Can't serialize poco class
See original GitHub issueI’m sening a POCO with a SignalR hub then, it gets sent to my subscribed client, but the data is lost.
The data I’m sending
public class WorkerOrderViewDto
{
public int Id { get; set; }
public string UserName { get; set; } = string.Empty;
public ICollection<SandwichDto> Sandwiches { get; set; } = new List<SandwichDto>();
public OrderState State { get; set; }
}
public enum OrderState
{
OrderPlaced,
InTheOven,
ToBeDelivered,
Delivered,
}
The Hub
public class WorkerViewOrderHub : Hub<IWorkerViewOrderClient>
{
}
public interface IWorkerViewOrderClient
{
Task ReceiveOrderAddedAsync(WorkerOrderViewDto orders);
}
Sending the data
private readonly IHubContext<WorkerViewOrderHub, IWorkerViewOrderClient> _workerViewHub;
public async Task<ActionResult> AddOrderAsync([FromBody] AddOrderDto addOrderDto)
{
var userId = _userManager.GetUserId(HttpContext.User) ?? throw new InvalidCommandException();
addOrderDto.UserId = userId;
addOrderDto.CustomerId = userId;
var result = await _serverOrderService.AddOrderAsync(addOrderDto);
var orderView = (await _serverOrderService.GetPendingOrdersForWorkerAsync()).SingleOrDefault(o => o.Id == result);
await _workerViewHub.Clients.All.ReceiveOrderAddedAsync(orderView);
return new OkResult();
}
SignalR Client
_connection.On<WorkerOrderViewDto>(HubNames.WorkerViewOrderHubNames.ReceiveOrderAddedAsync, OnOrdersModifiedAsync);
Task OnOrdersModifiedAsync(WorkerOrderViewDto order)
{
Orders.Add(order);
OrdersChanged?.Invoke();
return Task.CompletedTask;
}
Chrom debug inspection of the serialized data: https://imgur.com/gallery/m37ptwF
The client connects, gets the message, OnOrdersMidifiedAsync gets called, but the data is not serialized properly. Any idea what I’m doing wrong?
Issue Analytics
- State:
- Created 4 years ago
- Comments:5 (2 by maintainers)
Top Results From Across the Web
c# - Json.net cannot serialize if the class does not have an ...
If property's class has an empty constructer then this property is serialized but if there is only one constructer that you have to...
Read more >NonSerializedAttribute Class (System)
Indicates that a field of a serializable class should not be serialized. This class cannot be inherited.
Read more >XmlSerializer Class (System.Xml.Serialization)
Serializes and deserializes objects into and from XML documents. The XmlSerializer enables you to control how objects are encoded into XML.
Read more >SerializeReference Attribute? | Page 2
Is it really the case that a class can't serialize its own type reference? It's not a problem to add the extra interface...
Read more >Can't serialize nested class with JsonUtility
I'm trying to make a small save system using Json and would like to be able to serialize a custom serializable class.
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
@KuraiAndras I didn’t really like the idea of adding the
JsonPropertyName
attribute to each model used in communication with SignalR 😉 so I tried to solve the problem on the server side where “camelCase” serialization occurs:Models in messages incomming to client side are then deserialized without problems (WARN: for outgoing models camelCase is still applied but this is not problem - model are properly deserialized on server side).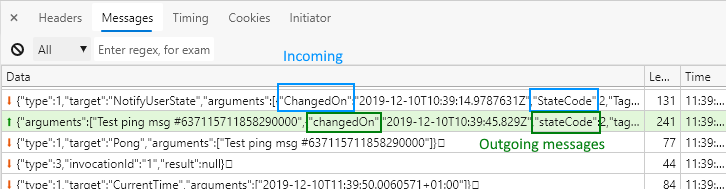
I have discovered that
JsonPropertyName
attribute is still required when using MessagePack otherwise we will encounter following exception on client side:If that is an API that is available on the original SignalR client and/or the JavaScript one yeah, we can have that.
The intent of this project is to wrap the JavaScript client and provide as much as possible the .Net API compatibility.