A random output
See original GitHub issueWhen I follow the usage, I got an output image which looks like noise. Something wrong in my code?
import torch
import numpy as np
from PIL import Image
import torchvision.transforms as transforms
import matplotlib.pyplot as plt
def transform_convert(img_tensor, transform):
"""
param img_tensor: tensor
param transforms: torchvision.transforms
"""
if 'Normalize' in str(transform):
normal_transform = list(filter(lambda x: isinstance(x, transforms.Normalize), transform.transforms))
mean = torch.tensor(normal_transform[0].mean, dtype=img_tensor.dtype, device=img_tensor.device)
std = torch.tensor(normal_transform[0].std, dtype=img_tensor.dtype, device=img_tensor.device)
img_tensor.mul_(std[:, None, None]).add_(mean[:, None, None])
img_tensor = img_tensor.transpose(0, 2).transpose(0, 1) # C x H x W ---> H x W x C
if 'ToTensor' in str(transform) or img_tensor.max() < 1:
img_tensor = img_tensor.detach().numpy() * 255
if isinstance(img_tensor, torch.Tensor):
img_tensor = img_tensor.numpy()
if img_tensor.shape[2] == 3:
img = Image.fromarray(img_tensor.astype('uint8')).convert('RGB')
elif img_tensor.shape[2] == 1:
img = Image.fromarray(img_tensor.astype('uint8')).squeeze()
else:
raise Exception("Invalid img shape, expected 1 or 3 in axis 2, but got {}!".format(img_tensor.shape[2]))
return img
ToTensor_transform = transforms.Compose([transforms.ToTensor()])
img = transform_convert(images[0].cpu(), ToTensor_transform)
plt.imshow(img)
plt.savefig('./test_out.png')
Issue Analytics
- State:
- Created a year ago
- Comments:6 (1 by maintainers)
Top Results From Across the Web
Python Random Module: Generate Random Numbers and ...
This lesson demonstrates how to generate random data in Python using a random module. In Python, a random module implements pseudo-random number generators...
Read more >Random Outputs
Here is the list of some features offered by Random Outputs (RO): ... FREE of Cost ✔️ Lightning-Fast Output Generation ✔️ Unlimited ...
Read more >Math.random() - JavaScript - MDN Web Docs
The Math.random() function returns a floating-point, pseudo-random number that's greater than or equal to 0 and ... expected output: a number from 0...
Read more >Python random module
The random module is a built-in module to generate the pseudo-random variables. It can be used perform some action randomly such as to...
Read more >List Randomizer
This page allows you to randomize lists of strings using true randomness, which for many purposes is better than the pseudo-random number algorithms ......
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
for how long did you train? what dataset you used? I trained a little bit on a small custom dataset and I’m getting stuff like this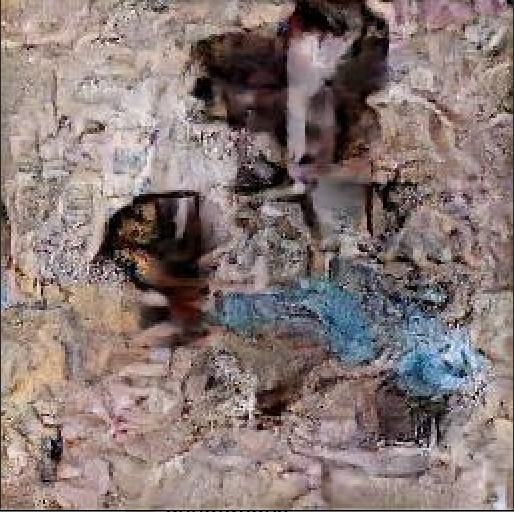
已收到