Creating a CubeTexture from 6 CompressedTextures doesn't work after r136
See original GitHub issueDescribe the bug
Prior to version r136
of the library, creating a CubeTexture
from 6 CompressedTextures
used to work just fine. However, it seems like this change introduced in r136 changed the behaviour of how textures are loaded into the GPU when WebGL 2 APIs are available, which broke this use-case.
As a work-around, I have been able to get the cube texture to render by setting texture.isVideoTexture = true
on the CubeTexture
. According to this line, that forces Three to opt-out of using texStorage2D
which seems to be causing the issue
Code
const loader = new KTX2Loader()
.setTranscoderPath( 'js/libs/basis/' )
.detectSupport( renderer );
const textures = await Promise.all([
loader.loadAsync( './textures/nx.ktx2' ),
loader.loadAsync( './textures/px.ktx2' ),
loader.loadAsync( './textures/ny.ktx2' ),
loader.loadAsync( './textures/py.ktx2' ),
loader.loadAsync( './textures/nz.ktx2' ),
loader.loadAsync( './textures/pz.ktx2' )
]);
textures.forEach((texture) => {
texture.generateMipmaps = false;
texture.encoding = THREE.LinearEncoding;
texture.needsUpdate = true;
});
const cubeTexture = new THREE.CubeTexture(textures);
cubeTexture.encoding = textures[0].encoding;
cubeTexture.format = textures[0].format;
cubeTexture.minFilter = textures[0].minFilter;
cubeTexture.magFilter = textures[0].magFilter;
cubeTexture.generateMipmaps = false;
// HACK: Setting `isVideoTexture` opts-out of using `texStorage2D`
cubeTexture.isVideoTexture = true;
cubeTexture.needsUpdate = true;
// Set scene background
scene.background = cubeTexture
Live example
- CodeSandbox (I’m using react-three-fiber, let me know if you want a vanilla Three.js version!)
Expected behavior
It should load the CubeTexture
just fine as the scene background. Instead, the scene background is black and the following errors show in the console:
[.WebGL-0x700abe0a00] GL_INVALID_VALUE: Texture dimensions must all be greater than zero.
and
[.WebGL-0x700abe0a00] GL_INVALID_OPERATION: Level of detail outside of range.
Upgrading to r140.2, also shows this added error:
THREE.WebGLTextures: sRGB encoded textures have to use RGBAFormat and UnsignedByteType.
Screenshots
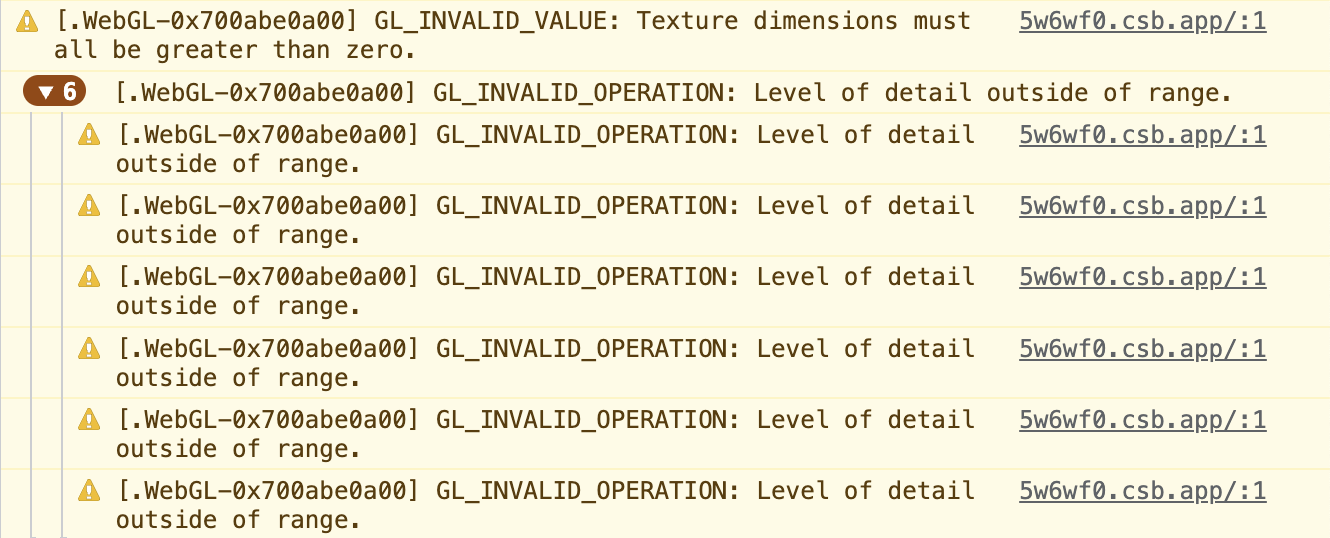
https://user-images.githubusercontent.com/8302959/168479550-cf0d681d-cd96-40a6-a816-18699047322b.mov
Platform:
- Device: Desktop
- OS: MacOS
- Browser: Chrome
- Three.js version: r136
Issue Analytics
- State:
- Created a year ago
- Comments:9 (2 by maintainers)
Your platform probably does not fully support S3TC.
This warning is generate in
WebGLTextures.verifyColorSpace()
. However, there is an early out for all compressed textures. So whenRGBA_ASTC_4x4_Format
is used in combination withsRGBEncoding
, there is no warning and the renderer should pickCOMPRESSED_SRGB8_ALPHA8_ASTC_4x4_KHR
.I think the main problem is that compressed cube textures also have to use
CompressedTexture
and notCubeTexture
. So usingnew THREE.CubeTexture(textures);
whiletextures
is an array of compressed textures does not work. It’s required to organize the compress cube map similar to howDDSLoader
works.