How can I combine drag by the handle with custom drag preview?
See original GitHub issueThe problem is that I have the same problem as here. Here is the main insight from that issue:
Make sure ItemDragPreview isnt also a DragSource
But how can I implement it, cause I need a DragPreview as a dnd handler?
This is how dnd handlers are implemented:
function collectSource(connect, monitor) {
return {
connectDragPreview: connect.dragPreview(),
connectDragSource: connect.dragSource(),
isDragging: monitor.isDragging(),
};
}
function collectTarget(connect, monitor) {
return {
connectDropTarget: connect.dropTarget(),
isOver: monitor.isOver(),
};
}
const taskSource = {...}
const taskTarget = {...}
class NormalRow extends Component {
componentDidMount() {
const { connectDragPreview } = this.props;
if (connectDragPreview) {
// Use empty image as a drag preview so browsers don't draw it
// and we can draw whatever we want on the custom drag layer instead.
connectDragPreview(getEmptyImage(), {
// IE fallback: specify that we'd rather screenshot the node
// when it already knows it's being dragged so we can hide it with CSS.
captureDraggingState: true,
});
}
}
render() {
const element = (
<div>
{columns.map(this.renderCell)}
</div>
);
return connectDropTarget(connectDragPreview(
<div>
{connectDragSource(<div className="dnd__item" />)}
{element}
</div>,
));
}
}
export default flow(
DragSource('entity', taskSource, collectSource),
DropTarget('entity', taskTarget, collectTarget),
)(NormalRow);
This is how I trying to create a custom dragLayer:
class CustomDragLayer extends React.Component {
renderItem = (type, item) => {
switch (type) {
case 'entity':
return <EntityDragPreview item={item} />;
default:
return null;
}
};
render() {
const { item, itemType, isDragging } = this.props;
if (!isDragging) return null;
return (
<div style={layerStyles}>
<div style={getItemStyles(this.props)}>
{this.renderItem(itemType, item)}
</div>
</div>
);
}
}
export default DragLayer(collect)(CustomDragLayer);
class EntityDragPreview extends React.Component {
render() {
const { item: { renderRow, row, draggingRowNumber } } = this.props;
return (
<div style={{ position: 'relative' }}>
<dsiv style={{ backgroundColor: 'red', position: 'absolute', left: '-20px' }}>
{renderRow(row, 3, true, draggingRowNumber)}
</dsiv>
</div>
);
}
}
export default EntityDragPreview;
Here is screenshot: https://ibb.co/X4P7JgD grey background - shadow from draggable element, white background - HTML styles, which I want to remove, red background - custom drag preview.
Issue Analytics
- State:
- Created 5 years ago
- Comments:8 (3 by maintainers)
Top Results From Across the Web
Handles and Previews - React DnD
React DnD lets you choose the draggable node, as well as the drag preview node in your component's render function. You may also...
Read more >How to Add Drag and Drop in React with React Beautiful DnD
Drag and drop is pretty much what it sounds like — it's an interaction allowing someone to click and drag an item, then...
Read more >Drag operations - Web APIs - MDN Web Docs - Mozilla
A drag may include data items of several different types. This allows data to be provided in more specific types, often custom types, ......
Read more >How To Create Drag and Drop Elements with Vanilla ...
Dragging -and-dropping is a common user interaction that you can find in many graphical user interfaces. There are pre-existing JavaScript ...
Read more >Drag and drop - Android Developers
A drag and drop operation starts when the user makes a UI gesture that your app recognizes as a signal to start dragging...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
@darthtrevino how to implement custom drag layer to this code ?
I managed to combine drag source + drop target with drag preview by repeating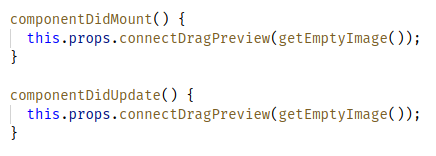
this.props.connectDragPreview(getEmptyImage());
at componentDidUpdate method of drag source component like so