Tilemaps Won't Load (Using Phaser3 Project Template)
See original GitHub issueVersion
- Phaser Version: 3.17.0
- Operating system: Linux Mint 19
Description
I am loading a tilemap exported from Tiled. It’s pretty simple and I followed the recommended export to json. Unfortunately I am getting some warnings and the screen appears blank. Here are the warnings I get in the console:
No map data found for key map ParseToTilemap.js:59
Invalid Tileset Image: RPGpack_sheet Tilemap.js:323
Invalid Tilemap Layer ID: Background Tilemap.js:736
Invalid Tilemap Layer ID: Blocked Tilemap.js:736
Example Test Code
Here is my class that extends Phaser.Scene where I attempt to load and create the tilemaps:
import 'phaser';
export default class GameScene extends Phaser.Scene {
constructor(key) {
super(key);
}
preload() {
this.load.tilemapTiledJSON('map', 'assets/tilemaps/map.json');
this.load.spritesheet('RPGpack_sheet', 'assets/images/RPGpack_sheet.png', {
frameWidth: 64,
frameHeight: 64
});
}
create() {
this.map = this.make.tilemap({key: 'map'});
this.tiles = this.map.addTilesetImage('RPGpack_sheet');
this.backgroundLayer = this.map.createStaticLayer('Background', this.tiles, 0, 0);
this.blockedLayer = this.map.createStaticLayer('Blocked', this.tiles, 0, 0);
}
}
and this is where I am using that class. I have verified it works fine as long as I am not trying to load these tilemaps:
import Phaser from "phaser";
import config from './config'
import GameScene from './scenes/Game';
class Game extends Phaser.Game {
constructor() {
super(config);
this.scene.add('Game', GameScene);
this.scene.start('Game');
}
}
window.game = new Game();
I’m also using this config file but otherwise I have left everything as is from the phaser3 project tempate
import 'phaser';
export default {
type: Phaser.AUTO,
parent: "phaser-example",
width: 800,
height: 800,
pixelArt: true,
roundPixels: true,
physics: {
default: 'arcade',
arcade: {
gravity: {y: 0},
debug: true
}
}
}
Additional Information
I printed out the value of this.map after creating it in the create method and got these values back:
currentLayerIndex: 0
format: null
height: 10
heightInPixels: 320
imageCollections: Array []
images: Array []
layers: []
length: 0
<prototype>: Array []
objects: {}
orientation: "orthogonal"
properties: Object { }
renderOrder: "right-down"
scene: Object { sys: {…}, game: {…}, anims: {…}, … }
tileHeight: 32
tileWidth: 32
tilesets: Array []
version: "1"
width: 10
widthInPixels: 320
many values are wrong (i.e tileHeight and tileWidth should be 64) but it gets things like height and width correct, perhaps this is just because 10 is default and this is a coincidence.
I have tried other json files for my tilemaps and still have the same issue so I am led to believe that it isn’t an issue with the tilemap I am using. This is rather hard for me to debug, due to the fact I am using the project template so I don’t have a specific solution in mind. Thank you!
Edit 1: I changed a line from the GameScene create function. Now it appears as:
create() {
this.map = this.make.tilemap('map');
this.tiles = this.map.addTilesetImage('RPGpack_sheet');
this.backgroundLayer = this.map.createStaticLayer('Background', this.tiles, 0, 0);
this.blockedLayer = this.map.createStaticLayer('Blocked', this.tiles, 0, 0);
}
Now this got rid of the first warning No map data found for key map ParseToTilemap.js:59
but the other 3 remain
Issue Analytics
- State:
- Created 4 years ago
- Comments:14 (6 by maintainers)
Make sure that the tileset image name match tileset name in Tiled, and the layer name in the code match the layer name in Tiled, as it described in this article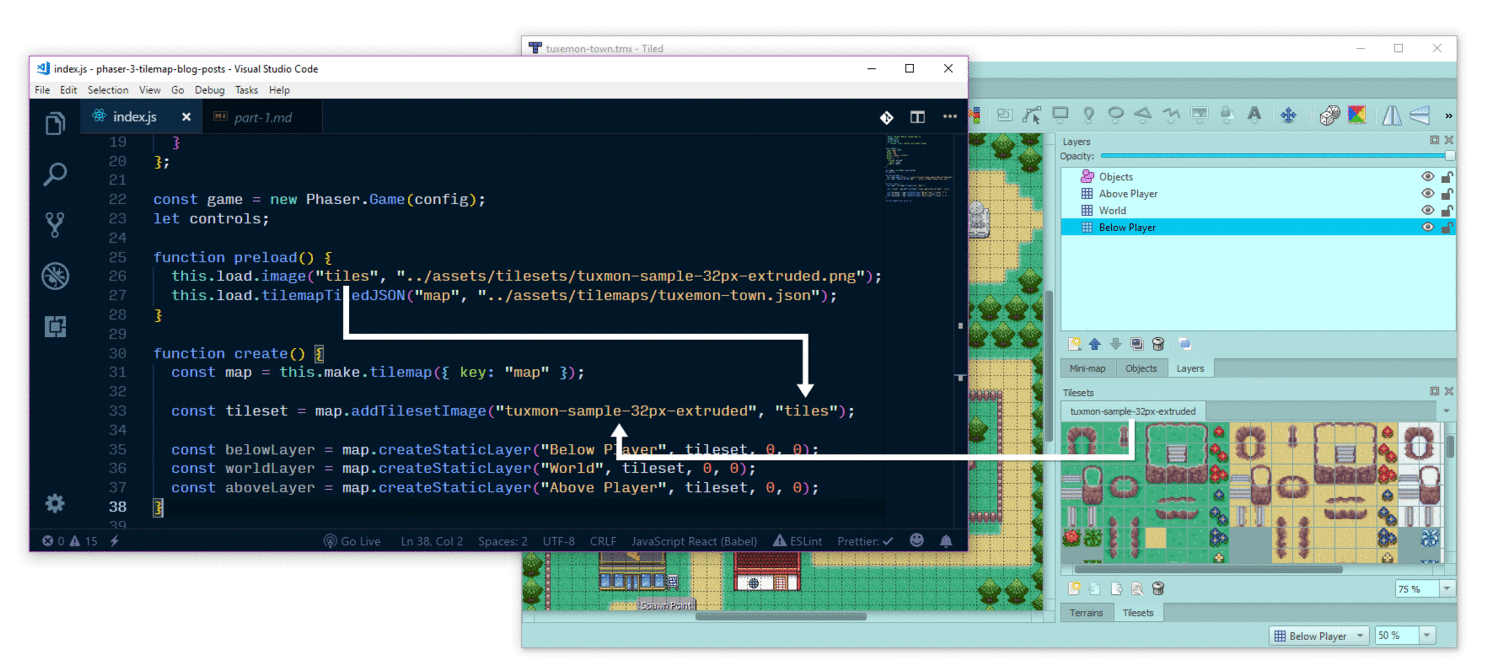
Thanks for all the help and hints!! I finally got it to work. This is the final code that I used to get it working:
So after checking the network tab I noticed I was getting 404s for loading in the images and json files. I tried changing the path to begin at src and this fixed mostly everything. So I suppose somewhere my root path isn’t set correctly. As of now I’m fine just specifying
src/assets/...
Then of course I should have been passing the object as
{key: 'map'}
rather than just passing'map'
tothis.make.tilemap()
as I was in the beginning. Even though this got rid of one of my original warnings, it was apparently why it was arriving as a string in ParseToTilemap