Merging / joining meshes
See original GitHub issueHow can two overlapping meshes be merged?
The figures and scripts / code snippets below describe the problem I’m trying to solve:
- At each of three points there is a sphere of radius 0.1:
- Point A: (0, 0, 0)
- Point B: (1, 0, 0)
- Point C: (2, 1, 0)
I want to create a mesh that surrounds these three points, something like this:
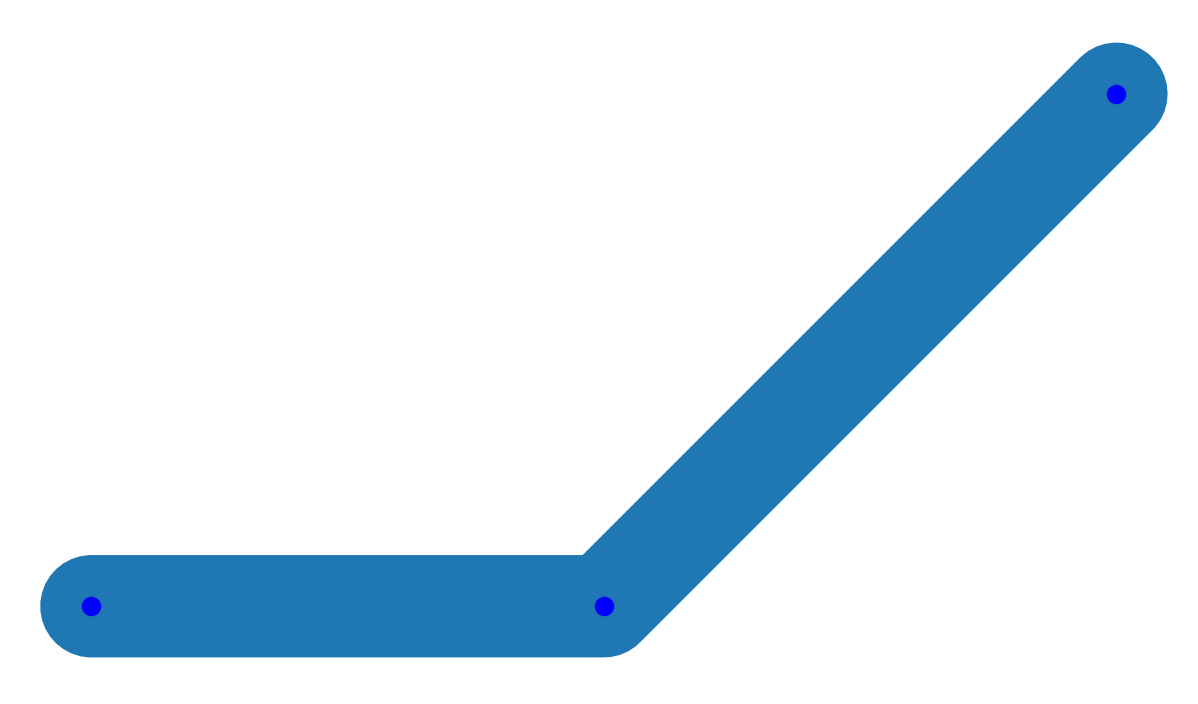
- The script below uses
convex_hull
to create a mesh over all A, B and C, but not in a piecewise manner:
from math import pi,ceil
import numpy as np
import trimesh
def generate_sphere_vertices(r, rad=0.1, n=36):
theta = np.linspace(0, pi, ceil(n/2))
phi = np.linspace(-pi, pi, n)
x0,y0,z0 = r
[t,p] = np.meshgrid(theta, phi)
phi,theta = t.flatten(), p.flatten()
x = x0 + rad * np.sin(theta) * np.cos(phi)
