Drawer hideStatusBar breaks SafeAreaView on repeated navigation with screens enabled
See original GitHub issueDescription
When you have a Drawer navigation with hideStatusBar
prop and screens enabled, repeated navigation to the same screen is setting SaveAreaView top inset to 0.
Screenshots
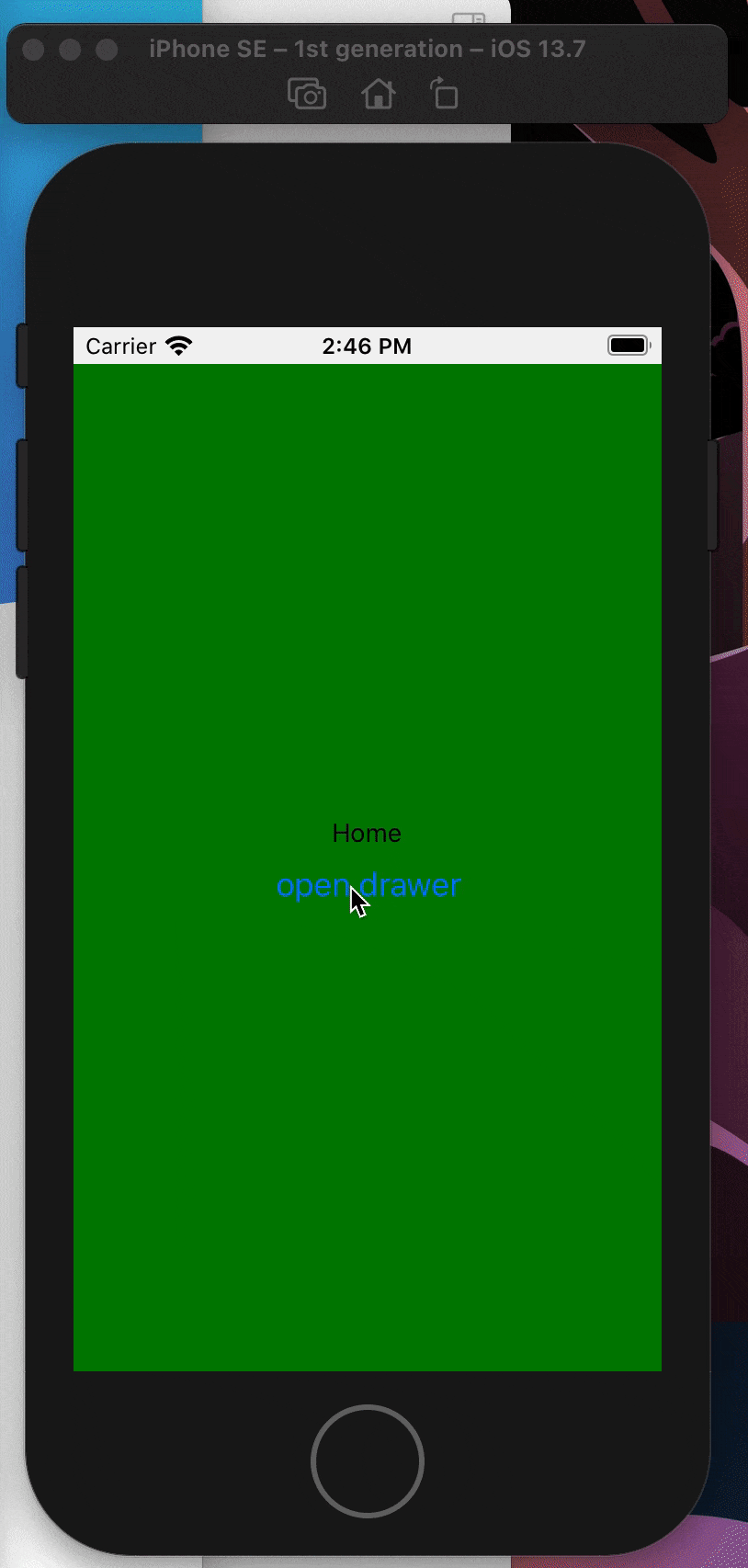
Steps To Reproduce
- open app, see that the green background does not cover status bar
- go to Notifications, status bar is not covered
- open drawer again and dismiss it or go to notifications again
- status bar is covered with green
Expected behavior
- status bar should not be covered
Snack or minimal code example
import 'react-native-gesture-handler';
import {createDrawerNavigator} from '@react-navigation/drawer';
import {NavigationContainer} from '@react-navigation/native';
import {View, Text, Button} from 'react-native';
import React, {StrictMode} from 'react';
import {SafeAreaProvider, SafeAreaView} from 'react-native-safe-area-context';
import {enableScreens} from 'react-native-screens';
enableScreens();
const Drawer = createDrawerNavigator();
function Home({navigation}) {
return (
<SafeAreaView style={{flex: 1}}>
<View
style={{
backgroundColor: 'green',
flex: 1,
alignItems: 'center',
justifyContent: 'center',
}}>
<Text>Home</Text>
<Button
onPress={() => {
navigation.openDrawer();
}}
title="open drawer"
/>
</View>
</SafeAreaView>
);
}
function Notifications({navigation}) {
return (
<SafeAreaView style={{flex: 1}}>
<View
style={{
backgroundColor: 'green',
flex: 1,
alignItems: 'center',
justifyContent: 'center',
}}>
<Text>Notifications</Text>
<Button
onPress={() => {
navigation.openDrawer();
}}
title="open drawer"
/>
</View>
</SafeAreaView>
);
}
function App() {
return (
<StrictMode>
<SafeAreaProvider>
<NavigationContainer>
<Drawer.Navigator initialRouteName="Home" hideStatusBar>
<Drawer.Screen name="Home" component={Home} />
<Drawer.Screen name="Notifications" component={Notifications} />
</Drawer.Navigator>
</NavigationContainer>
</SafeAreaProvider>
</StrictMode>
);
}
export default App;
Package versions
"@react-navigation/drawer": "^5.12.3",
"@react-navigation/native": "^5.9.2",
"react": "16.13.1",
