A testing bug in FCC's React Simple Counter Challenge
See original GitHub issueI found a subtle bug while doing the “React: Write a Simple Counter” challenge.
If you look at the code below you’ll see that I binded this in this.reset to the this .decrement method. It should be:
this.reset = this.reset.bind(this);
The consequence of this error is that clicking the reset button which calls this.reset does not reset the counter and decrements it instead.
However, when I run the tests, all the tests are passed and the error is not detected!
I think we need to add an additional test for that specific scenario.
Link to the page with the problem:
My buggy code
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
// change code below this line
this.increment = this.increment.bind(this);
this.decrement = this.decrement.bind(this);
this.reset = this.decrement.bind(this); //Wrong binding
// change code above this line
}
// change code below this line
increment(){
const count = this.state.count + 1;
this.setState({count});
}
decrement(){
const count = this.state.count - 1;
this.setState({count});
}
reset(){
const count = 0;
this.setState({count});
}
// change code above this line
render() {
return (
<div>
<button className='inc' onClick={this.increment}>Increment!</button>
<button className='dec' onClick={this.decrement}>Decrement!</button>
<button className='reset' onClick={this.reset}>Reset</button>
<h1>Current Count: {this.state.count}</h1>
</div>
);
}
};
browser and operating system
- Browser Name: Chrome
- Browser Version: 67
- Operating System: Mac OS X 10_13_3
Issue Analytics
- State:
- Created 5 years ago
- Comments:6 (5 by maintainers)
Top Results From Across the Web
Bug in last test - Write a Simple Counter - JavaScript
Tell us what's happening: There's a bug with the last test. It fails unless the increment and decrement both are already working.
Read more >How to troubleshoot and fix React bugs fast [step ... - Applitools
I've been playing around with Applitools for quite some time now, and I'd like to share what I've found to help you quickly...
Read more >Koleen Paunon's 100-Days-Of-React journey - GitHub Pages
Had a clear understanding of basic React props and implemented it on ... Challenge right now is the manipulation of array objects -...
Read more >How to create a simple counter Using ReactJS?
React is a front-end, open-source JavaScript library that is used to ... We'll be creating a simple application where we have 2 buttons...
Read more >Bug Hunting in Greenborder Pro - ITPro Today
Well-known security professionl Bill Stout posted a message to the Full Disclosure mailing list asking for help debugging Greenborder Pro.
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
This looks pretty good to me. I see you’ve made a PR, that’s great, we’ll QA that soon
@thecodingaviator In terms of the buggy code provided in this issue, I think only the last test case is wrong.
I checked if my changes work on local host.
After I made the change, we can see that the last test fails when I provide the wrong solution codes.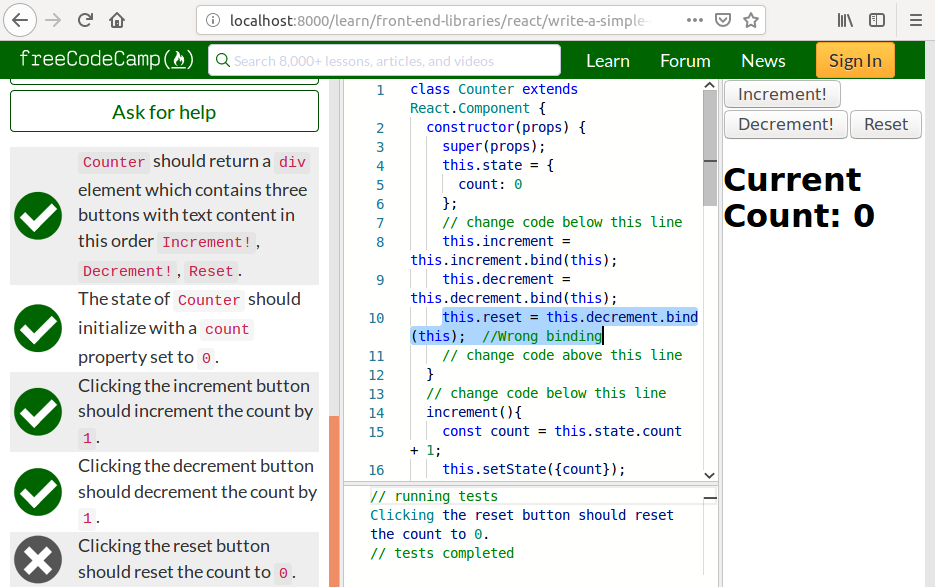
And the last test passes when I provide the correct solution codes.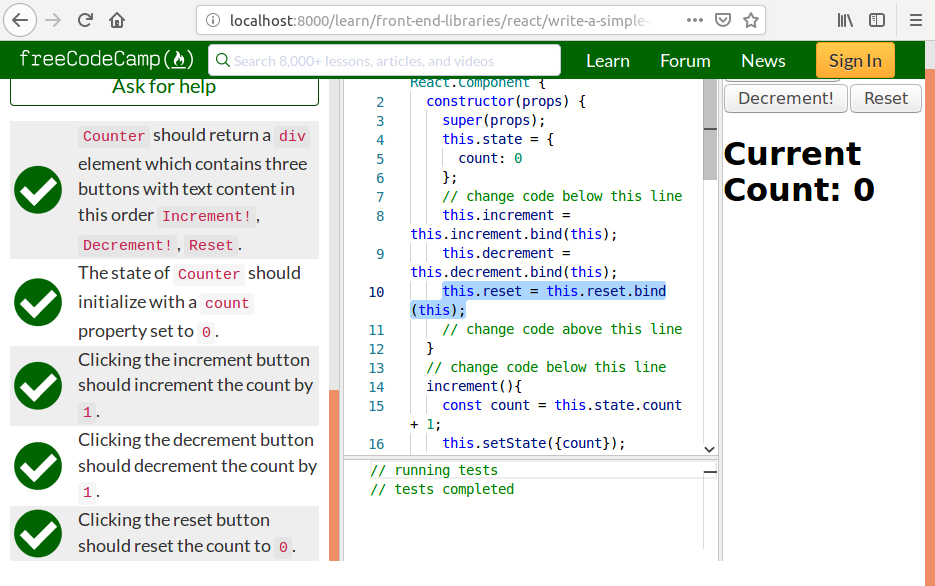