y = y0 + rad * np.sin(theta) * np.sin(phi)
z = z0 + rad * np.cos(theta)
return np.vstack( [x,y,z] ).T
vertsA = generate_sphere_vertices( (0,0,0) )
vertsB = generate_sphere_vertices( (1,0,0) )
vertsC = generate_sphere_vertices( (2,1,0) )
verts = np.vstack( [ vertsA, vertsB,vertsC ] )
pc = trimesh.PointCloud( verts )
mesh = pc.convex_hull
mesh.show()
The result looks like this:
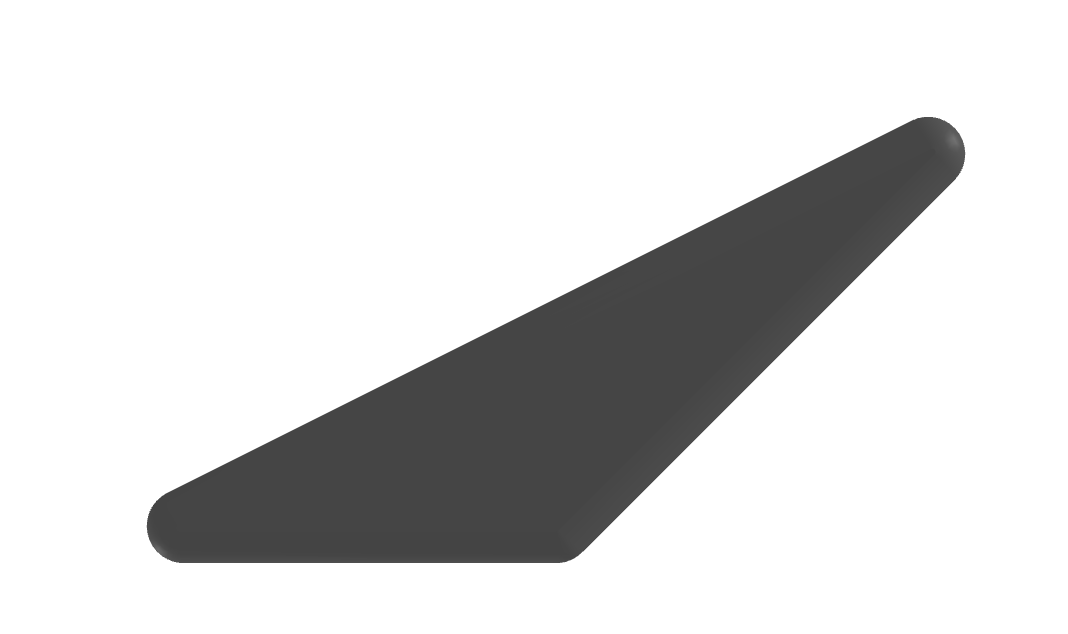
To create a piecewise mesh over AB, then BC I tried to use union
, as in the script below, but this does not work.
vertsAB = np.vstack( [ vertsA , vertsB ] )
vertsBC = np.vstack( [ vertsB , vertsC ] )
pcAB = trimesh.PointCloud( vertsAB )
pcBC = trimesh.PointCloud( vertsBC )
meshAB = pcAB.convex_hull
meshBC = pcBC.convex_hull
mesh = meshAB.union(meshBC)
mesh.show()
The resulting mesh looks the same as meshAB
.
