setting center coordinate changes zoom level after PR #1538 was merged
See original GitHub issueDescribe the bug
Before #1538 was by @naftalibeder merged, setting the center coordinate only resulted in the camera panning to the new center without changing the zoom level.
After #1538 was merged, the camera zooms in to the center coordinate.
To Reproduce
I modified the bug report template. Click on “set center” to see the new behavior:
import React from 'react';
import {Button} from 'react-native';
import {
MapView,
ShapeSource,
SymbolLayer,
CircleLayer,
Camera,
} from '@react-native-mapbox-gl/maps';
import bbox from '@turf/bbox';
const styles = {
mapView: {flex: 1},
circleLayer: {
circleRadiusTransition: {duration: 5000},
circleColor: '#ff0000',
},
};
const features = {
type: 'FeatureCollection',
features: [
{
type: 'Feature',
id: 'a-feature',
properties: {
icon: 'example',
text: 'example-icon-and-label',
},
geometry: {
type: 'Point',
coordinates: [-74.00597, 40.71427],
},
},
{
type: 'Feature',
id: 'b-feature',
properties: {
text: 'just-label',
},
geometry: {
type: 'Point',
coordinates: [-74.001097, 40.71527],
},
},
{
type: 'Feature',
id: 'c-feature',
properties: {
icon: 'example',
},
geometry: {
type: 'Point',
coordinates: [-74.00697, 40.72427],
},
},
],
};
function getCameraBounds(geojsonObject) {
const horizontalPadding = 100;
const verticalPadding = 100;
const mapBbox = bbox(geojsonObject);
const cameraSettings = {
bounds: {
ne: [mapBbox[0], mapBbox[1]],
sw: [mapBbox[2], mapBbox[3]],
paddingBottom: horizontalPadding,
paddingTop: horizontalPadding,
paddingLeft: verticalPadding,
paddingRight: verticalPadding,
},
padding: {
paddingBottom: horizontalPadding,
paddingTop: horizontalPadding,
paddingLeft: verticalPadding,
paddingRight: verticalPadding,
}
};
return cameraSettings;
}
class BugReportExample extends React.Component {
state = {
radius: 20,
centerCoordinate: undefined
};
render() {
const cameraDefaultSettings = getCameraBounds(
features,
);
const circleLayerStyle = {
...styles.circleLayer,
...{circleRadius: this.state.radius},
};
return (
<>
<Button
title="Set Center"
onPress={() => this.setState({centerCoordinate: [-74.00597, 40.71427]})}
/>
<MapView style={styles.mapView}>
<Camera defaultSettings={cameraDefaultSettings} centerCoordinate={this.state.centerCoordinate} />
<ShapeSource id={'shape-source-id-0'} shape={features}>
<CircleLayer id={'circle-layer'} style={circleLayerStyle} />
<SymbolLayer
id="symbol-id"
style={{
iconImage: ['get', 'icon'],
}}
/>
</ShapeSource>
</MapView>
</>
);
}
}
export default BugReportExample;
Expected behavior
When setting the center coordinate of the camera, zoom level SHOULD NOT change.
Actual behavior
Setting the center coordinate of the camera causes zoom level to change.
Videos
The different behavior is shown in the following gifs:
[EDITED BY ferdicus to have a side by side comparison and save space]
BEFORE | AFTER |
---|---|
22df62cb is the commit right before #1538 was merged | 6e7b0a4d is the commit of #1538 |
![]() |
![]() |
Issue Analytics
- State:
- Created 2 years ago
- Comments:7 (4 by maintainers)
Top Results From Across the Web
Electromagnetic Emission from Supermassive Binary Black ...
The "cutout" region covering the polar-coordinate origin at the center of mass appears in the center in black in the plots of Figures...
Read more >Charts/CHANGELOG.md at master · danielgindi/Charts - GitHub
Fixed bugs: Line charts: Line sections disappear when zoomed in (has PR). ... After setup i am trying to build the code it...
Read more >BUILDING RESILIENT SUPPLY CHAINS, REVITALIZING ...
Mr. President: It is our privilege to transmit to you the first set of reports that your Administration has developed pursuant.
Read more >PR-1538 Potting and Molding Compound - PPG Aerospace
This material is designed for potting electrical connectors, molding electrical cables and mechanical parts, circuit board coating and/or electrical embedding ...
Read more >Annual Report 2020 - JPMorgan Chase
and JPMorgan Chase & Co. merger ... Consistently ranked #1 in Markets revenue since ... funds, sovereign wealth funds and central banks.
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
@schiller-manuel @mfazekas @ferdicus I think this is all fair. Even without a new zoom specified, it makes sense to cache the previous zoom level and use that as an updated “default”.
@mfazekas To answer your question, in order to get padding to work with
centerCoordinate
, I needed to switch the SDK method used to center on the coordinate.I think this is the conditional that @schiller-manuel is hitting without a zoom, meaning it falls back on the altitude returned from bounding around a single point - that’s why it zooms in so much in his example. That would be a bug, which is my fault.
Like I said before, my instinct would say to immediately fall back on the zoom default, but I would be fine looking for the previous cached zoom (if any) first, and then the default if there isn’t one.
I can work on this. I’ll also look into this. Sound okay?
@naftalibeder looks good to me, I tried your PR with my reproducer, the result is as expected: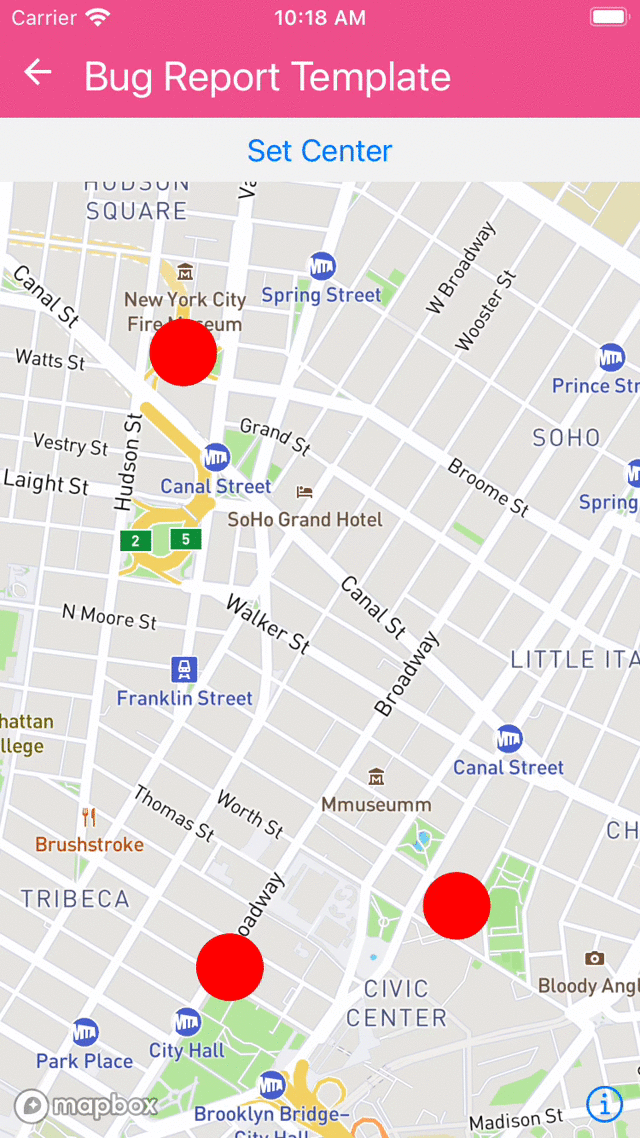