Slow Nested Queries - taking 2 seconds plus
See original GitHub issueBug description
Hi guys,
I’m building a reddit clone right now with Prisma
+ AWS
(as PostgreSQL Hoster) + Next.js
. I’m fetching a subreddit with all details on it (mainly posts and subscribed users) in getServerSideProps
. I make a fetch request to my api and in my api I make a call to my prisma database (hosted on Amazon AWS - PostgreSQL). This maybe related to #2481
Here is the working live example (just select a subreddit in the dropdown). Next.js has some posts, learnprogramming hasnt: https://reddit-clone-teal.vercel.app/
Is that slow / fast to you? For me it’s a bit slow with that little data (Vercel Analytics = 3 seconds) 😢
Here is the api (/api/subreddit/findSubreddit
)
const handler = async (req: NextApiRequest, res: NextApiResponse) => {
try {
const sub = await prisma.subreddit.findUnique({
where: { name: String(req.query.name) },
include: {
posts: {
include: { subreddit: true, user: true },
},
joinedUsers: true
},
});
console.log("here is the sub", sub);
if (!sub) {
return res.status(500).json({ error: "No sub was found with this name" });
}
res.json(sub);
} catch (e) {
res.json(e);
}
};
So nothing too crazy right (it’s 3 posts total)? Or am I including too much in the call? Anyway when I transition to a subreddit and this function runs (there are currently 3 dummy posts in the db) it takes sometimes 1.5 - 2 seconds after the page has loaded in. With such little data this should be much less loading time am I right?
I’m using swr
and here is how a subreddit page looks like:
First the getServerSideProps
function:
export async function getServerSideProps(ctx) {
const url = `${process.env.NEXTAUTH_URL}/api/subreddit/findSubreddit?name=${ctx.query.sub}`;
const fullSub = await fetchData(url);
return {
props: {
fullSub,
},
};
}
And then on the page itself (/pages/subReddits/[sub]
):
const { data: fullSub, error } = useSWR(
`/api/subreddit/findSubreddit?name=${sub}`,
fetchData,
{
initialData: props.fullSub,
}
);
Here is the log from prisma when I hit this api:
prisma:query SELECT “public”.“Subreddit”.“id”, “public”.“Subreddit”.“name”, “public”.“Subreddit”.“displayName”, >“public”.“Subreddit”.“infoBoxText”, “public”.“Subreddit”.“createdAt” FROM “public”.“Subreddit” WHERE >“public”.“Subreddit”.“name” = $1 LIMIT $2 OFFSET $3 prisma:query SELECT “public”.“Post”.“id”, “public”.“Post”.“title”, “public”.“Post”.“body”, “public”.“Post”.“subredditId”, >“public”.“Post”.“userId”, “public”.“Post”.“createdAt”, “public”.“Post”.“updatedAt” FROM “public”.“Post” WHERE >“public”.“Post”.“subredditId” IN ($1) OFFSET $2 prisma:query SELECT “public”.“users”.“id”, “public”.“users”.“name”, “public”.“users”.“email”, “public”.“users”.“email_verified”, >“public”.“users”.“image”, “public”.“users”.“created_at”, “public”.“users”.“updated_at” FROM “public”.“users” WHERE >“public”.“users”.“id” IN ($1) OFFSET $2 prisma:query SELECT “public”.“_SubredditToUser”.“A”, “public”.“_SubredditToUser”.“B” FROM “public”.“_SubredditToUser” WHERE >“public”.“_SubredditToUser”.“A” IN ($1) prisma:query SELECT “public”.“users”.“id”, “public”.“users”.“name”, “public”.“users”.“email”, “public”.“users”.“email_verified”, >“public”.“users”.“image”, “public”.“users”.“created_at”, “public”.“users”.“updated_at” FROM “public”.“users” WHERE >“public”.“users”.“id” IN ($1) OFFSET $2
Here a screenshot from Vercel Analytic:
Can you figure it out? Any ideas why this might long load and not cache? The db is hosted in frankfurt (on AWS) don’t know if that’s important to you. But im located in Austria and my ping to Frankfurt is 45ms. The api request is faster when opened on my local machine (localhost) (project running locally i mean), but also not “fast”
Full link to my repo: https://github.com/SelfDevTV/reddit-clone
If you need Anything please let me know I will test it out.
