color.rgb_to_hsv can't be converted to simplified onnx model
See original GitHub issueDescribe the bug
I’ve created a simple NN that simply convert RGB to HSV using the color.rgb_to_hsv() function. When I try to convert the model to Onnx and then simplify it (or convert it to blob format using this converter: http://blobconverter.luxonis.com) I get this error message: This is an invalid model. Type Error: Type ‘tensor(bool)’ of input parameter (3) of operator (CumSum) in node (CumSum_4) is invalid.
Reproduction steps
Use this code to reproduced the error:
import torch
from torch import nn
import kornia
import onnx
from onnxsim import simplify
class rgb2hsv(nn.Module):
def forward(self, image):
hsv_tensor = kornia.color.rgb_to_hsv(image)
return hsv_tensor
X = torch.ones((1, 3, 300, 300), dtype=torch.float32)
torch.onnx.export(
rgb2hsv(),
(X), # Dummy input for shape
"model.onnx",
opset_version=12,
do_constant_folding=True,
)
onnx_model = onnx.load("model.onnx")
model_simpified, check = simplify(onnx_model)
onnx.save(model_simpified, "model_simplified.onnx
### Expected behavior
Expecting the model to convert correctly
### Environment
```shell
- PyTorch Version (e.g., 1.0): 1.9.1
- OS (e.g., Linux): MacOS
- How you installed PyTorch (`conda`, `pip`, source): Pip
- Build command you used (if compiling from source): NA
- Python version: 3.8
- CUDA/cuDNN version: NA
- GPU models and configuration: NA
- Any other relevant information: NA
Additional context
No response
Issue Analytics
- State:
- Created 2 years ago
- Comments:13 (5 by maintainers)
Top Results From Across the Web
Converting Pytorch model .pth into onnx model - Stack Overflow
I want to convert that into Tensorflow protobuf. But I am not finding any way to do that. I have seen onnx can...
Read more >Module: tf.compat.v1 | TensorFlow v2.11.0
autograph module: Conversion of eager-style Python into TensorFlow graph code. ... estimator module: Estimator: High level tools for working with models.
Read more >Unable to convert ONNX model to TensorRT
