Pixi BlurFilter showing interference from other sprites.
See original GitHub issueHi, I’m having problems blurring images with transparent backgrounds. I’m animating multiple sprites with transparent backgrounds. The edges of each sprite seem to pick up the colour from the sprites around them as they move. The colour doesn’t always correspond to the sprite itself, so I’m assuming it picks up the other sprites’ colours somehow.
I’m running this on Pixi 5.1.5 on Chrome 78, Mac Mojave. Unfortunately I can’t show a link as it’s a client project I can’t share, but I’ve attached a screenshot of the effect, which I’m hoping will help identify.
Some excerpts of what I’m hoping are the relevant bits of code. Please let me know if anything else would be useful!
Initialising:
// Create a Pixi Application
this.pixi.app = new this.pixi.Application({
antialias: true,
transparent: true
});
// Add the Pixi created canvas to the HTML document
this.stage.appendChild(this.pixi.app.view);
// Canvas setup
this.pixi.app.renderer.autoDensity = true;
this.pixi.app.renderer.resize(window.innerWidth, window.innerHeight);
// Add sprites
this.pixi.loader.add(this.shapeURLs)
.load((loader, resources) => this.setupShapes(loader, resources));
Setting up sprites:
this.pixi.container = new this.pixi.Container();
for (let i = 0; i < this.requiredShapes; i++) {
let shapeSprite;
const texture = resources[`${this.shapesDirectory}/shape${this.shapes[i].shapeNum}-blur-0.png`].texture;
shapeSprite = new this.pixi.Sprite(texture);
shapeSprite.anchor.set(0.5, 0.5);
shapeSprite.resolution = 2;
shapeSprite.alpha = 0;
this.shapes[i].filter = new this.pixi.filters.BlurFilter(8);
shapeSprite.filters = [this.shapes[i].filter];
this.shapes[i].sprite = shapeSprite;
this.pixi.container.addChild(shapeSprite);
shapeSprite.scale.set(this.minScale, this.minScale);
}
this.pixi.app.stage.addChild(this.pixi.container);
and from the render function:
this.shapes[i].cycleTime = timestamp - this.shapes[i].cycleStartTime;
// Fade the shape in right at the start
const alphaVal = this.shapes[i].cycleTime / 1000 > 1 ? 1 : this.shapes[i].cycleTime / 1000;
if (this.shapes[i].alpha !== alphaVal) {
this.shapes[i].alpha = alphaVal;
this.shapes[i].sprite.alpha = alphaVal;
}
if (this.shapes[i].cycleTime >= this.shapes[i].duration) {
this.shapes[i].cycleStartTime = timestamp;
} else {
const fractionComplete = this.shapes[i].cycleTime / this.shapes[i].duration;
// Set the z which will be used to project 3d position to 2d
this.shapes[i].z = this.maxZ - this.maxZ * fractionComplete;
/*
* The zFocusPoint is the point in a shape's motion towards the screen at
* which it should be in focus. pauseForNTextures is how many textures' worth
* we'll keep it in focus (setting the pause by a number of textures requires
* less calculation than setting it as a decimal).
