Failed to export a file using drive API
See original GitHub issueI am using:
- googleapis@12.3.0
- node@4.3.2
I was trying to download a spreadsheet using Drive API v3 like this:
drive.files.export({
fileId: <some-valid-file-id>
mimeType: 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet'
}, (err, buf) => {
if (err) {
console.log('Error:', err);
return;
}
console.log('Received %d bytes', buf.length);
fs.writeFileSync('./out.xlsx', buf);
});
Resulting file was corrupted. Same situation with ‘application/pdf’ - I could not open them.
After some investigation, I noticed that node-request module downloads data as string (decoded as utf8) by default unless you specify encoding option set to null
. (what I am downloading here are binary files!)
// cited from - https://github.com/request/request
encoding - Encoding to be used on setEncoding of response data. If null, the body is returned as a Buffer. Anything else (including the default value of undefined) will be passed as the encoding parameter to toString() (meaning this is effectively utf8 by default). (Note: if you expect binary data, you should set encoding: null.)
By adding a line in drive.files.export() function like below, I was able to download the file successfully:
// ./apis/drive/v3.js
export: function (params, callback) {
var parameters = {
options: {
url: 'https://www.googleapis.com/drive/v3/files/{fileId}/export',
method: 'GET',
encoding: null // <===== added line
},
params: params,
requiredParams: ['fileId', 'mimeType'],
pathParams: ['fileId'],
context: self
};
I am aware this file is generated with swig, and probably other methods (like drive.files.get with “alt” set to “media” for binary data) are suffering from the same problem, I do not have sufficient knowledge to propose most effective solution to this. I hope some one could come up with a good idea to this problem.
Issue Analytics
- State:
- Created 7 years ago
- Comments:19 (9 by maintainers)
@enobufs I’m thinking #623 ought to be able to solve this for you (allow you to pass
encoding: null
).Hi , I am having the same problem I am using: googleapis@110.0.0 node@16.13.0 I have tested with and whithout the callback. and sending the “encoding:null” inside parameters , and in Options. When the service response , it responses an Object, and the data seems to be in the “data” field. But if I try to save it, the resulted file is always corrupted.
Here is what Im trying: ` let jsonDownlaodFile = { fileId: fileId, mimeType: ‘application/vnd.openxmlformats-officedocument.spreadsheetml.sheet’, encoding: null // <= If I debug inside v3.js , this seems to work. }
`
This is what it looks inside the buffer.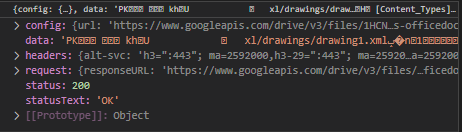
And this is the file result as an xlsx.
What am I missing ? I hope somebody could help me with this. Thank you so much in advance.