Add Cors to callable function
See original GitHub issueI wrote a callable function to invite new users for my app:
exports.UserInvitation = functions.https.onCall((data, context) => {
const email = data.email
const password = passwordGenerator.generate({
length: 12,
numbers: true
})
console.log('Inviting user:', email)
const mailOptions = {
from: '',
to: email,
subject: ``,
html: utils.formatEmail(`
Hello, you've been invited to use my app.
`)
}
return new Promise((resolve, reject) => {
admin.auth().createUser({
email: email,
emailVerified: false,
password: password
}).then((user) => {
console.log(`Created user with uid ${user.uid}`)
transporter.sendMail(mailOptions).then(() => {
console.log(`Email sent to ${email}`)
resolve()
})
}).catch((err) => {
console.error(err.code)
reject(new functions.https.HttpsError(err.code, err.message))
})
})
})
I call it like this in my app:
functions.httpsCallable('UserInvitation')({ email: this.input.value })
But I’m getting “No Access Control Allow Origin” error when trying to call it from client. How can I fix this if I don’t have access to req/res objects inside onCall, to enable cors?
Issue Analytics
- State:
- Created 5 years ago
- Reactions:13
- Comments:44 (2 by maintainers)
Top Results From Across the Web
Firebase Callable Function + CORS - Stack Overflow
Go to the cloud function tab · Select your cloud function (check box) · Click "Add members" under Permissions tab in the right...
Read more >CORS issue with https callable function : r/Firebase - Reddit
Hi everyone. Been stuck on this issue for a couple days, searching the web and can't seem to find a solution.
Read more >Call functions via HTTP requests | Cloud Functions for Firebase
Configuring CORS (Cross-Origin Resource Sharing) ... Use the cors option to control which origins can access your function. By default, HTTP functions don't...
Read more >You can give this one a read. It's unlikely to be a CORS issue ...
It's unlikely to be a CORS issue for onCall function.. https://stackoverflow.com/questions/50278537/firebase-callable-function-cors.
Read more >google cloud function has been blocked by cors policy
If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled. Open side panel....
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
I recently faced the CORS error only for newly created functions. After hours of desperate search I found that newly created functions don’t have Cloud Functions Invoker at https://console.cloud.google.com/functions while the old ones have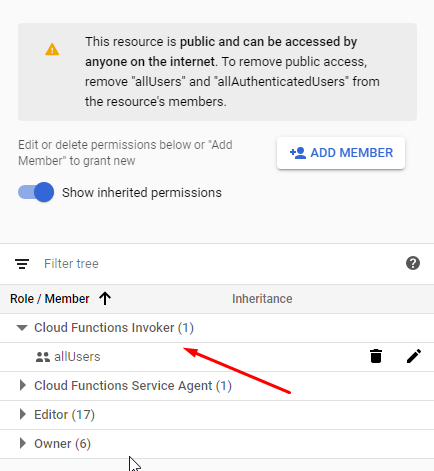
allUsers
:Here firebase mentions that starting from Jan 15, 2020 no allUsers is set anymore https://cloud.google.com/functions/docs/securing/managing-access-iam
@tricaricom @nicholmikey The CORS error in console with callable functions only indicates there is an error in the cloud function. Check the logs inside the Firebase console and you’ll see the actual error. CORS is not applicable for callable functions even when running on localhost