*/
const zFocusPoint = 0.65;
this.shapes[i].filter.blur = this.maxBlur - this.maxBlur * fractionComplete / zFocusPoint;
// Scale the shape up to full scale
const scale = this.minScale + (1 - this.minScale) * fractionComplete * fractionComplete; // easeInQuad
this.shapes[i].sprite.scale.set(scale, scale);
}
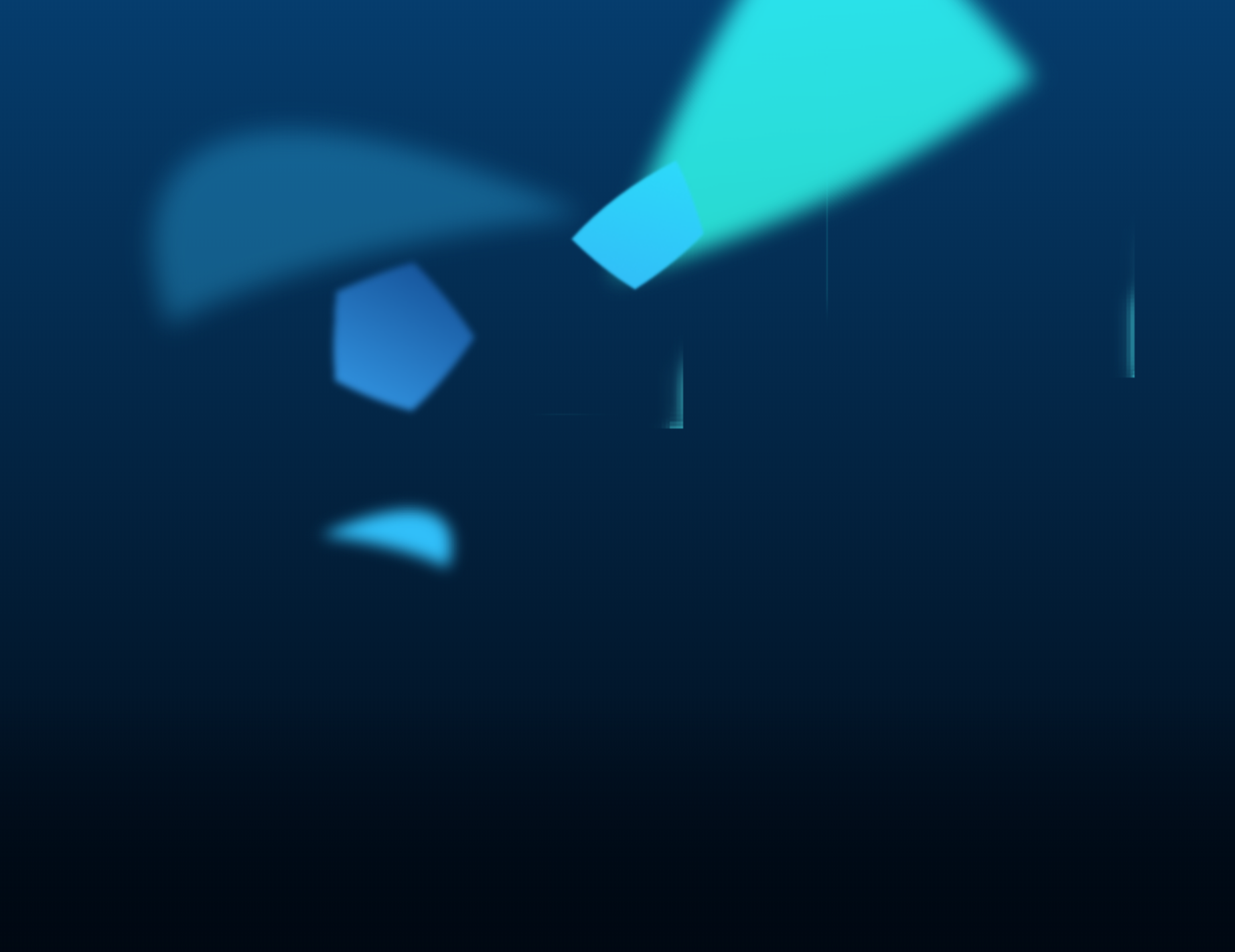
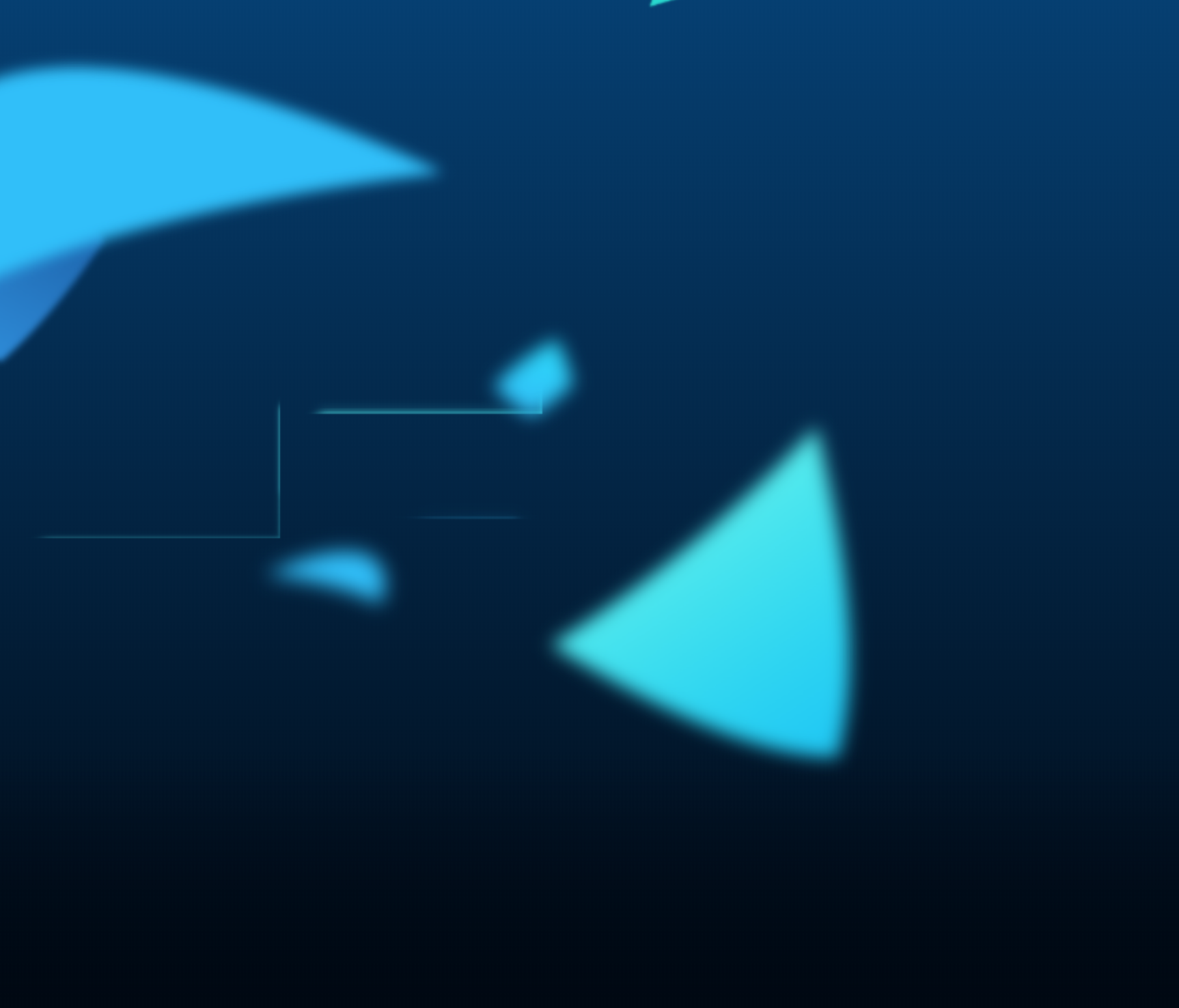
Thanks in advance!!!
Issue Analytics
- State:
- Created 4 years ago
- Comments:11 (1 by maintainers)
OK, temporary solution is here: https://www.pixiplayground.com/#/edit/kuhrhc4jG5QnRRtIfwGwk
Basically i went here: https://github.com/pixijs/pixi.js/blob/dev/packages/filters/filter-blur/src/BlurFilterPass.js#L88
and changed
true
tofalse
@jteutenberg can you make a Pull request to fix that in the pixi-filters repo?