Error loading discovery document Angular 6.0.3
See original GitHub issueUsing Angular 6.0.3, I am attempting to using this module but keep running into this error. Not sure how to resolve this after about 4 hours of research. Using the Tour of Heroes example from the Angular.io website
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { NgModule } from '@angular/core';
import { HttpClientInMemoryWebApiModule } from 'angular-in-memory-web-api';
import { InMemoryDataService } from './services/in-memory-data.service';
import { HttpClientModule } from '@angular/common/http';
import { AppComponent } from './app.component';
import { HeroesComponent } from './heroes/heroes.component';
import { HeroDetailComponent } from './hero-detail/hero-detail.component';
import { MessagesComponent } from './messages/messages.component';
import { AppRoutingModule } from './/app-routing.module';
import { DashboardComponent } from './dashboard/dashboard.component';
import { HeroSearchComponent } from './hero-search/hero-search.component';
import { AppAuthnModule } from './/app-authn.module';
import { HomeComponent } from './home/home.component';
@NgModule({
declarations: [
AppComponent,
HeroesComponent,
HeroDetailComponent,
MessagesComponent,
DashboardComponent,
HeroSearchComponent,
HomeComponent
],
imports: [
BrowserModule,
FormsModule,
AppRoutingModule,
HttpClientModule,
// The HttpClientInMemoryWebApiModule module intercepts HTTP requests
// and returns simulated server responses.
// Remove it when a real server is ready to receive requests.
HttpClientInMemoryWebApiModule.forRoot(InMemoryDataService, {
dataEncapsulation: false
}),
AppAuthnModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
app.component.ts
import { Component } from '@angular/core';
import { OAuthService } from 'angular-oauth2-oidc';
import { JwksValidationHandler } from 'angular-oauth2-oidc';
import { authConfig } from './app-authn.config';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
title = 'Tour of Heroes';
constructor(private oauthService: OAuthService) {
this.configureWithNewConfigApi();
}
private configureWithNewConfigApi() {
this.oauthService.configure(authConfig);
this.oauthService.tokenValidationHandler = new JwksValidationHandler();
this.oauthService.loadDiscoveryDocumentAndTryLogin();
}
}
app-authn.module.ts
import { Component } from '@angular/core';
import { OAuthService } from 'angular-oauth2-oidc';
import { JwksValidationHandler } from 'angular-oauth2-oidc';
import { authConfig } from './app-authn.config';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
title = 'Tour of Heroes';
constructor(private oauthService: OAuthService) {
this.configureWithNewConfigApi();
}
private configureWithNewConfigApi() {
this.oauthService.configure(authConfig);
this.oauthService.tokenValidationHandler = new JwksValidationHandler();
this.oauthService.loadDiscoveryDocumentAndTryLogin();
}
}
app-authn.config.ts
import { AuthConfig } from 'angular-oauth2-oidc';
export const authConfig: AuthConfig = {
// Url of the Identity Provider
issuer: 'https://steyer-identity-server.azurewebsites.net/identity',
// URL of the SPA to redirect the user to after login
redirectUri: window.location.origin + '/home',
// The SPA's id. The SPA is registered with this id at the auth-server
clientId: 'spa-demo',
// set the scope for the permissions the client should request
// The first three are defined by OIDC. The 4th is a usecase-specific one
scope: 'openid profile email voucher',
showDebugInformation: true
// sessionChecksEnabled: false
};
home.component.ts
import { Component } from '@angular/core';
import { OAuthService } from 'angular-oauth2-oidc';
@Component({
templateUrl: './home.component.html'
})
export class HomeComponent {
constructor(private oauthService: OAuthService) {}
public login() {
this.oauthService.initImplicitFlow();
}
public logoff() {
this.oauthService.logOut();
}
public get name() {
const claims = this.oauthService.getIdentityClaims();
if (!claims) {
return null;
}
return claims;
}
}
home.component.html
<h1 *ngIf="!name">
Hallo
</h1>
<h1 *ngIf="name">
Hallo, {{name}}
</h1>
<button class="btn btn-default" (click)="login()">
Login
</button>
<button class="btn btn-default" (click)="logoff()">
Logout
</button>
<div>
Username/Passwort zum Testen: max/geheim
</div>
Each time I navigate to /home the following error occurs:
error loading discovery document {body: {…}, url: "https://steyer-identity-server.azurewebsites.net/identity/.well-known/openid-configuration", headers: HttpHeaders, status: 404, statusText: "Not Found"}
I can navigate fine to the URL. I am not sure if this is a TLS issue, CORS issue or a problem with the module itself. I do not believe it is a TLS issue since the cert that is served is trusted by the browser and nodejs. I do not believe it is a CORS issues since the author of the identity server would have setup the correct Cross Origin Support. I am running on OSX 10.12.6
Any assistance is appreciated.
Issue Analytics
- State:
- Created 5 years ago
- Reactions:1
- Comments:9
Hello, I have a similar issue, please let me know if my configuration data is correct.
I found 2 error messages:
error loading discovery document
andERROR Error: "Uncaught (in promise): HttpErrorResponse: {"headers":{"normalizedNames":{},"lazyUpdate":null,"headers":{}},"status":0,"statusText":"Unknown Error","url":"https://learn.deluxus.net/oauth/v2/auth","ok":false,"name":"HttpErrorResponse","message":"Http failure response for https://learn.deluxus.net/oauth/v2/auth: 0 Unknown Error","error":{"isTrusted":true}}"
I am facing the below error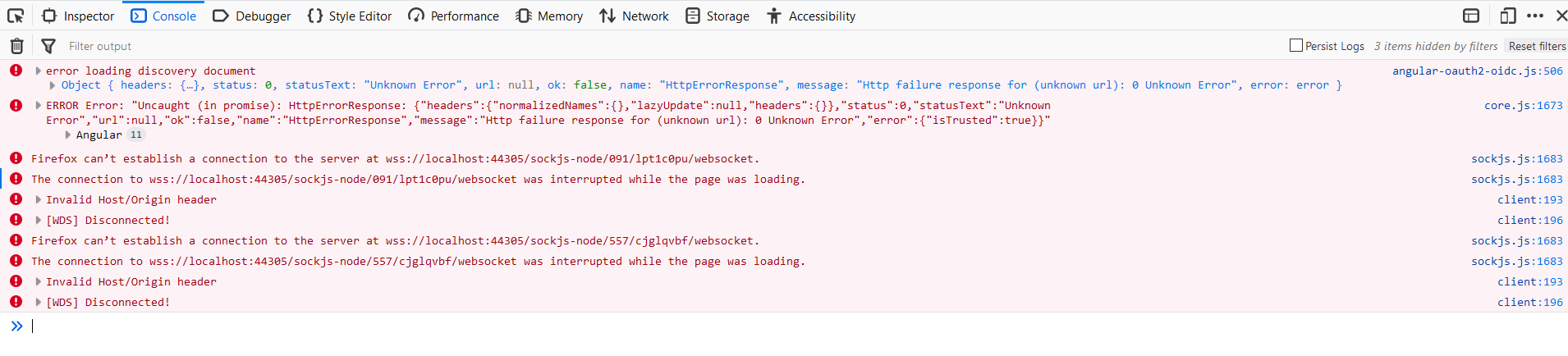