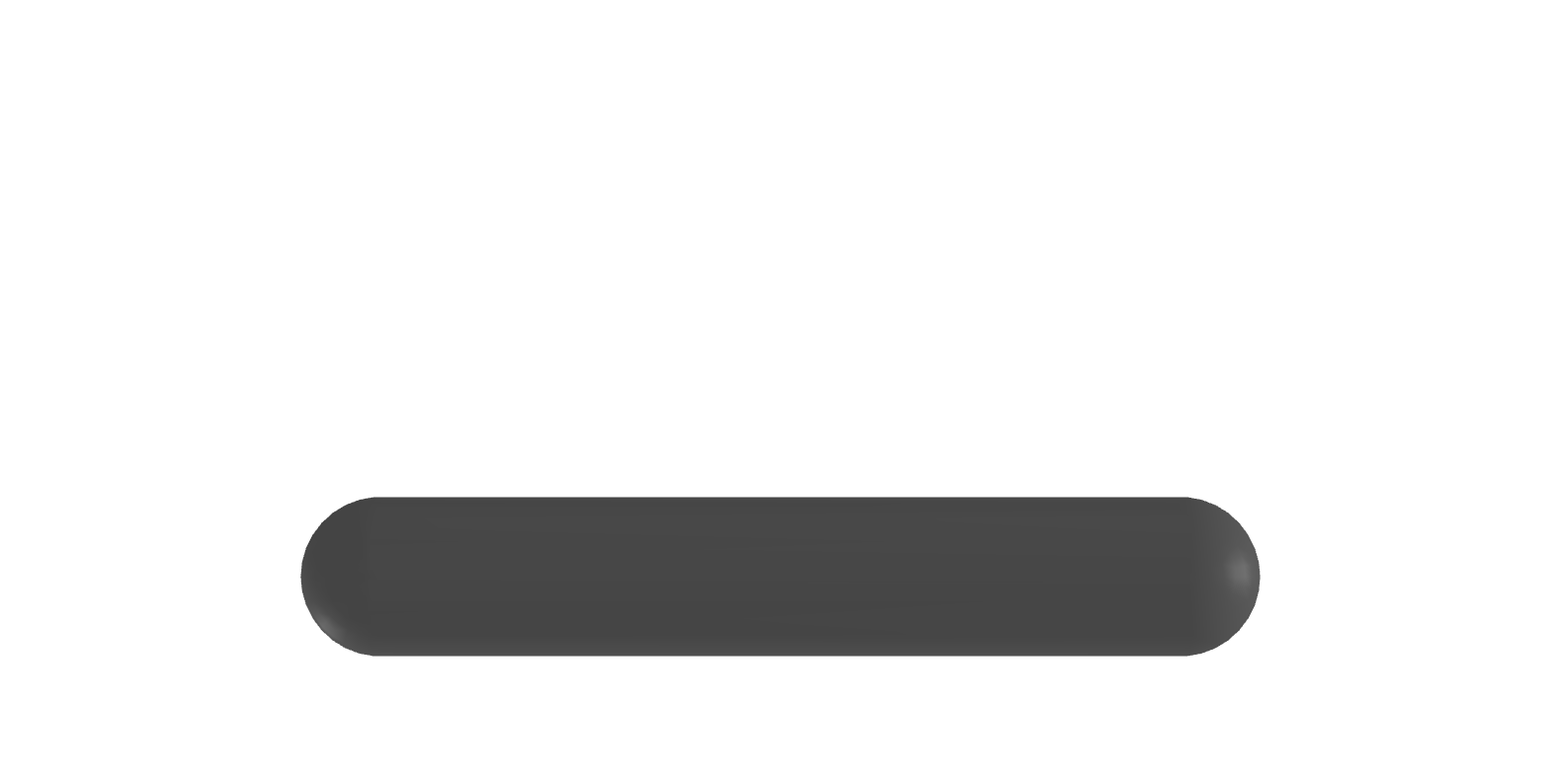
Is it possible to join or merge the two meshes?
What is union
actually doing?
Issue Analytics
- State:
- Created 4 years ago
- Comments:6 (2 by maintainers)
Top Results From Across the Web
Merge multiple meshes into one single mesh?
Select the separate objects that you want to combine. Press Ctrl + Numpad + , to perform a union operation · Combine two...
Read more >Blender: Merge Objects – Simply Explained - All3DP
Here's how to join two objects: Left-click to select the first object – the one that you don't want to be the parent....
Read more >Blender 2.9 Tutorial for Merging Meshes, Joining ... - YouTube
blender #3dmodeling #tutorialLearn how to merge multiple meshes into one so you can use free 3D models and use them in any game...
Read more >Join Mesh Objects – Blender Knowledgebase - KatsBits.com
Once a collection of mesh objects is available, from the Object menu top-left of the 3D View, select Join from the options available...
Read more >How to Merge Objects in Blender - MakeUseOf
Joining Objects · Select the first object by left-clicking on it. This would be the non-parent object. · Once selected, hold down the...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Oops! I just noticed that this question was already answered in #245, sorry about that!
I used:
But I still don’t understand what
union
is doing in the case above. Why doesn’tunion
work?the concatenate command from above works as expected: