org.http4s.client.middleware.Retry consumes memory proportional to number of retries performed
See original GitHub issuePlease consider this simple example:
name := "http4s-retry-leak"
version := "0.1"
scalaVersion := "2.13.2"
libraryDependencies += "org.http4s" %% "http4s-blaze-client" % "0.21.4"
import cats.effect.{ExitCode, IO, IOApp}
import cats.syntax.option._
import javax.net.ssl.SSLContext
import org.http4s.client.blaze.BlazeClientBuilder
import org.http4s.client.middleware.{Retry, RetryPolicy}
import org.http4s.{Method, Request, Uri}
import scala.concurrent.ExecutionContext.global
import scala.concurrent.duration._
object App extends IOApp {
override def run(args: List[String]): IO[ExitCode] =
BlazeClientBuilder[IO](global, SSLContext.getDefault.some).resource
.use { client =>
val retryPolicy: RetryPolicy[IO] = (_, _, _) => 5.millis.some
val clientWithRetries = Retry[IO](retryPolicy)(client)
val request =
Request[IO](Method.GET,
uri = Uri.fromString("http://unknown-host").toOption.get)
clientWithRetries.stream(request).compile.drain
}
.as(ExitCode.Success)
}
Each time the client makes an attempt, a series of new objects get allocated and retained. Here’s how it looks like after ~148k attempts:
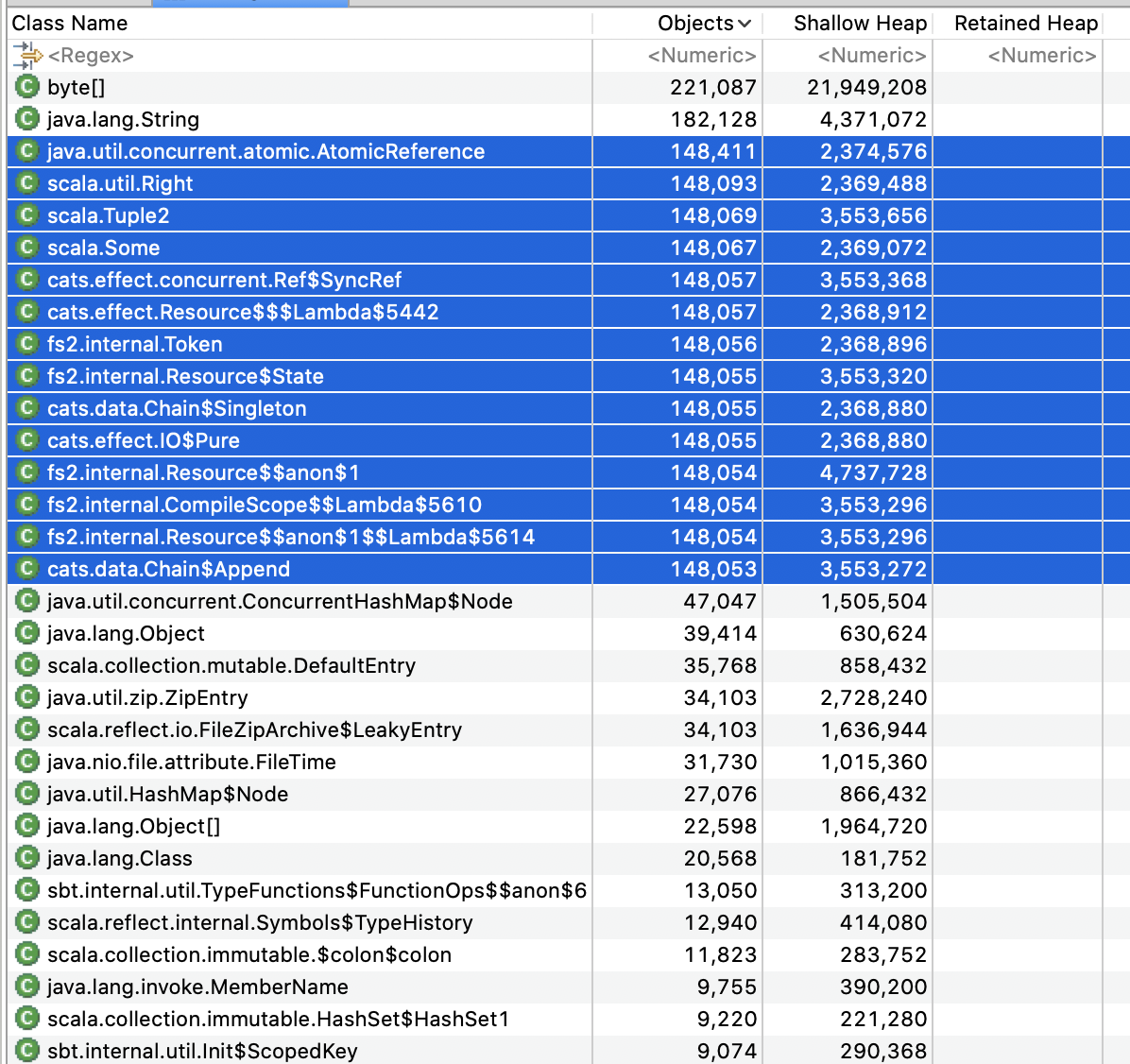
And here you can find the heap dump itself: https://www.dropbox.com/t/GkFbbEMDge8uJdba
From what I understand, the issue might as well be in fs2, not in http4s, but I haven’t yet found a way to minimize the example further.
Issue Analytics
- State:
- Created 3 years ago
- Comments:9 (6 by maintainers)
Top Results From Across the Web
object Retry - http4s
Provides extension methods for using the a http4s org.http4s.client.Client import scalaz.concurrent.Task import org.http4s._ import org.http4s.client.
Read more >Changelog - http4s
A new internal websocket client is published for implementation across repos. We expect to release this as a public API in the next...
Read more >HTTP Client - http4s
Http4s includes some middleware Out of the Box in the org.http4s.client.middleware package. These include: Following of redirect responses (Follow Redirect) ...
Read more >HTTP Client - http4s
Let's create a client with http4s to try our service. ... _ import org.http4s.server.middleware. ... There are a number of ways to construct...
Read more >HTTP Client - http4s
The Client trait in http4s can submit a Request to a server and return a Response . ... EmberServerBuilder import org.http4s.server.middleware.
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
A sawtooth shape in the heap graph is very normal, it usually indicates that garbage collection ran and removed objects from the heap. If anything, that indicates that it’s maybe not a leak (where references are held and cannot be garbage collected), but rather memory pressure (lots of garbage being created and then cleaned)
I wonder whether this is fixed in 1.0, with its hotswap.