Infinite loop of "callbacks_poll"
See original GitHub issueSo, it’s YOU! 😃
I’ve been seeing this endless loop of “callbacks_poll” that is killing my debugging performance and pleasure.
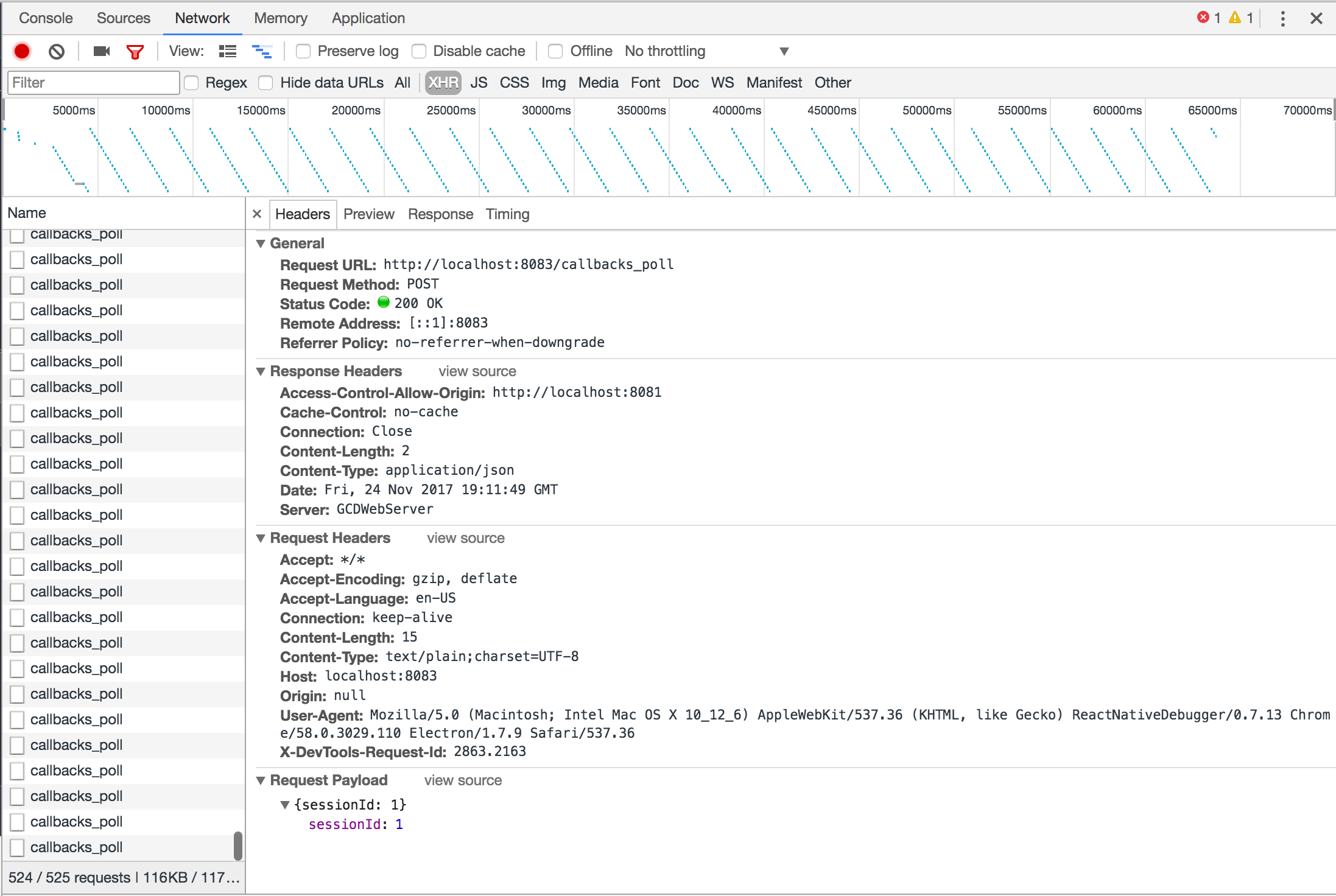
Goals
Get rid of the endless loop of callbacks_poll in my networking request pane and the associated memory leak
Expected Results
No callbacks_poll visible and no memory leak
Actual Results
See image. Endless loop. Makes it hard to identify individual network requests and there seems to be an associated memory leak, although I can’t guarantee that it is related.
