[BUG] Generic pydantic model causes inconsistent OpenAPI spec generation
See original GitHub issueDescribe the bug I am wrapping my responses in a generic model like below (full code in toggle section).
class Response(GenericModel, Generic[DataT]):
"""Wrapper for responses"""
data: Optional[DataT]
I see two issues:
- The
/docs
endpoint renders things differently for a contained list or a contained single element. - PyCharm warns on type mismatch according to the annotations
I am wondering if I am using the GenericModel
in a wrong way or if there is a bug?
Click to toggle
from typing import TypeVar, Generic, Optional, List
import uvicorn
from fastapi import FastAPI
from pydantic import BaseModel
from pydantic.generics import GenericModel
DataT = TypeVar('DataT')
class Response(GenericModel, Generic[DataT]):
"""Wrapper for responses"""
data: Optional[DataT]
class ProjectOut(BaseModel):
"""Project model (out)"""
id: int
app = FastAPI()
out = ProjectOut(id=1)
@app.get(
'/projects/{item_id}',
response_model=Response[ProjectOut]
)
def get_single() -> Response[ProjectOut]:
return Response[ProjectOut](data=out)
@app.get(
'/projects',
response_model=Response[List[ProjectOut]])
def get_projects() -> Response[List[ProjectOut]]:
return Response[List[ProjectOut]](data=[out])
if __name__ == '__main__':
uvicorn.run(
f"{__name__}:app",
host='0.0.0.0',
port=8888,
reload=True,
debug=True,
log_level='info')
To Reproduce
Expected behavior
- The list response is shown as
Response[List[ProjectOut]]
- I see no warnings in PyCharm when I am using annotations correctly.
Screenshots
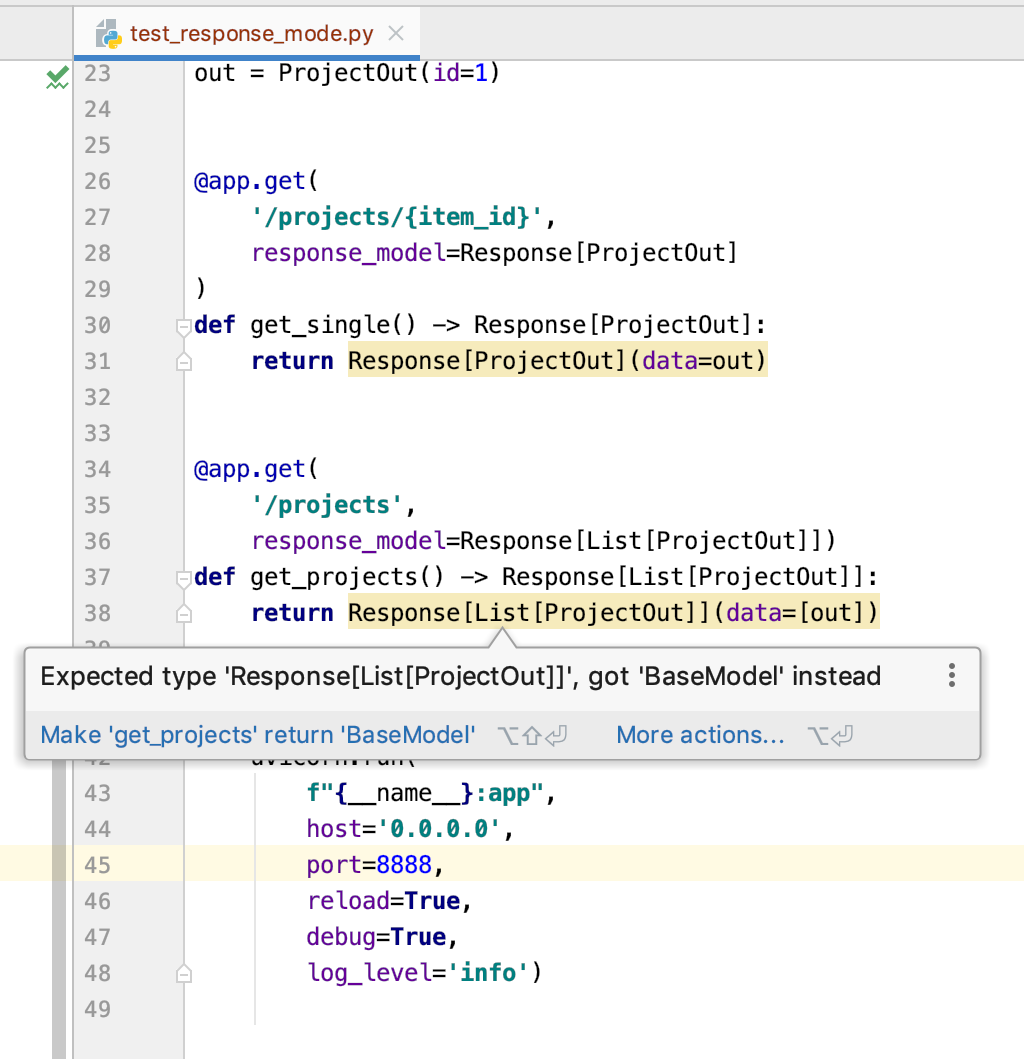
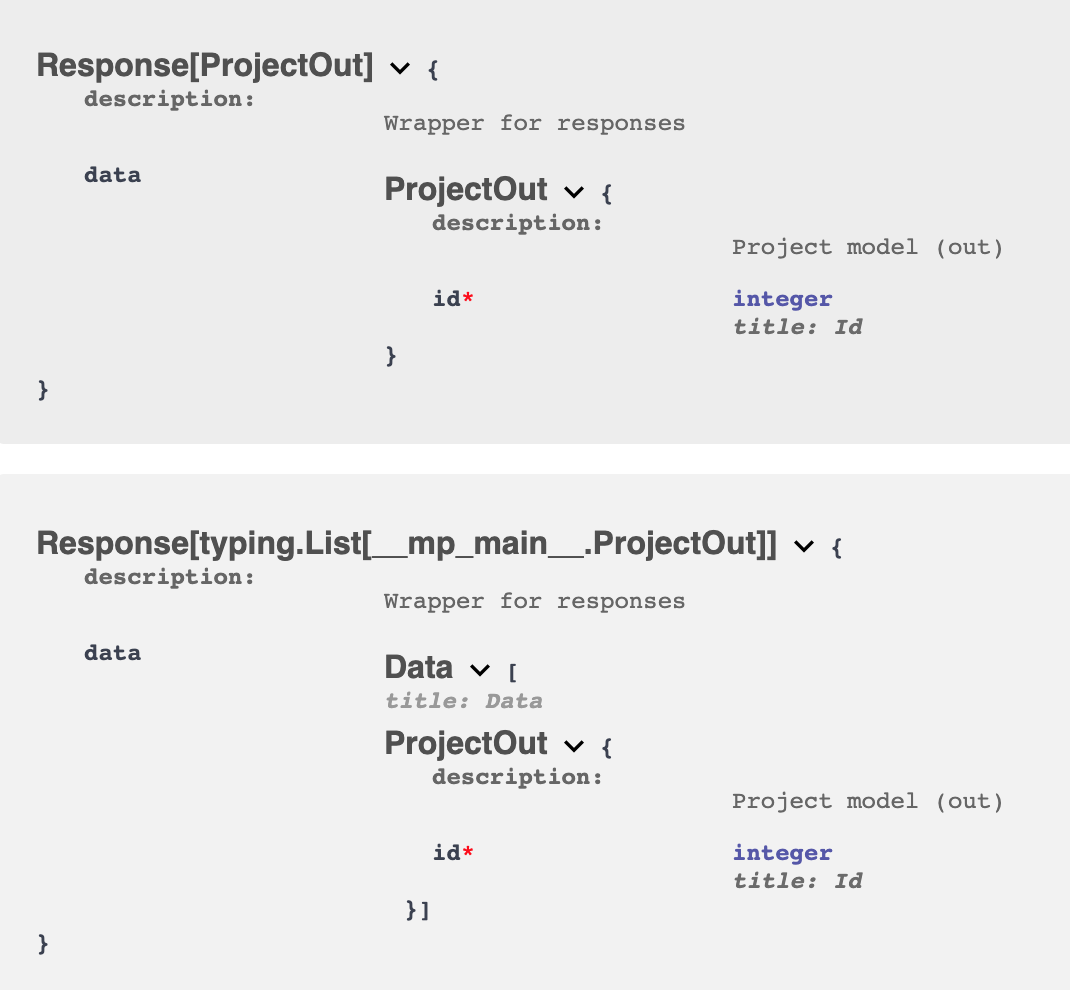
Environment:
- OS: macOS
- FastAPI Version: 0.42
- Python version: 3.7.4
Additional context Add any other context about the problem here.
