React-Native-Skia parity with React-Native-SVG Circle
See original GitHub issueI’ve been experimenting with React-Native-Skia trying to do some of the things we can do with React-Native SVG. Currently I am trying to create a circular progress indicator. Problem is, there doesn’t seem to be a way with Circle
to offset the stroke. This is about as far as I could get with Skia.
export const DonutChart: FC<CircularProgressProps> = ({
strokeWidth,
radius,
backgroundColor,
percentageComplete,
}) => {
const innerRadius = radius - strokeWidth / 2;
const circumfrence = 2 * Math.PI * innerRadius;
return (
<View style={styles.container}>
<Canvas style={StyleSheet.absoluteFill}>
<Circle
cx={radius}
cy={radius}
r={innerRadius}
color={'purple'}
style={'stroke'}
strokeWidth={20}
strokeCap="round"
/>
</Canvas>
</View>
);
};
In react-native-svg on the other hand we could just do something like this:
export const CircularProgress: FC<CircularProgressProps> = ({
radius,
strokeWidth,
backgroundColor,
percentageComplete,
}) => {
const innerRadius = radius - strokeWidth / 2;
const circumfrence = 2 * Math.PI * innerRadius;
return (
<View style={styles.container}>
<Svg style={StyleSheet.absoluteFill}>
<Circle
cx={radius}
cy={radius}
r={innerRadius}
fill={'transparent'}
stroke={backgroundColor}
strokeDasharray={`${circumfrence} ${circumfrence}`}
strokeWidth={strokeWidth}
strokeDashoffset={2 * Math.PI * (innerRadius * 0.5)}
strokeLinecap="round"
/>
</Svg>
</View>
);
};
then get the following result
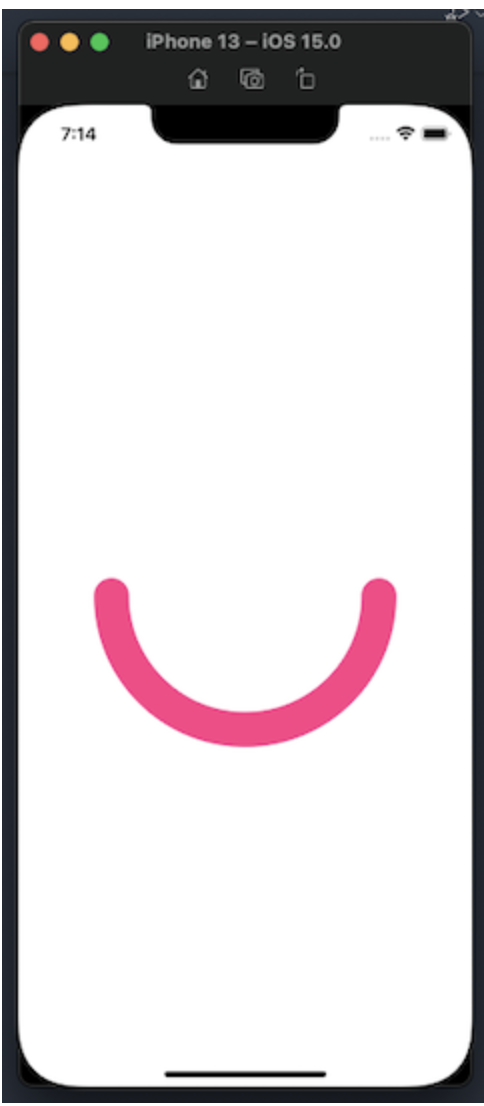
Is it not possible to offset the Circle Stroke on React-Native-Skia or is there an entirely different way to accomplish an effect like this that I am missing?
Issue Analytics
- State:
- Created a year ago
- Comments:5
Top Results From Across the Web
Shopify/react-native-skia@90db9fc - GitHub
High-performance React Native Graphics using Skia. Contribute to Shopify/react-native-skia development by creating an account on GitHub.
Read more >Ellipses | React Native Skia - Shopify
import { Canvas , Circle } from "@shopify/react-native-skia";. const CircleDemo = () => {. const r = 128;. return (. < Canvas style...
Read more >Build a Morphing Circle Animation with React Native Skia
A guide to building a Morphing Circle Animation in React - Native. A really cool and fun animation to add to your app...
Read more >GitHub is home to over 40 million developers ... - fasrcooking
facebook+reactjs+react-native-community+airbnb+react-community+reactide ... info+ku.reactjs.org+openr+chef-cookbooks+react-native-video+react-native-svg+ur.
Read more >D3 Charts and React Native Skia for Beginners | by Daniel Friyia
Learn to draw and Animate Beautiful Charts with React-Native-Skia. ... written about how to use React-Native and D3 with React-Native SVG and Re-Animated...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
you can use the following:
@Noitham you can use the
path.transform
method for that