Log polar transformation in torchvision.transforms
See original GitHub issue🚀 The feature
Log polar transformation for images, which maps an image from the 2D Cartesian coordinate system to the log-polar system. This transformation each pixel [x, y] to the (ρ, θ) coordinate system, where ρ is the log of the distance from the center and θ is the angle.
Motivation, pitch
I’ve been researching ways of making one of my CNNs rotation invariant and happened to find this paper. I looked all over the docs for a similar transformation but couldn’t find any, so I was wondering if this transformation could be included in the torchvision.transforms
module in order to make it easier to do this type of preprocessing on images.
Alternatives
No response
Additional context
I already have a minimal implementation for my personal use that is based on existing transformations in the package. If you think this feature will be useful, I could polish it up and open a pull request to merge it.
Issue Analytics
- State:
- Created 2 years ago
- Comments:5 (2 by maintainers)
@aftoul meanwhile you can use the following code as a custom transformation for you use-case. This implementation uses OpenCV to warp the image:
Code
Usage
Output
Original: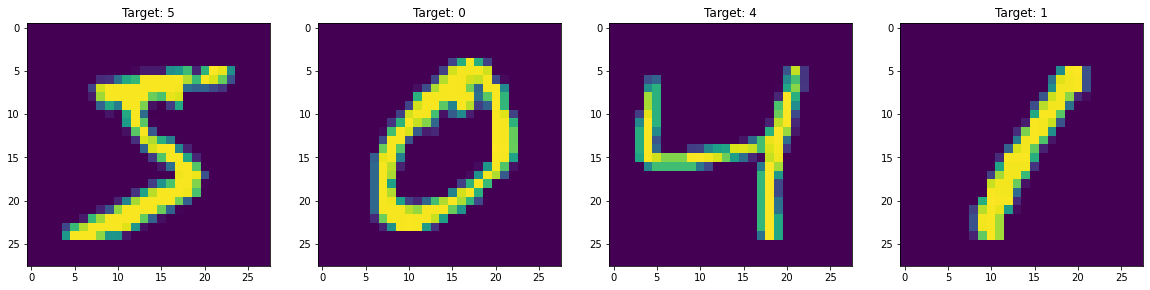
Log-Polar: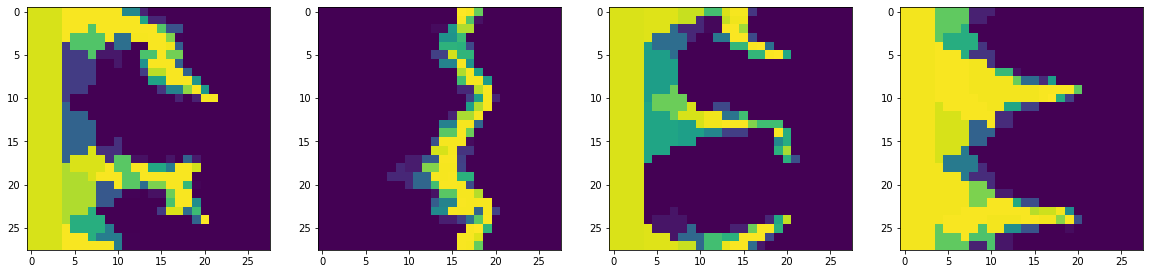
Reconstruction: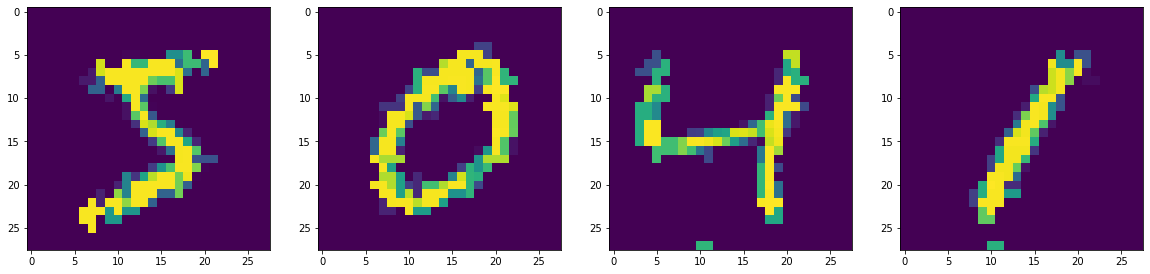
HTH
Maybe, it is about providing Log-Polar and its inverse transformations. An image from the paper on MNIST:
Image from the paper…