Extruding a 3D surface
See original GitHub issueIs there a correct way to extrude a 2.5/3D surface? At the moment I’m extruding a 2D surface, then editing the ‘original’ vertices (i.e. those left at z=0) with the shape of my surface:
import numpy as np
from scipy.spatial import Delaunay
import trimesh
# Generate coordinates
x = np.arange(0, 10)
y = np.arange(0, 10)
z = np.random.rand(10,10).round(2)
xx, yy = np.meshgrid(x, y)
# Triangulate
coords = np.vstack(np.dstack((xx, yy)))
tris = Delaunay(coords)
# Mesh
mesh = trimesh.creation.extrude_triangulation(
vertices=coords, faces=tris.simplices, height=-10
)
# Edit non-extruded verts with correct z values
verts = mesh.vertices.view(np.ndarray)
verts[verts[:,2] == 0, 2] = z.reshape(-1)
mesh.export(file_obj="mesh.ply");
Thanks for Trimesh – it’s very handy to have a pure python mesh library.
Issue Analytics
- State:
- Created 3 years ago
- Reactions:3
- Comments:5
Top Results From Across the Web
Extruding Surfaces from a 2D or 3D Face - 2018
To extrude from a 2D face, press Alt + select the planar face. You can select either a surface or the face of...
Read more >How to use SOLIDWORKS Surface Extrude without Pre ...
Using the SOLIDWORKS Surface Extrude feature, we can extrude the boundary edges of a face of a solid body or edges of a...
Read more >Extruding a 3D surface - Allplan
To extrude the surface, move the crosshairs into the required direction and click the target point. Use the viewport with the view that...
Read more >About Creating a Solid or Surface by Extruding | AutoCAD 2021
