Implicit Symbol.iterator call in for..of loops / spread destructuring doesn't infer `this` generic type parameter
See original GitHub issueTypeScript Version: 4.0.0-dev.20200507
Search Terms: Symbol.iterator type parameter implicit calling for…of loops object spread destructuring this generic
Code
What follows is 3 different ways of iterating over an object using a custom-made iterator. However, using Symbol.iterator
implicitly via the for…of loop fails to produce the same behavior:
const obj = {
x: 1,
y: 2,
z: 3,
*[Symbol.iterator]<T extends object>(
this: T,
): Generator<NonNullable<{ [K in keyof T]: [K, NonNullable<T[K]>] }[keyof T]>, void, unknown> {
for (const entry of Object.entries(this)) yield entry as never;
},
};
{
const iter = obj[Symbol.iterator]();
for (let result = iter.next(); !result.done; result = iter.next()) {
const { value } = result;
value; // ["x", number] | ["y", number] | ["z", number]
}
}
for (const value of obj[Symbol.iterator]()) {
value; // ["x", number] | ["y", number] | ["z", number]
}
for (const value of obj) {
value; // NonNullable<{ [K in keyof T]: [K, NonNullable<T[K]>]; }[keyof T]>
// bad! This should be ["x", number] | ["y", number] | ["z", number]
}
// also, spread destructuring suffers from the same problem!
const values = [...obj]; // Array<NonNullable<{ [K in keyof T]: [K, NonNullable<T[K]>]; }[keyof T]>>
// bad! This should be Array<["x", number] | ["y", number] | ["z", number]>
Expected behavior: for (const value of obj)
should implicitly do the type equivalent of calling our iterator symbol, i.e. for (const value of obj[Symbol.iterator]())
. That means our type parameter should be correctly inferred like so: obj[Symbol.iterator]<T>(this: T)
.
Actual behavior: T
cannot be inferred as the local this
type, so all derivative types cannot be evaluated and the type stays as its generic version.
Related Issues: n/a
Issue Analytics
- State:
- Created 3 years ago
- Reactions:2
- Comments:6 (1 by maintainers)
I fixed it!! I’ll submit a PR soon!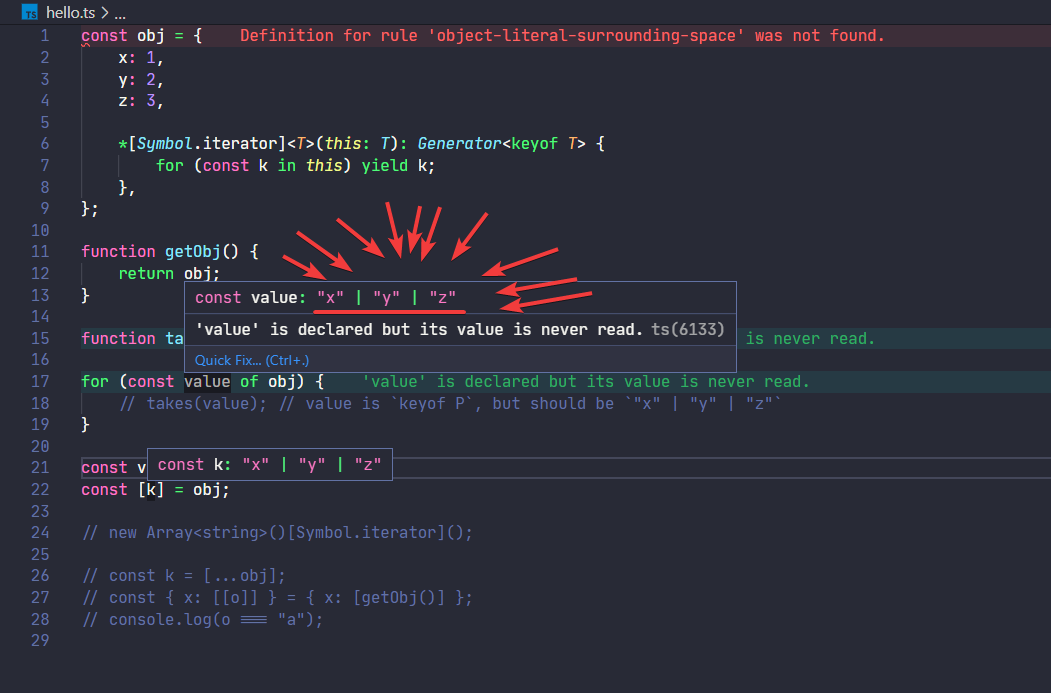
I think I ran into this issue recently. I had code like this
It complains about this.registry not existing but it does exist and the code actually runs fine. I worked around it by simplifying the code but was surprised TS lost track of
this
.