Popular Animation Library (GSAP) doesn't work after site is built
See original GitHub issueSummary
Using GSAP on my gatsby site works fine during development, but does not work in the built version. I’m guessing this has something to do with me using it only in componentDidMount, and therefore being ignored from the server rendering or something of that sort. Either way, I’m not sure how I’d work around this. Any ideas would be greatly appreciated!
Relevant information
Using the latest GSAP, and the latest gatsby (v2). Using gatsby-starter-blog Win10
Environment (if relevant)
Ran gatsby info --clipboard but it did not work, despite being on the latest gatsby-cli
File contents (if changed)
gatsby-config.js
:
module.exports = {
siteMetadata: {
title: 'Gatsby Starter Blog',
author: 'Kyle Mathews',
description: 'A starter blog demonstrating what Gatsby can do.',
siteUrl: 'https://gatsbyjs.github.io/gatsby-starter-blog/',
},
pathPrefix: '/gatsby-starter-blog',
plugins: [
{
resolve: `gatsby-source-filesystem`,
options: {
path: `${__dirname}/src/pages`,
name: 'pages',
},
},
{
resolve: `gatsby-plugin-emotion`,
options: {
// Accepts all options defined by `babel-plugin-emotion` plugin.
},
},
{
resolve: `gatsby-transformer-remark`,
options: {
plugins: [
{
resolve: `gatsby-remark-images`,
options: {
maxWidth: 590,
},
},
{
resolve: `gatsby-remark-responsive-iframe`,
options: {
wrapperStyle: `margin-bottom: 1.0725rem`,
},
},
'gatsby-remark-prismjs',
'gatsby-remark-copy-linked-files',
'gatsby-remark-smartypants',
],
},
},
`gatsby-transformer-sharp`,
`gatsby-plugin-sharp`,
{
resolve: `gatsby-plugin-google-analytics`,
options: {
//trackingId: `ADD YOUR TRACKING ID HERE`,
},
},
`gatsby-plugin-feed`,
{
resolve: `gatsby-plugin-manifest`,
options: {
name: `Gatsby Starter Blog`,
short_name: `GatsbyJS`,
start_url: `/`,
background_color: `#ffffff`,
theme_color: `#663399`,
display: `minimal-ui`,
icon: `src/assets/gatsby-icon.png`,
},
},
`gatsby-plugin-offline`,
`gatsby-plugin-react-helmet`,
{
resolve: 'gatsby-plugin-typography',
options: {
pathToConfigModule: 'src/utils/typography',
},
},
],
}
package.json
:
{
"name": "gatsby-starter-blog",
"description": "Starter Gatsby Blog",
"version": "1.0.0",
"author": "Kyle Mathews <mathews.kyle@gmail.com>",
"bugs": {
"url": "https://github.com/gatsbyjs/gatsby-starter-blog/issues"
},
"dependencies": {
"emotion": "^9.2.12",
"emotion-server": "^9.2.12",
"gatsby": "^2.0.0",
"gatsby-plugin-emotion": "^2.0.5",
"gatsby-plugin-feed": "^2.0.5",
"gatsby-plugin-google-analytics": "^2.0.5",
"gatsby-plugin-manifest": "^2.0.2",
"gatsby-plugin-offline": "^2.0.5",
"gatsby-plugin-react-helmet": "^3.0.0",
"gatsby-plugin-sharp": "^2.0.5",
"gatsby-plugin-typography": "^2.2.0",
"gatsby-remark-copy-linked-files": "^2.0.5",
"gatsby-remark-images": "^2.0.1",
"gatsby-remark-prismjs": "^3.0.0",
"gatsby-remark-responsive-iframe": "^2.0.5",
"gatsby-remark-smartypants": "^2.0.5",
"gatsby-source-filesystem": "^2.0.1",
"gatsby-transformer-remark": "^2.1.1",
"gatsby-transformer-sharp": "^2.1.1",
"gsap": "^2.0.2",
"lodash": "^4.17.11",
"lodash-es": "^4.17.11",
"prismjs": "^1.15.0",
"react": "^16.5.1",
"react-dom": "^16.5.1",
"react-emotion": "^9.2.12",
"react-helmet": "^5.2.0",
"react-typography": "^0.16.13",
"typeface-merriweather": "0.0.43",
"typeface-montserrat": "0.0.43",
"typography": "^0.16.17",
"typography-theme-wordpress-2016": "^0.15.10"
},
"devDependencies": {
"eslint": "^4.19.1",
"eslint-plugin-react": "^7.11.1",
"gh-pages": "^1.2.0",