Issue Analytics
- State:
- Created 4 years ago
- Comments:8 (4 by maintainers)
Top Results From Across the Web
Changelog - pydantic
support generic models with discriminated union, #3551 by @PrettyWood ... refactor schema generation to be compatible with JSON Schema and OpenAPI specs, ...
Read more >pydantic [python-library] - Occam :: Details
Fast and extensible, pydantic plays nicely with your linters/IDE/brain. Define how data should be in pure, canonical Python 3.6+; validate it with pydantic....
Read more >datamodel-code-generator - GitHub Pages
This code generator creates pydantic model from an openapi file and others.
Read more >pydantic - Bountysource
Bug. Output of python -c "import pydantic.utils; ... One of my model's fields is a Callable and I would like to call .json()...
Read more >pydantic — pydantic v0.28 documentation
use of recursive pydantic models, typing 's List and Dict etc. and ... pydantic would try to validate the default None which would...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
@KiraPC – I just stumbled upon the same issue as you and Google led me here, so I’ll write down the solution to that:
Instead of deriving your
PaginationModel
frompydantic.BaseModel
you have to usepydantic.generics.GenericModel
, so the following gives you the expected result:Hello everyone.
I have a similar issue to the one presented here.
Basically I’m using a pydantic BaseModel to represent the Pagination response. This class extends generic with a TypeVar.
Then I use as Generic input the object that should be in the results list
Then use it as route response model
When I print the openapi spec, I expect to have something like
but what actually I get is
I’m doing somethings wrong, or it is a bug?