filtered scenes are squeezed by resizable windows
See original GitHub issueI’ve been converting apps to use resizable windows. Mostly this just involves invoking AppSettings.setResizable()
and adjusting the GUI layout each time the window gets resized.
I’ve encountered an issue involving filters in resizable windows, and I believe it’s a bug in the Engine. When I resize a window to a new aspect ratio, the rendered scene gets squashed to “fit” the new aspect ratio. This only happens when there are filters. In an unfiltered scene, no squashing occurs.
Here’s a simple testcase based on TestCartoonEdge
:
package jme3test.post;
import com.jme3.app.SimpleApplication;
import com.jme3.light.DirectionalLight;
import com.jme3.material.Material;
import com.jme3.math.ColorRGBA;
import com.jme3.math.FastMath;
import com.jme3.math.Quaternion;
import com.jme3.math.Vector3f;
import com.jme3.post.FilterPostProcessor;
import com.jme3.post.filters.CartoonEdgeFilter;
import com.jme3.scene.Geometry;
import com.jme3.scene.Node;
import com.jme3.scene.Spatial;
import com.jme3.scene.Spatial.CullHint;
import com.jme3.system.AppSettings;
import com.jme3.texture.Texture;
public class TestIssueXXXX extends SimpleApplication {
private FilterPostProcessor fpp;
public static void main(String[] args) {
AppSettings s = new AppSettings(true);
s.setResizable(true);
TestIssueXXXX app = new TestIssueXXXX();
app.setSettings(s);
app.start();
}
private void setupFilters() {
fpp = new FilterPostProcessor(assetManager);
int numSamples = getContext().getSettings().getSamples();
if (numSamples > 0) {
fpp.setNumSamples(numSamples);
}
CartoonEdgeFilter toon = new CartoonEdgeFilter();
toon.setEdgeColor(ColorRGBA.Yellow);
fpp.addFilter(toon);
viewPort.addProcessor(fpp);
}
private void makeToonish(Spatial spatial) {
if (spatial instanceof Node) {
Node n = (Node) spatial;
for (Spatial child : n.getChildren()) {
makeToonish(child);
}
} else if (spatial instanceof Geometry) {
Geometry g = (Geometry) spatial;
Material m = g.getMaterial();
if (m.getMaterialDef().getMaterialParam("UseMaterialColors") != null) {
Texture t = assetManager.loadTexture("Textures/ColorRamp/toon.png");
m.setTexture("ColorRamp", t);
m.setBoolean("UseMaterialColors", true);
m.setColor("Specular", ColorRGBA.Black);
m.setColor("Diffuse", ColorRGBA.White);
m.setBoolean("VertexLighting", true);
}
}
}
private void setupLighting() {
DirectionalLight dl = new DirectionalLight();
dl.setDirection(new Vector3f(-1, -1, 1).normalizeLocal());
dl.setColor(new ColorRGBA(2, 2, 2, 1));
rootNode.addLight(dl);
}
private void setupModel() {
Spatial model = assetManager.loadModel("Models/MonkeyHead/MonkeyHead.mesh.xml");
makeToonish(model);
rootNode.attachChild(model);
}
@Override
public void simpleInitApp() {
viewPort.setBackgroundColor(ColorRGBA.Gray);
flyCam.setDragToRotate(true);
setupLighting();
setupModel();
setupFilters();
}
}
Issue Analytics
- State:
- Created a year ago
- Reactions:1
- Comments:7 (7 by maintainers)
Top Results From Across the Web
Allow user to resize an undecorated Stage - Stack Overflow
Scene ; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; /** * Util class to handle window resizing when a stage style set to StageStyle.
Read more >issue stop resizing cell and slicer - Microsoft Tech Community
I did a dashboard but each time that a click a button in the slicer the row resizing the filters too. I did...
Read more >Resizing Outputs
You can resize any individual output. To do this, left-click the appropriate output and move your scroll wheel up or down until you...
Read more >FFmpeg Filters Documentation
This sets size of window which will be processed at once. ... This filter is also able to stretch/squeeze the audio data to...
Read more >Keyboard Control - mpv.io
Alt+0 (and command+0 on macOS): Resize video window to half its original size. ... the actual filter function will be squeezed into the...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Yes. All the way back to 3.1.0-stable .
Here’s an example of a squashed scene: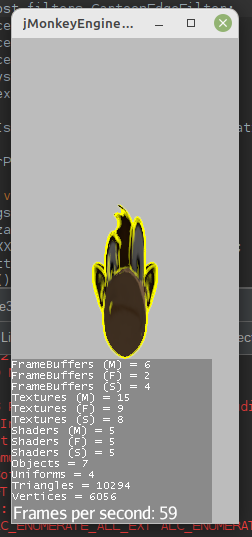