"prettier": "^1.14.2"
},
"homepage": "https://github.com/gatsbyjs/gatsby-starter-blog#readme",
"keywords": [
"gatsby"
],
"license": "MIT",
"main": "n/a",
"repository": {
"type": "git",
"url": "git+https://github.com/gatsbyjs/gatsby-starter-blog.git"
},
"scripts": {
"dev": "gatsby develop",
"lint": "./node_modules/.bin/eslint --ext .js,.jsx --ignore-pattern public .",
"test": "echo \"Error: no test specified\" && exit 1",
"format": "prettier --trailing-comma es5 --no-semi --single-quote --write 'src/**/*.js' 'src/**/*.md'",
"develop": "gatsby develop",
"build": "gatsby build",
"deploy": "gatsby build --prefix-paths && gh-pages -d public",
"fix-semi": "eslint --quiet --ignore-pattern node_modules --ignore-pattern public --parser babel-eslint --no-eslintrc --rule '{\"semi\": [2, \"never\"], \"no-extra-semi\": [2]}' --fix gatsby-node.js"
}
}
gatsby-node.js
:
const _ = require('lodash')
const Promise = require('bluebird')
const path = require('path')
const { createFilePath } = require('gatsby-source-filesystem')
exports.createPages = ({ graphql, actions }) => {
const { createPage } = actions
return new Promise((resolve, reject) => {
const blogPost = path.resolve('./src/templates/blog-post.js')
resolve(
graphql(
`
{
allMarkdownRemark(sort: { fields: [frontmatter___date], order: DESC }, limit: 1000) {
edges {
node {
fields {
slug
}
frontmatter {
title
}
}
}
}
}
`
).then(result => {
if (result.errors) {
console.log(result.errors)
reject(result.errors)
}
// Create blog posts pages.
const posts = result.data.allMarkdownRemark.edges;
_.each(posts, (post, index) => {
const previous = index === posts.length - 1 ? null : posts[index + 1].node;
const next = index === 0 ? null : posts[index - 1].node;
createPage({
path: post.node.fields.slug,
component: blogPost,
context: {
slug: post.node.fields.slug,
previous,
next,
},
})
})
})
)
})
}
exports.onCreateNode = ({ node, actions, getNode }) => {
const { createNodeField } = actions
if (node.internal.type === `MarkdownRemark`) {
const value = createFilePath({ node, getNode })
createNodeField({
name: `slug`,
node,
value,
})
}
}
gatsby-browser.js
: N/A
gatsby-ssr.js
: N/A
Issue Analytics
- State:
- Created 5 years ago
- Comments:19 (10 by maintainers)
Top Results From Across the Web
Animation doesn't work on refresh - GSAP - GreenSock
However I'm hitting a snag, every time I refresh the page, all animations stop working, it's like that on my IIS/Flask webserver and......
Read more >GSAP animation does not work in FF and Safari. - GreenSock
I am in the process of creating a website. On the page there are some animations. In Chrome everything works great but in...
Read more >Animation doesn't seem to work - GSAP - GreenSock
Hi All, I've recently seen a javascript animation created on codepen: See the Pen bkLwt by GreenSock (@GreenSock) on CodePen Problem is it ......
Read more >How to make animations and image load after the page is ...
Hi,. I created a project using GSAP TimelineMax Plugin where the text on the left side slides in the original position from the...
Read more >Animation do not trigger on first render - GSAP - GreenSock
Hi, I am currently trying to animate text with scroll trigger + gsap scroll smoother on a Gabtsy(React) website. My problem, is that...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
OK, figured out what was wrong. Turns out I had to import the GSAP libraries from “gsap” rather than “gsap/all”, even though that is the path they suggest in the docs. Go figure.
