Is there a way to skip redraw on opacity or other style related updates
See original GitHub issueCurrently, I am working on a project that ties a slider to the opacity of a tile layer. It looks like whenever the opacity value is updated, the entire overlay is redrawn. Performance feels sluggish when there are a few overlays on the map due to all the redraws. Is there a way to do this without going through the lifecycle methods and perhaps just update the inline styles instead? Please advise, thanks!
Sample code below:
const generateImageTiles = (data, opacity) => {
return new TileLayer({
opacity,
getTileData: ({ x, y, z }) =>
load(
http
.get(getTileUrl(data, x, y, z), {
responseType: 'arraybuffer',
withCredentials: true,
})
.then((response) => response.data),
ImageLoader
),
});
};
const Component = ({ opacity, data }) => {
const { map } = useContext(MapContext);
const [overlayRef, setOverlayRef] = useState();
useEffect(() => {
if (map) {
if (!overlayRef) {
const ref = new GoogleMapOverlay({
layers: [generateImageTiles(data, opacity)],
});
ref.setMap(map);
setOverlayRef(ref);
} else {
const layers = [generateImageTiles(data, opacity)];
overlayRef.setProps({ layers });
}
}
}, [data, opacity, overlayRef, map]);
return null;
};
I was able to around this somewhat by setting overlayRef._overlay.getPanes().overlayLayer.style.opacity
. However, this only gives control over the entire overlay but not the individual child layers.
Issue Analytics
- State:
- Created 3 years ago
- Comments:5
Top Results From Across the Web
Stylesheet not updating when I refresh my site - Stack Overflow
On Mac OS (in Chrome) use: Cmd + Shift + R . This will force your browser to reload and refresh all the...
Read more >How to create high-performance CSS animations - web.dev
Use the transform and opacity CSS properties as much as possible, and avoid anything that triggers layout or painting.
Read more >will-change - CSS: Cascading Style Sheets - MDN Web Docs
The will-change CSS property hints to browsers how an element is expected to change. Browsers may set up optimizations before an element is ......
Read more >Opacity Doesn't Live Update when using Properties Panel + ...
Click in the Opacity field and use the keyboard to increment the opacity up or down. Note that the object's opacity doesn't change...
Read more >Dropdown menu transparent after update - WordPress.org
Like you, since the yesterday update, the style of the menu is completely ... not mix with this one as it is another...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
I think I was hoping that there would be something like
overlayRef._overlay.getPanes().overlayLayer[0].style.opacity
where the index is the layer. But it goes against the concept of reactive programming and we are manipulating data outside of the lifecycles methods.I am going to close out the issue. Thanks for the taking out the time to answer the question.
Thanks for the link! Definitely appreciate all the work that’s being done with this library. That was very informative. Kudos to the team!
Our old implementation was using Google map APIs directly. Using the same slider and have it auto slide from first image to third image with no throttling, here are the results for each implementation.
Here is using our old implementation calling Google Map APIs: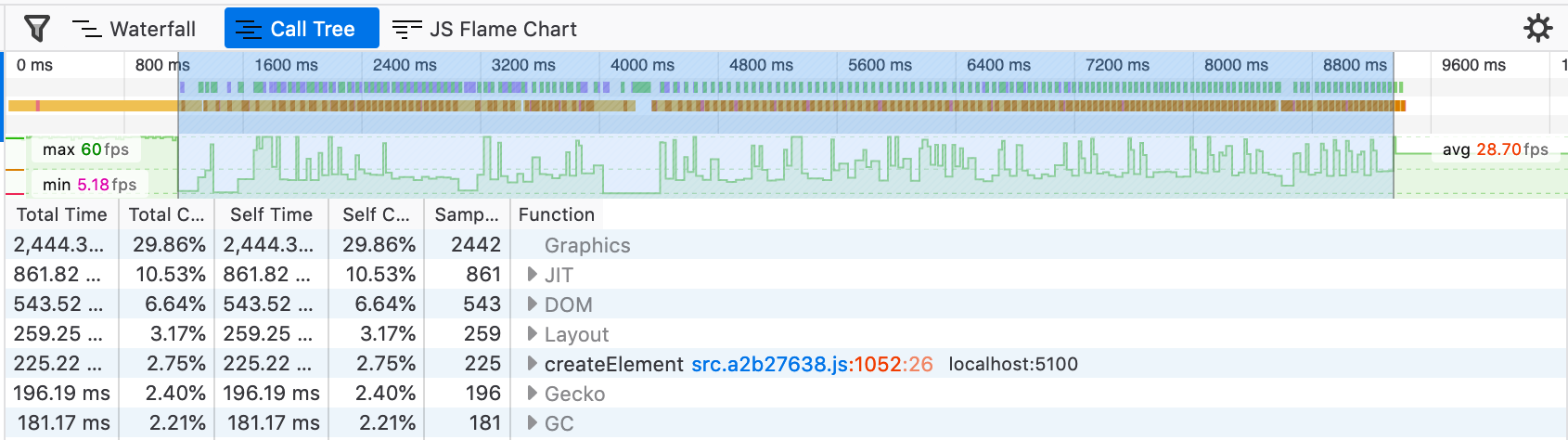
Here is the performance using deck.gl.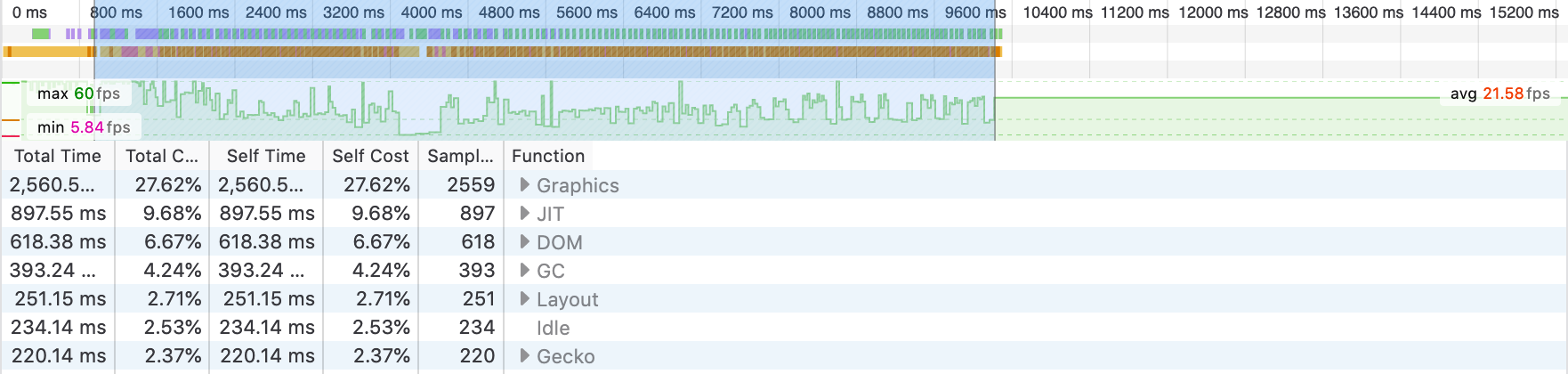
There are fewer frames per second with deck.gl and it’s also slightly slower. We have it working but was just curious if there’s an alternative.