Fields are ignored in WHERE clause
See original GitHub issueBug description
We use the following model for a table with uniq compound key by employeeId
& paymentDate
model Payslip {
id String @id @default(uuid())
status String
employeeId String
employee Employee @relation(fields: [employeeId], references: [id])
paymentDate DateTime
# rest of fields
@@unique([employeeId, paymentDate], name: "uniqKeyByEmployeeIdAndPaymentDate")
}
Queries like findFirst
, findMany
, updateMany
(maybe some other, haven’t check yet) ignore some fields in a where clause.
Query examples:
const { count } = await this.prisma.payslip.updateMany({
where: {
AND: [
{ employeeId: { in: pluck('id', employees) } },
{ status: Status.Uploaded },
{ paymentDate: new Date(paymentDate) },
{ publishedAt: null },
],
},
data: {
status: Status.Published,
publishedAt: new Date(),
updatedAt: new Date(),
},
})
const { count } = await this.prisma.payslip.updateMany({
where: {
status: Status.Uploaded,
paymentDate: new Date(paymentDate),
employeeId: { in: pluck('id', employees) },
publishedAt: null,
},
data: {
status: Status.Published,
publishedAt: new Date(),
updatedAt: new Date(),
},
})
The output from logging is the following
SELECT `payslip`.`Payslip`.`id` FROM `payslip`.`Payslip` WHERE (`payslip`.`Payslip`.`status` = ? AND 1=1 AND `payslip`.`Payslip`.`employeeId` IN (?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?) AND `payslip`.`Payslip`.`publishedAt` IS NULL) ["UPLOADED","1","10","103","104","11","12","13","14","15","16","17","18","19","19007763-e05e-472e-a840-c3e40dcedc91","1bc472b4-2fed-456b-9a0a-63de068edb66","2","20","21","22","23","24","25","26","3","302bb32d-03ff-41e8-9915-185b65d77b67","31","32","36","39","4","40","41","44","47","48","49","5","50","51","54","55","6","69","7","70","71","72","73","74","8","88","89","9","e01e5c65-2315-47eb-ae71-db446c180279","e5edb7f1-5de0-45d0-b5f1-265b6dd9ebb3","eebaac6e-afd8-489a-9804-127e697ca00c"]
UPDATE `payslip`.`Payslip` SET `updatedAt` = ?, `status` = ?, `publishedAt` = ? WHERE `payslip`.`Payslip`.`id` IN (?,?,?,?,?,?,?,?,?,?,?) [2020-10-21 07:25:04.376 UTC,"PUBLISHED",2020-10-21 07:25:04.376 UTC,"03882468-0abd-434c-afb1-f74b0549e498","18b0b22d-eaf2-4621-89d5-4f99dae8be21","5d877156-8040-411e-af3e-fd5f66a5b367","7b65281a-0ed1-45a8-bb66-a461ded2461e","919a15b9-a5d2-497e-8316-8d77bd7febfd","9ddaa446-733c-4437-a2ab-23dbbe60d14d","a196dc31-73a1-4988-9d79-b47e63de89dd","bbb39f70-4d1b-440c-a714-69ed415e5780","bf395bf3-c019-4ce4-97f9-2b716155b562","e715fa49-0758-47f2-a053-e8039078c818","ef96351e-0fc2-45f3-b2f6-9edffe2b98b6"]
From the log fields status
, paymentDate
, publishedAt
are missed from WHERE clause
Expected behavior
Fields which are in a where clause should not be ignored.
Environment & setup
- @prisma/cli - 2.9.0
- @prisma/client - 2.9.0
- OS: Alpine Linux v3.11
- Database: MySQL
- Node.js version: v12.18.4
Issue Analytics
- State:
- Created 3 years ago
- Comments:8 (4 by maintainers)
Top Results From Across the Web
SQL Server - where clause condition being ignored
Your where clause isn't being ignored. You need to understand the order of precedence of your predicates. Add parenthesis where they need to...
Read more >How to remove Condition from where clause for the fields ...
In case user is selecting only 1 parameter, rest other parameters are being used in SQL with DEFAULT values example @NULLDATE for Date...
Read more >Ignore part of a where clause if null - MSDN - Microsoft
If the OP tried to return all records on NULL in the search parameter, say, by using the technique "WHERE @SomeParam IS NULL...
Read more >field_definitions Clause - Oracle Help Center
The ALL FIELDS OVERRIDE clause tells the access driver that all fields are present and that they are in the same order as...
Read more >MySQL 8.0 Reference Manual :: 13.2.9 LOAD DATA Statement
The syntax of the FIELDS and LINES clauses is the same for both ... With neither IGNORE nor LOCAL , data-interpretation errors terminate...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
@matthewmueller This is unrelated to the date format. This related to client generating the wrong query which is sent to the engine.
When using
new Date()
(aka passing a plain js object in the reproduction), the following query is sent to the engine which has empty date field:But when you pass ISO date string to the client
new Date().toISOString()
, it sends this query to the engine which returns the desired result:Diff: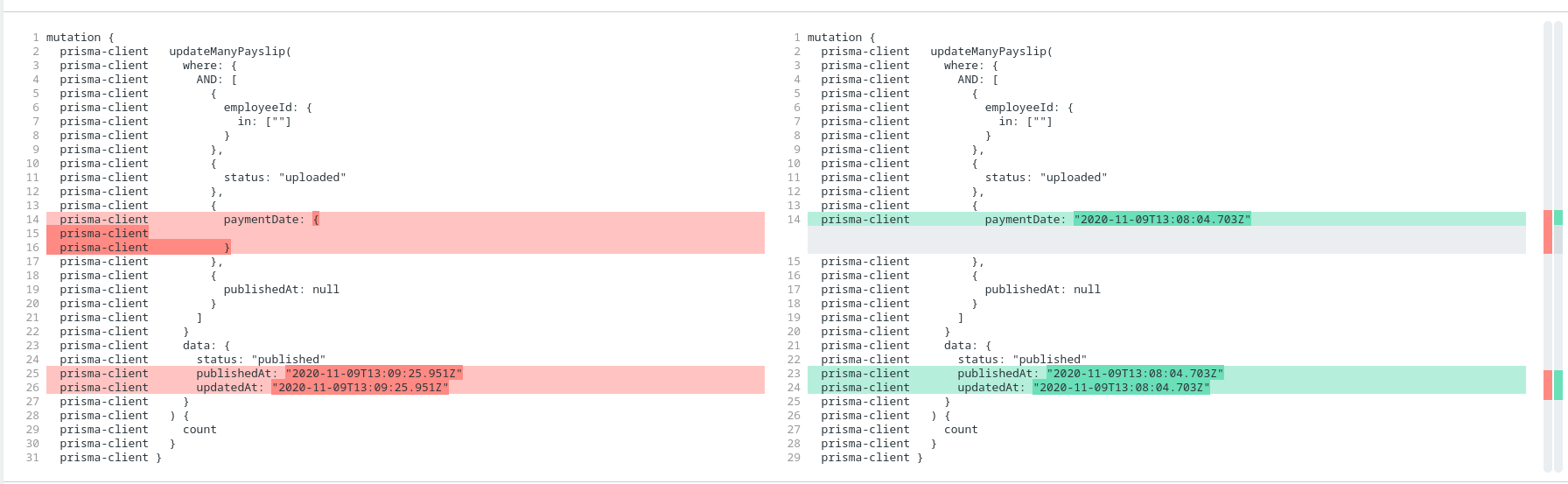
So there is a bug in client query generation. cc/ @timsuchanek
If only
paymentDate
is dropped, then this issue is likely related: https://github.com/prisma/prisma/issues/3987