Weird Instructions -> Add Elements to the End of an Array Using concat Instead of push
See original GitHub issueAffected page
Your code
function nonMutatingPush(original, newItem) {
// Only change code below this line
return original.concat([newItem]); //[ 1, 2, 3, [ 4, 5 ] ]
// Only change code above this line
}
const first = [1, 2, 3];
const second = [4, 5];
nonMutatingPush(first, second);
Expected behavior
Since newItem, which references an array should be added to the original array (per the instructions), I expected to pass the test. Instead it says “nonMutatingPush([1, 2, 3], [4, 5]) should return [1, 2, 3, 4, 5].”, which would be concatenating the two arrays, but it isn’t a non mutating alternative to .push()
as the instructions suggest.
Screenshots
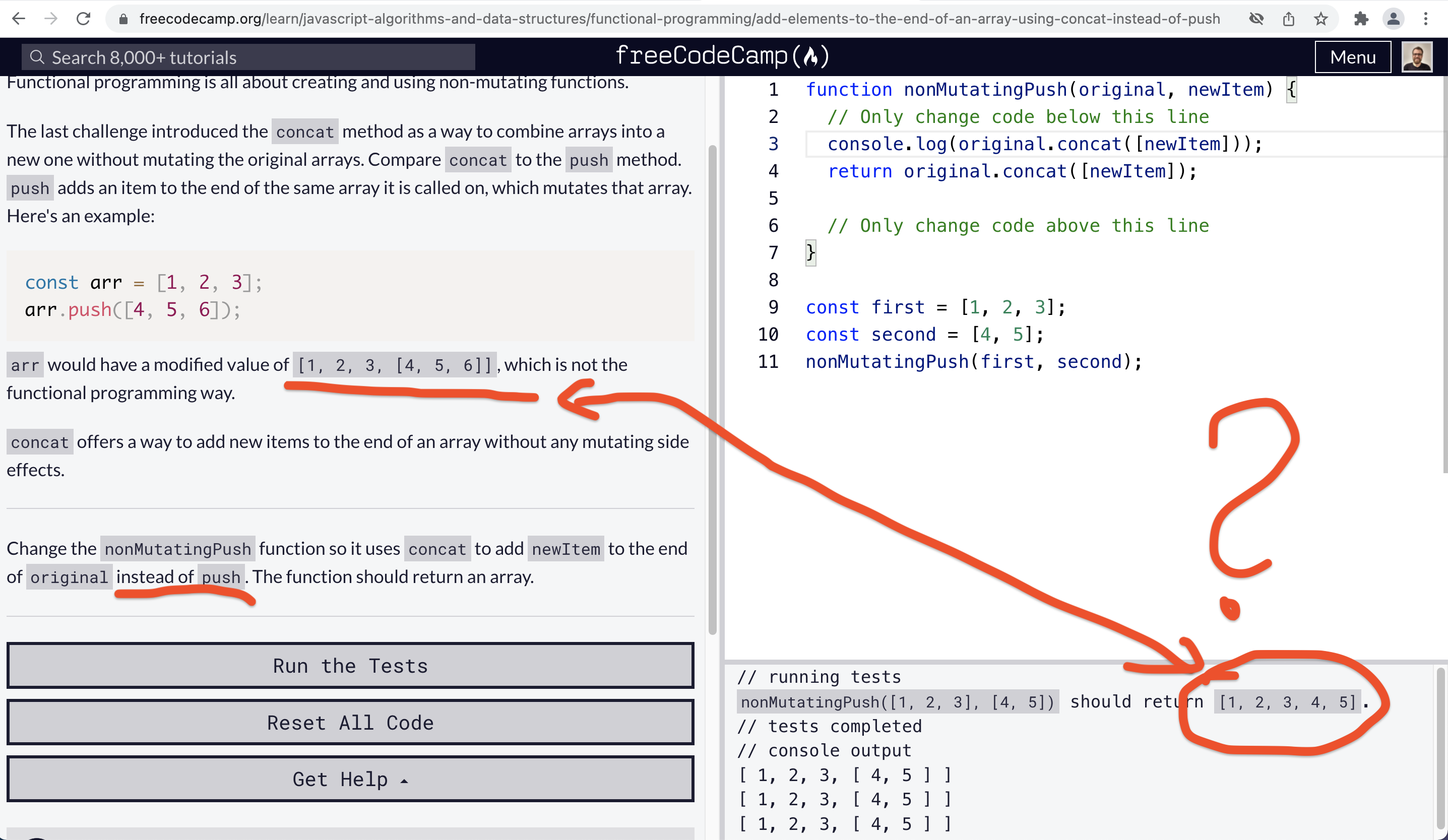
System
- Device: [laptop]
- OS: [macOS]
- Browser: [chrome]
Additional context
Issue Analytics
- State:
- Created 2 years ago
- Reactions:1
- Comments:21 (13 by maintainers)
Top Results From Across the Web
Add Elements to the End of an Array Using concat ... - YouTube
In this functional programming tutorial we add elements to the end of an array using concat instead of push. This video constitutes one...
Read more >Add Elements to the End of an Array Using concat Instead of ...
Problem Explanation. Where the push method adds new element to the end of the orginal array, the concat method creates a new array...
Read more >Javascript Array.push is 945x faster than Array.concat - DEV ...
Apparently the answer has a lot to do with the fact that .concat creates a new array while .push modifies the first array....
Read more >How to extend an existing JavaScript array with another array ...
Push expects a list of items to add to the array. The apply() method, however, takes the expected arguments for the function call...
Read more >Array methods - The Modern JavaScript Tutorial
We already know methods that add and remove items from the beginning or the end: arr.push(...items) – adds items to the end, ...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
If we want users to learn a “non mutating push”, I think its better that the solution not provide an avenue to mutate the original arrays.
I think the current intended solution is fine but the wording describing it isn’t quite accurate.
@jeremylt The challenge doesn’t say anything about using a spread operator, instead it asks users to “Change the nonMutatingPush function so it uses concat to add newItem to the end of original instead of push”. Pushing an array to an array would produce an array in an array. Since “The concat() method is used to merge two or more arrays” (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/concat) to replicate the effect of push one needs to put the array about to be “pushed” into a new array, so the outer array gets merged and the inner array stays intact. My suggestion would be, if replicating the push behaviour isn’t the case, but merging the two given arrays, the instructions should ask users to “Change the nonMutatingPush function so it uses concat to merge newItem with original, without altering
const first
andconst second
”