Question: Cypress MSW started, but not intercepted
See original GitHub issueI’m not sure where the best place to ask questions, so I’m asking them here. This is a simple example and I’ll abstract it more when I can get the simple example working. What I’m trying to do is load MSW as part of the Cypress tests and not part of the application itself. This will allow me use use MSW to mock any part of the application — just like fixtures
do in Cypress. The benefit being that MSW allows me to inspect the request before responding, something Cypress doesn’t allow at the moment.
Environment
Name | Version |
---|---|
msw | ^0.20.5 |
browser | 85.0.4183.83 |
OS | macOS 10.15.6 |
Request handlers
// App.js in CRA
function App() {
const [message, setMessage] = useState()
useEffect(() => {
setTimeout(() => {
fetch('https://jsonplaceholder.typicode.com/todos/1')
.then(response => response.json())
.then(json => console.log(json))
}, 1000)
}, [])
return (
<div className="App">
<h1>{message}</h1>
<div>
<button type="button" onClick={() => setMessage('First')}>
First
</button>
<button type="button" onClick={() => setMessage('Second')}>
Second
</button>
<button type="button" onClick={() => setMessage('Third')}>
Third
</button>
</div>
</div>
)
}
// In Cypress test
import { setupWorker, rest } from 'msw'
describe('First', () => {
let worker
beforeEach(() => {
cy.visit('/', {
onBeforeLoad(win) {
worker = setupWorker(
rest.get(
'https://jsonplaceholder.typicode.com/todos/1',
(req, res, ctx) => {
return res(
ctx.json({
userId: 1,
id: 1,
title: 'Lord of the rings',
completed: false,
}),
)
},
),
)
worker.start()
win.msw = { worker, rest }
},
})
})
it('should be able to set the first message', () => {
cy.window().then(win => {
console.log(win.msw)
})
cy.findByRole('button', { name: /first/i }).click()
cy.findByRole('heading', { name: /first/i }).should('be.visible')
})
})
Actual request
// Example of making a request. Provide your code here.
fetch('https://jsonplaceholder.typicode.com/todos/1')
.then(response => response.json())
.then(json => console.log(json))
Current behavior
I can see from the console that MSW was started and that it is available on the window
. The request says it went though the serviceworker, but it actually get the response back from the API and not MSW.
Expected behavior
I was hoping it would be intercepted by MSW and the response sent back. Obviously something about the manner in which I’m doing this doesn’t work. It would be great to get some input as to why, maybe there is some other way I can do this.
Response should be
{
"userId": 1,
"id": 1,
"title": "Lord of the rings",
"completed": false,
}
Screenshots

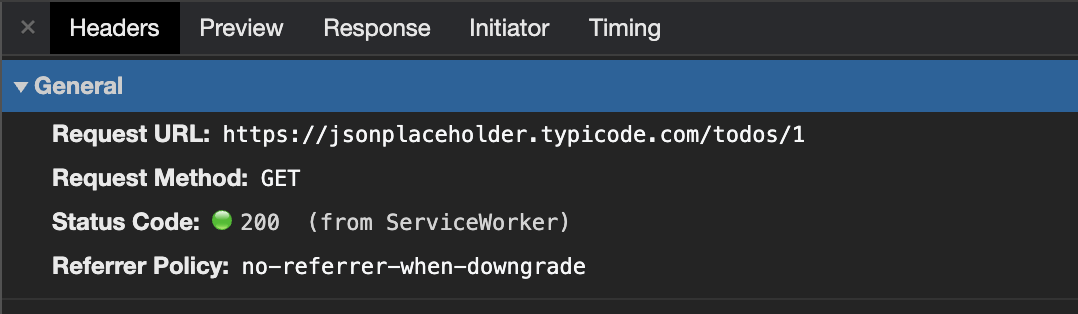
Issue Analytics
- State:
- Created 3 years ago
- Reactions:12
- Comments:23 (5 by maintainers)
@msutkowski that example expects it to be loaded as part of the application (index.tsx). It works if I do that. However, what I want to achieve is to make
msw
not a dependency of the application, but add it in as part of Cypress. It’s adding it inonBeforeLoad
, this way, I can make a global server in Cypress, then add custom commands to set up routes just likefixtures
work in Cypress. It is getting loaded and available in the window, but the route doesn’t get intercepted.I’ve been a little bit busy lately, but I will make sometime on this one. I need a bit of input from the the team. I’ll wait to hear back on some of the questions, and then tackle some of the suggestions from @kettanaito