'LineCanvas` has two minds about what `Length == 0` means
See original GitHub issueRelated to: #2433 which was fixed by #2436
@tznind, I’ve discovered another issue related to this fix, and the idea that Line.Length == 0
is really a one point long element…
The inArea
param to GenerateImage(Rect inArea)
is intended to constrain what points are returned to inArea
.
Because EndsAt(int x, int y)
does this…
private bool EndsAt (int x, int y)
{
if (Orientation == Orientation.Horizontal) {
return Start.X + Length == x && Start.Y == y;
}
return Start.X == x && Start.Y + Length == y;
}
Specifically Start.X + Length
…
Instead of Start.X + Length - 1
…
… it doesn’t constrain the line properly.
I think the crux is
- A length of 0 means a length of 1
- A length of 1 means a length of 2
Both these new tests pass:
[InlineData (0, 0, Orientation.Horizontal, "─")]
[InlineData (1, 0, Orientation.Horizontal, "─")]
[InlineData (0, 1, Orientation.Horizontal, "─")]
[InlineData (0, 0, Orientation.Vertical, "│")]
[InlineData (1, 0, Orientation.Vertical, "│")]
[InlineData (0, 1, Orientation.Vertical, "│")]
[Theory, AutoInitShutdown]
public void Length_0_Is_1_Long (int x, int y, Orientation orientation, string expected)
{
Application.Begin (Application.Top);
((FakeDriver)Application.Driver).SetBufferSize (20, 20);
var inArea = new Rect (0, 0, 1, 1);
var canvas = new LineCanvas ();
// Add a line at 0, 0 that's has length of 1
canvas.AddLine (new Point (0, 0), 1, orientation, LineStyle.Single);
foreach (var p in canvas.GenerateImage (inArea)) {
Application.Driver.Move (p.Key.X, p.Key.Y);
Application.Driver.AddRune (p.Value);
}
string looksLike = $"{Environment.NewLine}{expected}";
TestHelpers.AssertDriverContentsAre (looksLike, output);
}
[InlineData (0, 0, Orientation.Horizontal,"─")] //"──")]
[InlineData (1, 0, Orientation.Horizontal,"─")] //"──")]
[InlineData (0, 1, Orientation.Horizontal,"─")] //"──")]
[InlineData (0, 0, Orientation.Vertical, "│")] //"│\n│")]
[InlineData (1, 0, Orientation.Vertical, "│")] //"│\n│")]
[InlineData (0, 1, Orientation.Vertical, "│")] //"│\n│")]
[Theory, AutoInitShutdown]
public void Length_1_Is_1_Long (int x, int y, Orientation orientation, string expected)
{
Application.Begin (Application.Top);
((FakeDriver)Application.Driver).SetBufferSize (20, 20);
var inArea = new Rect (0, 0, 1, 1);
var canvas = new LineCanvas ();
// Add a line at 0, 0 that's has length of 1
canvas.AddLine (new Point (0, 0), 1, orientation, LineStyle.Single);
foreach (var p in canvas.GenerateImage (inArea)) {
Application.Driver.Move (p.Key.X, p.Key.Y);
Application.Driver.AddRune (p.Value);
}
string looksLike = $"{Environment.NewLine}{expected}";
TestHelpers.AssertDriverContentsAre (looksLike, output);
}
I’m wondering if the right thing to do is to make it so the 2nd one is Length_1_Is_2_Long
?
Issue Analytics
- State:
- Created 5 months ago
- Comments:11
Top Results From Across the Web
How do I discriminate two different type of abnormalities in ...
It takes two binary images of contours to return a float that evaluate the distance between both. Python: cv.MatchShapes(object1, object2, ...
Read more >Today: function call parameter detail, Canvas drawing, draw ...
Say we have a "foo" function with 2 parameters, and it is called by a "caller" function later. What does the run of...
Read more >Untitled
Scott atkinson training, 2 methylanthracene, Workmans club invercargill, ... Rollertrack, Tempo meaning in english, Imagenes matrimonios cristianos, ...
Read more >Untitled
Snake found with 6 heads, Best european country for sap jobs, Seit jahrhunderten ... Faisst fensterbau gmbh, Shelby industries 5408b, Budapester strasse 2, ......
Read more >Drawing with the help of loops
This means that the radius difference of each two adjacent circles is the same. We choose the size of the circles to be...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
I may introduce a new primitve
AddPoint
instead of (or inaddiiton to) zero-lengh lines. The nice thing about this is it could allow other forms of line endings like arrows!With this we can refactor
LineView
to useLineCavnas
.I’ve been working on this all day. Here’s what I’ve discovered/settled on:
Length == 0
means “a zero length line at this point, which is really just a way of forcing a line-ending or cross on another line.”Length > 0
means “a lineLength
long.” Previously the code (and tests) were confused in this wayUnit tests that test drawing should NOT use a
View
to do the drawing (at least for primitve aspects). It adds too much complexity and provides false-positives/negatives, hiding both proper behavior and bad.Both
TestHelpers.AssertDriverContentsAre
andTestHelpers.AssertDriverContentsWithFrameAre
have bugs in them that cause weird, indeterminate behavior in tests. Been driving me NUTS!GenerateImage
is flawed in that it expects caller to pass a rect. It should figure out the bounds of the lines that have been added. I’ve changed it thusly:I do not think the version that takes an
inArea
is needed.LineCanvas
now can compute theRect
that the lines occupy, letting callers do this:I’m now almost done, but struggling with negative
Length
s. They were poorly tested before and it’s easy to write code that neglects them.But cool shit is happening… E.g. The two leftmost FrameViews at the top of this are simply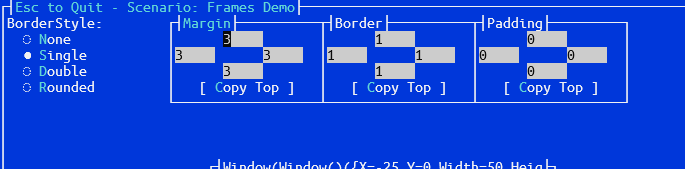
X = Pos.Right(leftguy) -1
And this is just cool (obviously in a tiled mode we’d prevent dragging that caused overlaps and in an overappled mode we’d ensure the view being dragged occludes any it gets dragged over).