[ANDROID] react-navgiation auth flow causes the source navigator to not be removed when destination navigator uses layout animations.
See original GitHub issueDescription
I’ve been using the layout animations but have noticed odd behavior when used in conjunction with react-navigation, specifically when replacing one navigator with another, for example: during an auth flow (https://reactnavigation.org/docs/auth-flow/).
export const NavigationStacks: React.FC = () => {
const context = useContext(MyContext);
if (context.isLoggedIn) {
return (
<TabsStack.Navigator>
<TabsStack.Screen name="HomeTab1" component={HomeScreen} />
<TabsStack.Screen name="HomeTab2" component={HomeScreen} />
</TabsStack.Navigator>
);
}
return (
<OnboardingStack.Navigator>
<OnboardingStack.Screen name="HomeScreen" component={LoggedOutScreen} />
</OnboardingStack.Navigator>
);
};
When launching the app from cold, if you replace a navigator with a screen that does not have an Animated.View with a layout animation, eg:
export const LoggedOutScreen: React.FC = () => {
const context = useContext(MyContext);
const navigation = useNavigation();
return (
<View style={styles.container}>
<Pressable
style={{backgroundColor: 'black', padding: 16, marginTop: 32}}
onPress={() => context.setIsLoggedIn(!context.isLoggedIn)}>
<Text style={{color: 'white', fontWeight: 'bold'}}>Login</Text>
</Pressable>
</View>
);
};
with a navigator whose first screen does have a layout animation, eg:
export const HomeScreen: React.FC = () => {
const context = useContext(MyContext);
return (
<View style={styles.container}>
<Animated.View entering={FadeIn}>
<Text>This is text with entering transition</Text>
</Animated.View>
<Button
onPress={() => context.setIsLoggedIn(!context.isLoggedIn)}
title="Logout"
/>
</View>
);
};
The first navigator stays in the android view hierarchy:
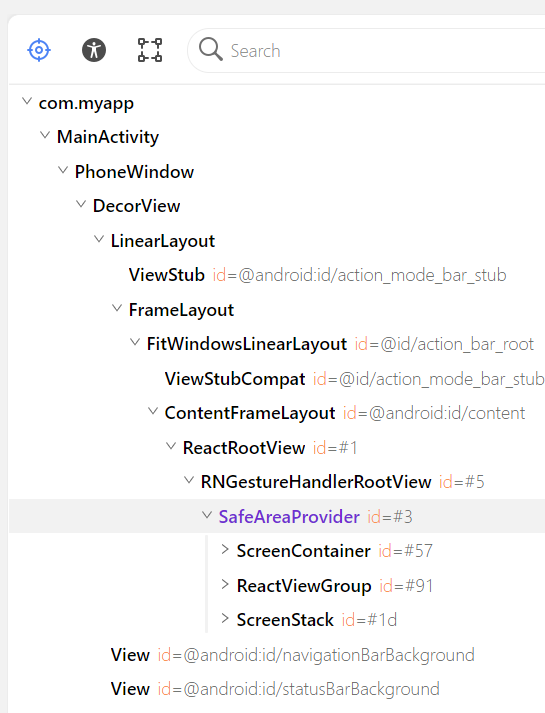
Things to note:
- If the source navigator also has an Animated.View with a layout animation, this does not happen.
- This only happens when launching cold. If I reload the app, it then works fine.
- The source navigator is rendered on top of the destination navigator, blocking any interaction.
- I don’t have a mac so can’t test this on iOS.
Expected behavior
When swapping out the navigators the layout animation should play and the previous navigator should be removed
Actual behavior & steps to reproduce
When swapping out the navigators, the source navigator remains in the android view hierarchy and blocks any interaction with the destination navigator.
Snack or minimal code example
I’ve created a project to demonstrate this: https://github.com/AcidNoX/navigation-reanimated-repro
Package versions
name | version |
---|---|
react-native | 0.66.4 |
react-native-reanimated | ^2.3.1 |
NodeJS | 12.22.6 |
Xcode | N/A |
Gradle | 4.2.2 |
expo | N/A |
@react-navigation/native | ^6.0.6 |
react-native-gesture-handler | ^2.1.1 |
react-native-screens | ^3.10.1 |
Affected platforms
- Android
- iOS
- Web
Issue Analytics
- State:
- Created 2 years ago
- Reactions:1
- Comments:7
Thank you for checking iOS @ankushss. Greatly appreciated.
Happening only on Android. iOS works well