Steps to Reproduce
Not sure. This happened after I upgraded Realm but I didn’t realize it at the time. Here’s my Realm setup
Code Sample
class User {}
User.schema = {
name: 'User',
primaryKey: 'id',
properties: {
id: { type: 'int' },
nickName: { type: 'string' },
displayName: { type: 'string' },
title: { type: 'string', optional: true },
email: { type: 'string' },
photo: { type: 'string', optional: true },
photoDir: { type: 'string' },
gender: { type: 'string' },
refId: { type: 'string', optional: true },
sessionToken: { type: 'string', optional: true }
}
};
export default User;
import Realm from 'realm';
import { logoutUser } from 'modules/login/actions/loginActions';
import { delToken, clearTokens } from 'modules/login/actions/oauthActions';
import * as config from 'config';
import { dbg } from 'utils';
import UserRecord from 'data/records/userRecord';
import {
EMIT_USER,
GET_USER,
STORE_USER,
DEL_USER,
CLEAR_ALL
} from '../userActionTypes';
import User from '../schemas/userDbSchema';
const realmSettings = {
schema: [User],
schemaVersion: 1,
migration: (oldRealm, newRealm) => {
// only apply this change if upgrading to schemaVersion 1
/* if (oldRealm.schemaVersion < 1) {
const oldObjects = oldRealm.objects('User');
const newObjects = newRealm.objects('User');
// loop through all objects and set the name property in the new schema
for (let i = 0; i < oldObjects.length; i++) {
newObjects[i].sessionToken = undefined;
}
} */
}
};
const realm = new Realm(realmSettings);
export function getUser() {
return {
type: GET_USER
};
}
export function emitUser(user) {
return {
type: EMIT_USER,
user
};
}
export function loadUser(userId) {
return async (dispatch, getState) => {
dispatch(getUser());
const sessionUser = getState().users.sessionUser;
if (sessionUser && sessionUser.id === userId) return sessionUser;
let user = null;
if (userId) user = realm.objectForPrimaryKey('User', userId);
else {
const users = realm.objects('User');
if (users.length > 0) user = users.values().next().value;
}
dbg(user ? `User ${user.id} loaded` : 'No user loaded');
if (user) {
dispatch(emitUser(new UserRecord(user)));
return user;
}
return null;
};
}
Version of Realm and Tooling
- Realm JS SDK Version: 2.0.11
- Node or React Native: React Native 0.49.5
- Client OS & Version: Mac Os
- Which debugger for React Native: React-Native-Debugger or Chrome
Issue Analytics
- State:
- Created 6 years ago
- Reactions:38
- Comments:67 (16 by maintainers)
Top Results From Across the Web
Infinite loop callbacks_poll React Native Debugger
I see this endless loop in the debugger network pane, both in Chrome and React Native Debugger using iOS Simulator and RN 0.49.5....
Read more >Avoiding infinite loops inside JavaScript callbacks - a TDD ...
Avoiding infinite loops inside JavaScript callbacks - a TDD approach ... JavaScript callbacks are most commonly implemented as functions, ...
Read more >Implementing listener and callback, infinite loop - MathWorks
Hi, · My problem follows the one I described here: · So in my example, a class "Car" as properties "Brand" and "Color"....
Read more >What is the event loop in Node.js? - Educative.io
This phase is used to invoke setImmediate() callbacks. If the poll phase is empty or callbacks using setImmediate() have been queued, the event...
Read more >The Node.js Event Loop, Timers, and process.nextTick()
When one of these operations completes, the kernel tells Node.js so that the appropriate callback may be added to the poll queue to...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
@blagoev I think it’s a high priority issue because the chrome debugger turn very slow and we almost cannot do any thing. Thanks.
This issue is causing my app to be almost impossible to work on; the app/debugger is painfully slow and crashes often. Please, please prioritise fixing this!