Date range validation
See original GitHub issueI’m really excited about this library, however I found an issue that I’m not sure how to deal with. I’d like to be able to validate a date range, but it doesn’t seem to work. I’ve written two tests to show what does and does not work for me.
test('validate a number range', () => {
return yup
.object({
start: yup.number().required(),
end: yup.number().when('start', (st, schema) => {
return schema.min(st);
}),
})
.validate({
start: 5,
end: 3,
})
.catch((e) => {
expect(e.message).toEqual(
'end must be greater than or equal to 5', // works :)
);
});
});
test('validates a date range', () => {
return yup
.object({
start: yup.date().required(),
end: yup.date().when('start', (st, schema) => {
return schema.min(st);
}),
})
.validate({
start: moment().toDate(),
end: moment()
.subtract(1, 'days')
.toDate(),
})
.catch((e) => {
expect(e).toEqual(1); // this line is never executed
});
});
The first test works, but I get an error message RangeError: Maximum call stack size exceeded
on the second test. Is there something I can do differently to validate a date range?
Issue Analytics
- State:
- Created 5 years ago
- Reactions:5
- Comments:11 (2 by maintainers)
Top Results From Across the Web
Validating date range - FormValidation
The following form asks you to enter a date in the range of 2000/01/01 and 2020/12/30. It can be implemented by using the...
Read more >Javascript - date range validation - Stack Overflow
i've a form user can enter any date , but i want to set a date range validation . for example: from 1-12-2012...
Read more >Use Excel Data Validation for Entering Dates - Contextures
On any sheet in the workbook, type "StartDate" in one cell, and type "EndDate" in the cell below. In the cells to the...
Read more >Javascript Data Type How to - Check date range validation
We would like to know how to check date range validation. Answer. <!DOCTYPE html> <html> ...
Read more >Validate Date Range (From - To). · GitHub
Validate Date Range (From - To). Raw. date-range-validation.js ...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Unlike the documentation, I got this working by using
yup
instead ofschema
.Here are two solutions that worked for me.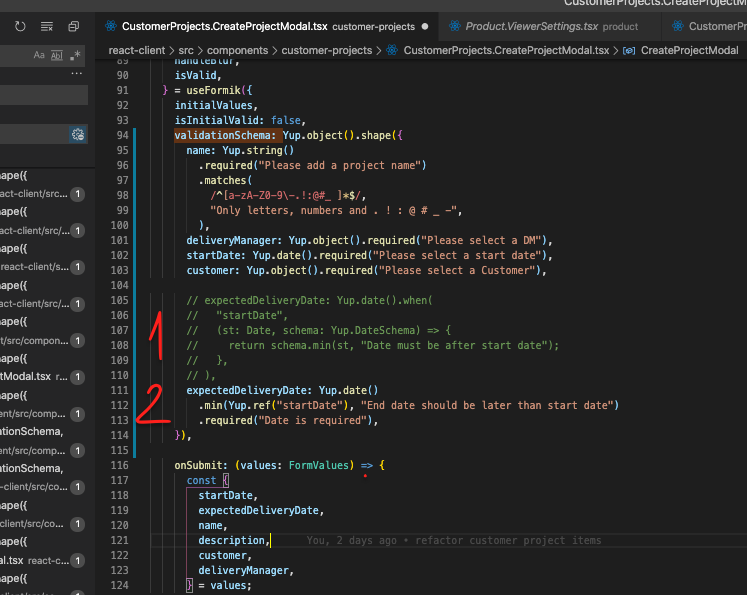