top Results From Across the Web
TypeError: e.preventDefault is not a function in react
The event handlers in react are expecting a function, not a function call like in HTML. When you do handleSubmit(sendprop) , you're calling...
Read more >preventDefault() Event Method - W3Schools
The preventDefault() method cancels the event if it is cancelable, meaning that the default action that belongs to the event will not occur....
Read more >useForm - handleSubmit - React Hook Form
This function will receive the form data if form validation is successful. Props. Name, Type, Description. SubmitHandler, (data: Object, e?: Event) => void ......
Read more >SyntheticEvent - React
This reference guide documents the SyntheticEvent wrapper that forms part of React's Event System. See the Handling Events guide to learn more.
Read more >React submit form returns event.preventDefault is not a ...
Coding example for the question React submit form returns event.preventDefault is not a function-Reactjs.
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
The line in question is:
event && event.preventDefault()
.In order for you to get that error,
event
must be truthy, butevent.preventDefault
not be a function. Perhaps it needs to beevent && event.preventDefault && event.preventDefault()
?Published fix in
v3.1.1
.