Visual bug when used with anchor_id="top"
See original GitHub issueStep 1: Are you in the right place?
- I have verified there are no duplicate active or recent bugs, questions, or requests
- I have verified that I am using the latest version of the library.
Step 2: Describe your environment
- Library version: 1.0-alpha06
- Android version: 8.1.0
- Support library version: 27.1.1
- Device brand: Pixel XL
Step 3: Describe the problem:
I’m using a SpeedDialView
in a CoordinatorLayout
. I’ve anchored the SpeedDialView
to the top of a NestedScrollView
. It looks fine when collapsed but the big button changes position when expanded as shown in this gif:
What I expect to happen is that the menu options appear above the FAB without it jumping down.
Here is my XML layout:
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/coordinatorLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
<android.support.design.widget.AppBarLayout
android:id="@+id/appBarLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/collapsingToolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:contentScrim="?colorAccent"
app:layout_scrollFlags="scroll|exitUntilCollapsed"
app:titleEnabled="false">
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:importantForAccessibility="no"
android:scaleType="centerCrop"
app:layout_collapseMode="parallax"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:importantForAccessibility="no"
android:paddingBottom="@dimen/grid_space_2x"
android:paddingTop="@dimen/grid_space_2x"/>
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@android:color/transparent"
app:layout_collapseMode="pin"
tools:layout_marginTop="@dimen/grid_space_3x"
/>
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
<android.support.v4.widget.NestedScrollView
android:id="@+id/nestedScrollView"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<--Frame layout contents-->
</FrameLayout>
</android.support.v4.widget.NestedScrollView>
<com.leinardi.android.speeddial.SpeedDialOverlayLayout
android:id="@+id/detailsOverlay"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/overlayBackground"/>
<com.leinardi.android.speeddial.SpeedDialView
android:id="@+id/actionsMenu"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="gone"
app:layout_anchor="@+id/nestedScrollView"
app:layout_anchorGravity="top|end"
app:sdExpansionMode="top"
app:sdMainFabClosedSrc="@drawable/ic_add_24dp"
app:sdOverlayLayout="@id/detailsOverlay"
tools:visibility="visible"/>
</android.support.design.widget.CoordinatorLayout>
Issue Analytics
- State:
- Created 5 years ago
- Reactions:1
- Comments:7 (2 by maintainers)
Top Results From Across the Web
What are Visual Bugs : How to find & rectify them | BrowserStack
This article highlights the basics of visual bugs with examples. It will also guide users on how they can eliminate critical visual bugs...
Read more >bizarre visual bug in applications - Microsoft Community
Hi, my computer was working fine after the windows problem solver applied some random setting to try and fix my audio and it...
Read more >Strange visual bug when using the Sunbracers ornament ...
The object completely goes away when taking off Sunbracers or even simply removing the "Eyes of Mercury" ornament for the weapon. Really strange ......
Read more >Visual Bug iOS 14 - iPhone - Apple Support Communities
When I open the App Library page and the widgets page I get very strange visual glitches. Anyone know what the issue here...
Read more >Speckled Visual Glitch | Firefox Support Forum
Hello. In any firefox windows, the glitch (see attached images) is present, Any time i mouse over an interactive elelment, all of the...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Well. I would have never ever thought of that. Thanks! Here is some code if someone else needs it. I used java instead of kotlin (and width instead of height)
@karatektus @leinardi so I’ve found a solution for my issue, albeit in a hacky way. The key is to use the
translationY
property on the Menu item when it’s expanded.So in the layout I posted above the solution would be to listen to the
onToggleChanged
event (when the menu is expanded) and change its translationY property accordingly (i use android-ktx fordoOnNextLayout
here):Result: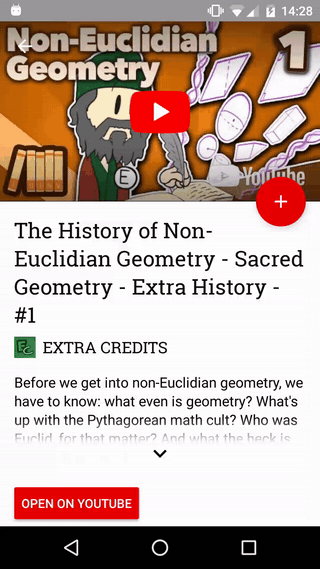