Thanks for the offer to help @endymion1818. Unfortunately I wasn’t fully able to figure out how to post on Spectrum. I’ll have to learn that one of these days 😃
@TylerBarnes @endymion1818 I’m having the same Issue. Everything works fine in development but when pushed to production the animations are not rendered and do not throw any console errors. I tried the above solutions but still no luck. I uninstalled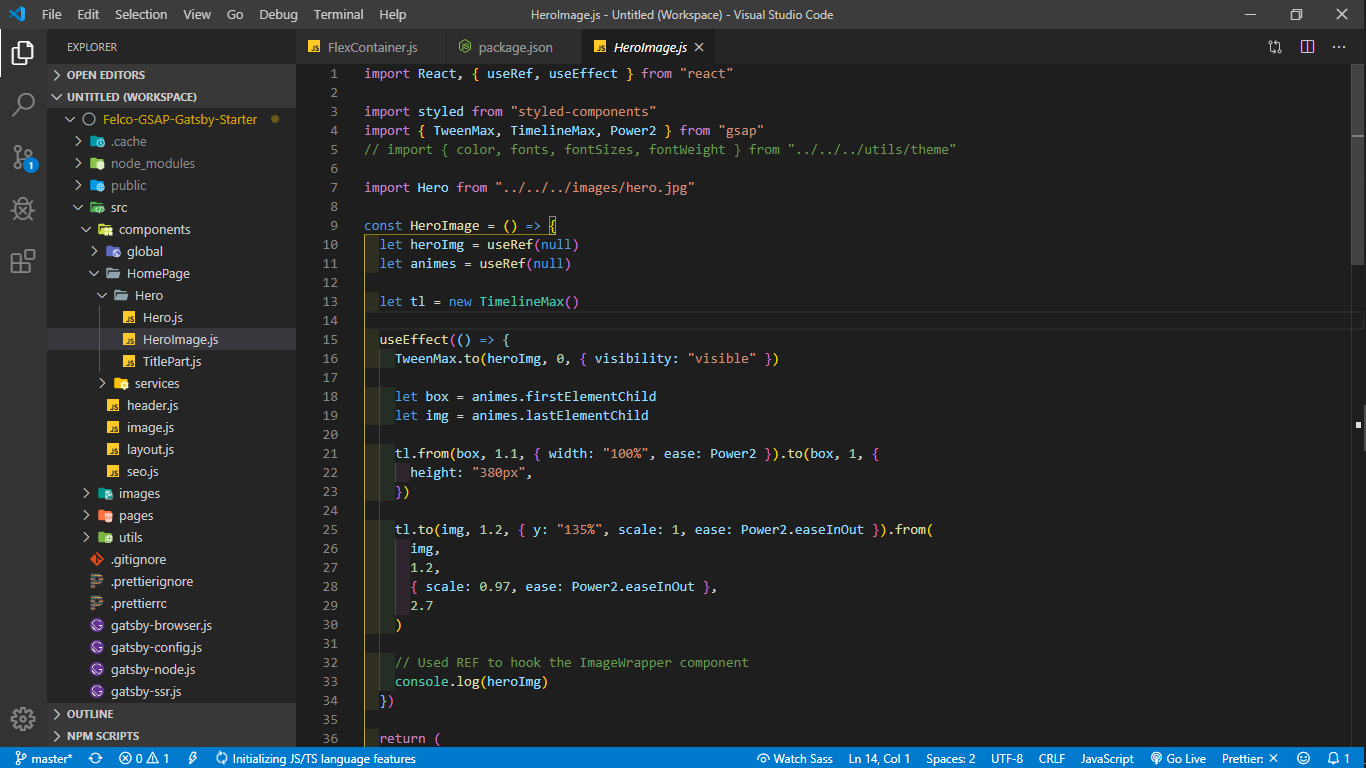
gatsby-plugin-offline
but couldn’t find the extension mentioned above. Here is my code.