Create a 3D solid or surface by stretching curves into 3D space. The EXTRUDE command creates a solid or surface that extends the...
Read more >Is there a way to extrude the entire selected surface? - Reddit
You can create a plane normal to the direction of whatever surface you're wanting to extrude, and then create a sketch on it,...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Dear all,
I also would like to know how to achieve an extrusion for 3D surfaces. Please help. Here an example for a better explanation of my problem:
1.) Input mesh plane: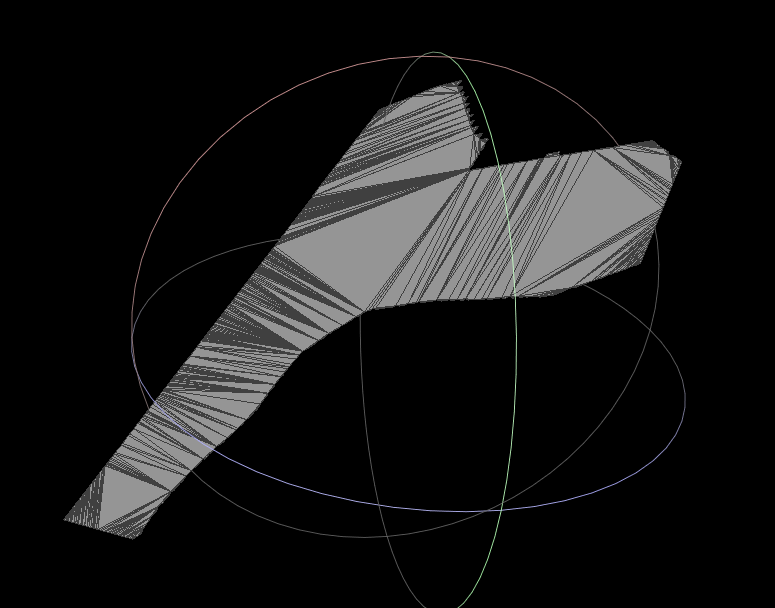
2.) Output using trimesh extrusion for 2D triangulation (ignoring z values):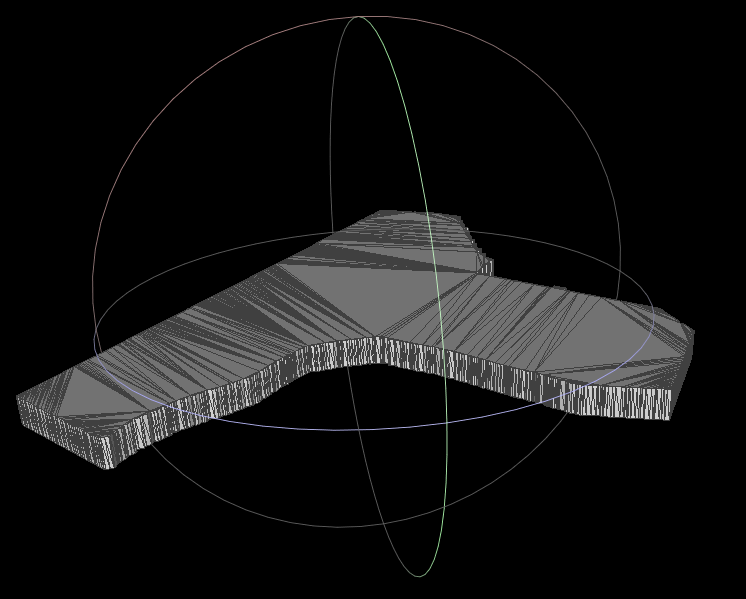
3.) Output using the proposed approach of @dt99jay for considering the z values: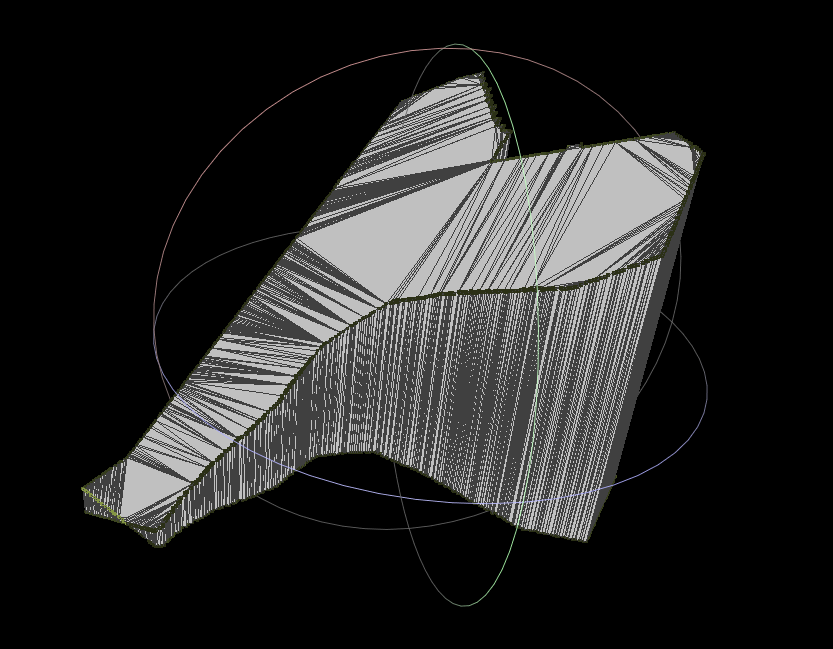
4.) Expected result (I used the extrusion function in Blender for this case):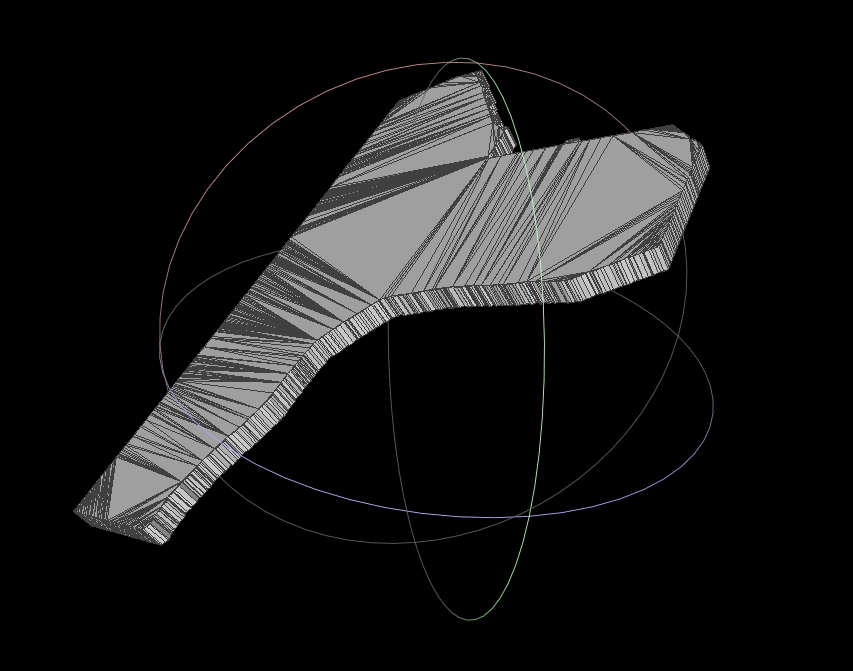
Do you know how I can get the same result such as in 4.) with trimesh or any other python library?
Hi @dylanjkline, for my use-case I only needed to extrude along a straight line.
I tried it by creating a sphere using
sphere = trimesh.creation.icosphere(subdivisions=3, radius=2)
The result of my
extrude_convex_mesh()
creates a convex mesh of the extruded part - i.e. the sphere is still there as-is ( drawn in gray in my screenshot ) and the extruded mesh is “clued” to it ( drawn in red ), but both meshes are not connected.That may not exactly what you expect it to be - I guess you expect that the extruded part is replacing the “upper” part of the sphere in the example screenshot, which is actually not the case.