Hi, I am trying to convert EfficientDet model from this Repo, which is implemented in Pytorch, to TensorRT to deploy on edge devices...
Read more >How to convert cv::Mat to pcl::pointcloud with color-Opencv
I tried simple example, following your paradigm, and it works perfectly (PCL 1.8 built from sources, OpenCV 3.1 built from sources, g++ 5.x...
Read more >Digital Signal Processing - SAS Help Center
Color space conversion ( COLORSPACE ) translates the ... The notation follows the model described for PROC SSM, with the matrices wt.
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
My workaround for now. There are causes for errors in both kornia and onnx-simplifier. Thus, it removes the error sources for both kornia and onnx-simplifier.
kornia
onnx-simplifier
Gather
). The last two lines of logic are essentially unnecessary, but I left them in to illustrate that there is an option to disable the optimization logic of onnx-simplifier. model.onnx and model_non-opt.onnx will generate the exact same file.Wikipedia https://en.wikipedia.org/wiki/HSL_and_HSV
Note that I have not firmly verified that the RGB to HSV conversion custom logic works correctly. This procedure does not dare to optimize ONNX and will produce a very redundant model. Therefore, if you do not like it, please ignore it.
ONNX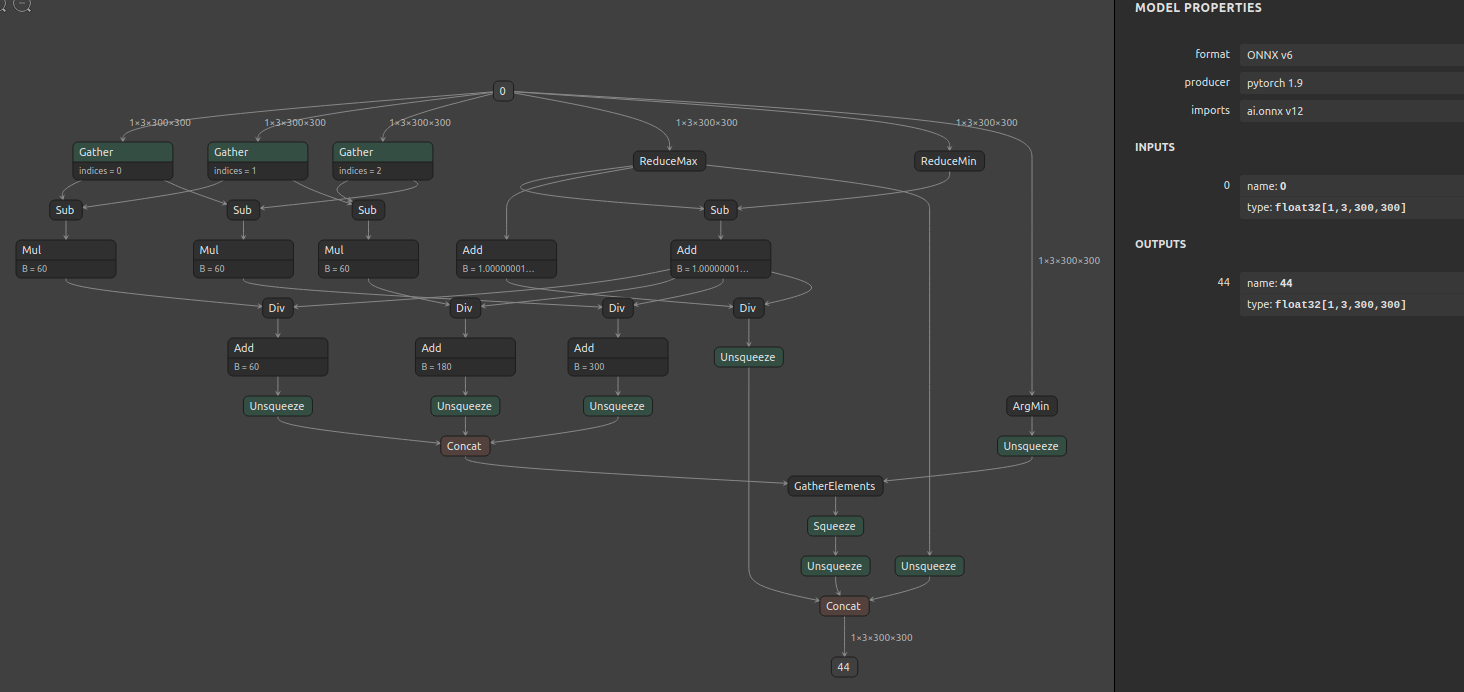
OpenVINO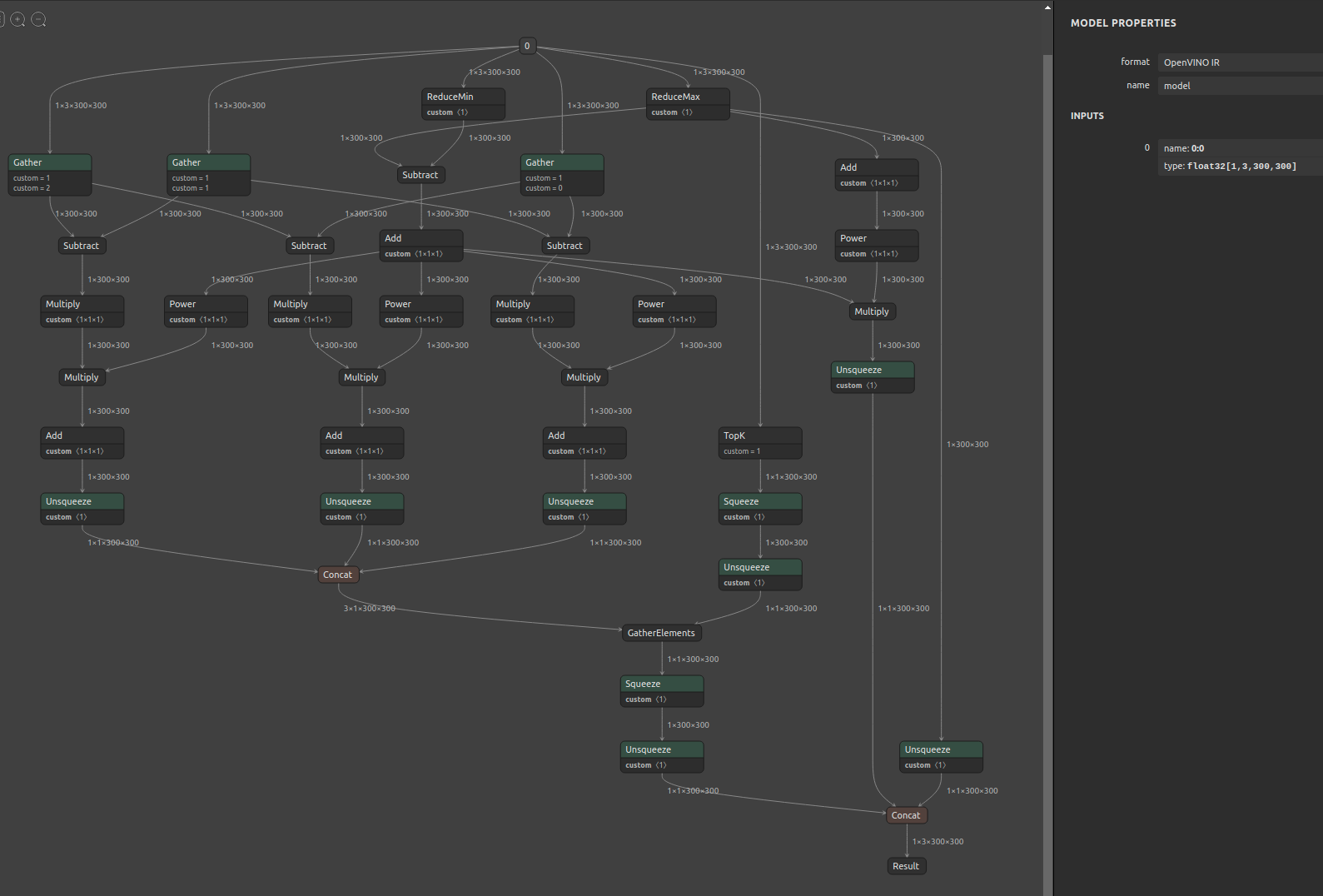
Myriad Blob model.blob.tar.gz
By the way, I don’t know what kind of tool
kornia
is. One last thing. If you need to convert models between frameworks, it is recommended that you do not use boolean masks. This is because the implicit type conversion between True/False and 0/-1 will fail in frameworks that require strict type specification. For example, Myriad or EdgeTPU, TensorFlow Lite.@simetin let’s keep it open until we merge #1329. We need to create a proper mechanism to test from kornia side that after we export to onnx, later is will be usable. Because in this case, the exporter was was working and later you found that error.