Blury on rotated image.
See original GitHub issueHere is a comparison between PIL rotation and kornia rotation. It is pretty clear that the image was blurred.
import torch
import kornia.augmentation.functional as F
from torchvision.transforms import functional as tvF
from torchvision.transforms import transforms
import matplotlib.pyplot as plt
import numpy as np
to_tensor = transforms.ToTensor()
to_pil = transforms.ToPILImage()
def tensor_pre_transform_wrapper(input: torch.Tensor):
""" A wrapper that tried to reproduce the actual output from:
- transforms.ToPILImage()
- transforms.ToTensor()
For each image, simply (img * 255).int() // 255
"""
return (input * 255).int().float() / 255
in_tensor = torch.rand((3, 23, 44))
in_pil = to_pil(in_tensor)
degrees = 10
out_tensor = F._apply_rotation(
tensor_pre_transform_wrapper(in_tensor), {'degrees': torch.tensor(degrees)}, False)
out_pil = tvF.rotate(in_pil, angle=degrees)
plt.imshow(np.moveaxis(out_tensor.numpy(), 0, -1))
plt.imshow(np.array(out_pil))
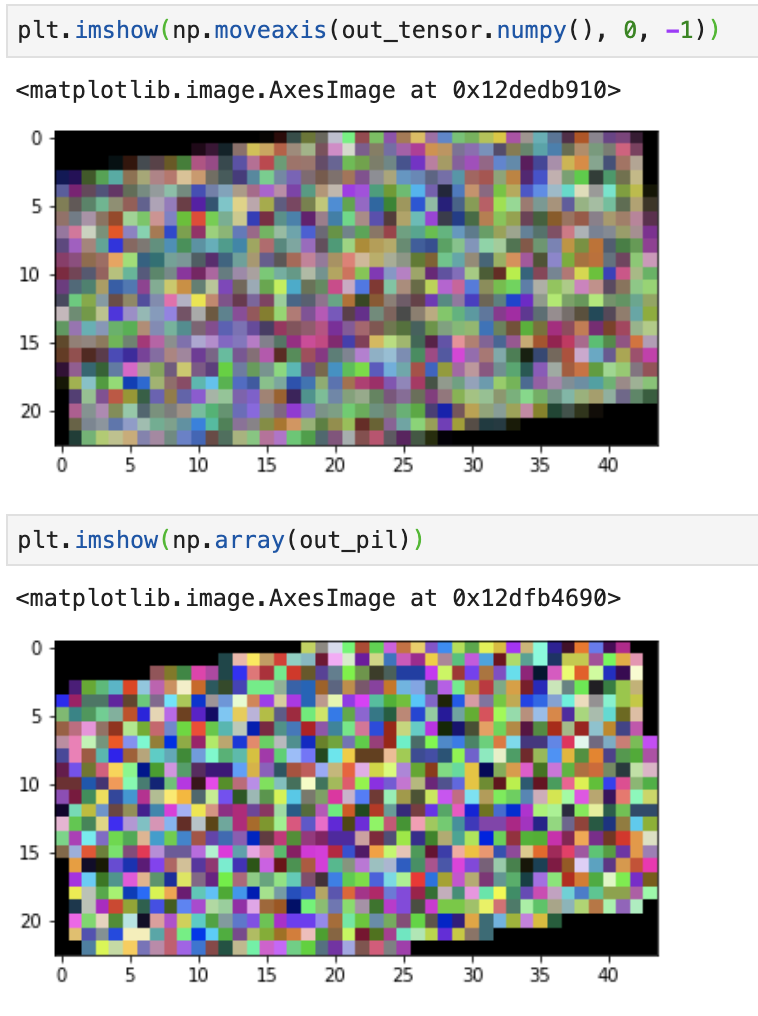
Issue Analytics
- State:
- Created 4 years ago
- Comments:6 (2 by maintainers)
Top Results From Across the Web
Rotating my drawings make them pixelated and blurry
Solved: When i ctrl + t and rotate or move something it comes out pixelally and gross. It didnt do this before but...
Read more >Image is getting blurry after rotating it. : r/GIMP - Reddit
Hi, Im trying to rotate an image, but after rotating it becomes blurry like in the picture below. The picture i try to...
Read more >Rotation makes the image blurred (#2280) - GIMP - GitLab
When I try to rotate an image, it gets blurred. And the more I apply a rotation, the more it gets blurred. I...
Read more >CSS Transforms - Why does a simple rotation make the image ...
Looking to rotate an image 90deg, but the resulting image is unacceptably blurry. transform: rotate(90deg); This is the same in both Firefox and...
Read more >Image blurred after rotation. - Procreate Folio
I noticed the quality of an image is reduced whenever it is rotated, be it it's the original sketches or after duplication of...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Let’s add that flag
@doube1008 to rotate image use
kornia.geometry.transform.rotate