"react-native": "0.63.4",
"react-native-gesture-handler": "^1.10.1",
"react-native-reanimated": "^1.13.2",
"react-native-safe-area-context": "^3.1.9",
"react-native-screens": "^2.17.1"
Issue Analytics
- State:
- Created 3 years ago
- Comments:7 (3 by maintainers)
Top Results From Across the Web
Different status bar configuration based on route
Different status bar configuration based on route. If you don't have a navigation header, or your navigation header changes color based on the...
Read more >how to hide a screen from drawer in react navigation 5
1. function DrawerNavigator() { ; 2. return ( ; 3. <NavigationContainer> ; 4. <Drawer.Navigator ; 5. initialRouteName="Home".
Read more >How to use SafeAreaView for Android notch devices?
Do something like import { StyleSheet, Platform, StatusBar } from "react-native"; export default StyleSheet.create({ AndroidSafeArea: ...
Read more >How to Handle Safe Area Insets for iPhone X, iPad X, Android P
Devices with Edge-to-Edge Screens and a Notch or Display Cutout ... adds tab navigation (iOS) or drawer menu (Android) Navigation {
Read more >Combining Drawer, Tab and Stack navigators in React ...
We will also implement each of the routes as a Stack Navigator, since we now that, for instance, the Book flow will contain...
Read more >
Top Related Medium Post
No results found
Top Related StackOverflow Question
No results found
Troubleshoot Live Code
Lightrun enables developers to add logs, metrics and snapshots to live code - no restarts or redeploys required.
Start Free
Top Related Reddit Thread
No results found
Top Related Hackernoon Post
No results found
Top Related Tweet
No results found
Top Related Dev.to Post
No results found
Top Related Hashnode Post
No results found
Thanks a lot! Closing it then as soon as this is expected behavior
I am not too keen with the implementation of the library, but it may be related to usage of
ViewControllers
for each of the screens in the navigator, when usingreact-native-screens
. Each screen perhaps requires theSafeAreaProvider
to be under it. Maybe @janicduplessis could elaborate more on this. Can I help you any more regarding this issue or can we close it?