Thank you so much! PS: Prisma rocks!
How to reproduce
Go to my link and try it out: https://reddit-clone-teal.vercel.app/
Expected behavior
It should be way faster to load the 3 posts on my site.
Environment & setup
- OS: Windows 10 latest
- Database: PostgreSQL hosted on AWS (Frankfurt EU)
- Node.js version: v14.15.1
- Prisma version:
@prisma/cli : 2.13.1
@prisma/client : 2.13.1
Current platform : windows
Query Engine : query-engine fcbc4bb2d306c86c28014f596b1e8c7980af8bd4 (at node_modules\@prisma\engines\query-engine-windows.exe)
Migration Engine : migration-engine-cli fcbc4bb2d306c86c28014f596b1e8c7980af8bd4 (at node_modules\@prisma\engines\migration-engine-windows.exe)
Introspection Engine : introspection-core fcbc4bb2d306c86c28014f596b1e8c7980af8bd4 (at node_modules\@prisma\engines\introspection-engine-windows.exe)
Format Binary : prisma-fmt fcbc4bb2d306c86c28014f596b1e8c7980af8bd4 (at node_modules\@prisma\engines\prisma-fmt-windows.exe)
Studio : 0.329.0
Issue Analytics
- State:
- Created 3 years ago
- Reactions:1
- Comments:9 (3 by maintainers)
Hello @SelfDevTV
I am pretty sure this is a deployment issue. I deployed your app to vercel and with a digitalocean database(pgbouncer enabled and it is hosted in the San Francisco region) here: https://prisma-issue-4884.vercel.app/
Repository: https://github.com/harshit-test-org/prisma-issue-4884
If you inspect this deployment, you will see it is as fast as the database numbers that you are sharing.
Vercel deploys all the lambdas to SFO1 region of AWS by default. You can confirm this by logging
VERCEL_REGION
environment variable as you can see in the above screenshot.So please make sure your database is hosted in the same region as your vercel lambda deployments. Otherwise network will add heavy latency.
Here is the result of the performance route(https://prisma-issue-4884.vercel.app/api/performance?name=nextjs):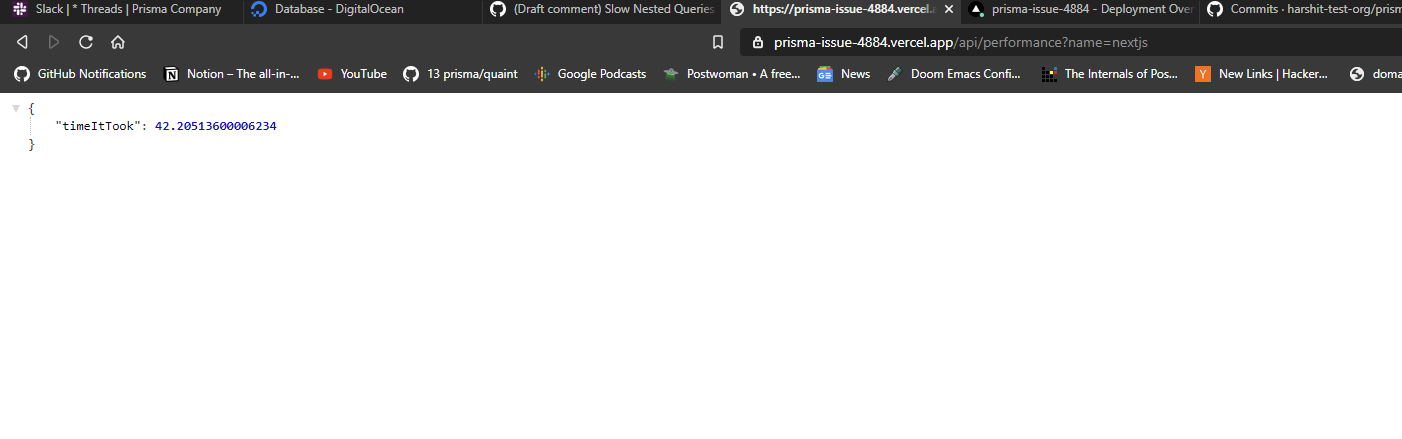
I will also post the vercel analytics result for this deployment once their dashboard updates. Right now it show 100 for the home route:
Edit(posted):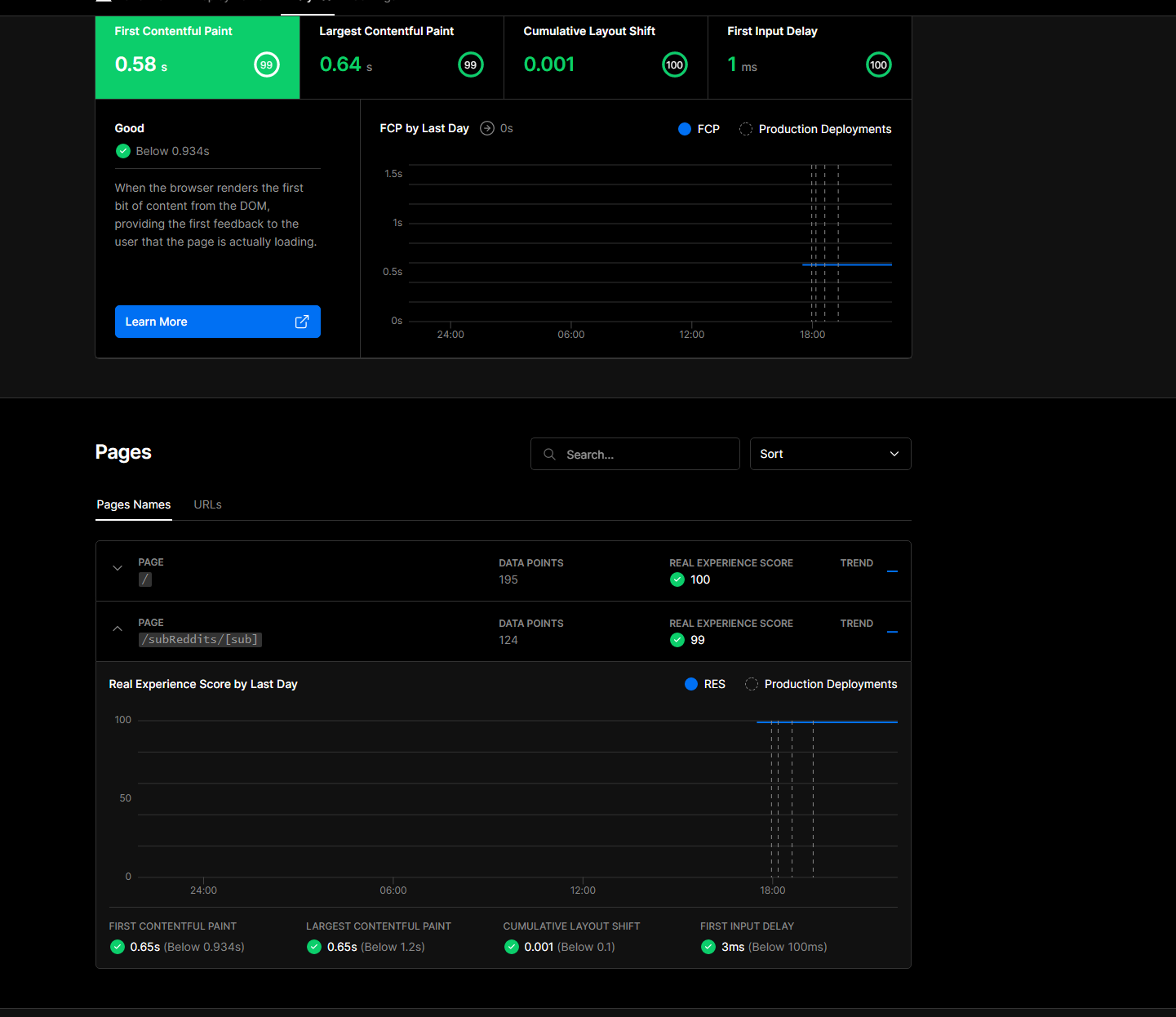
Thanks for the confirmation